Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial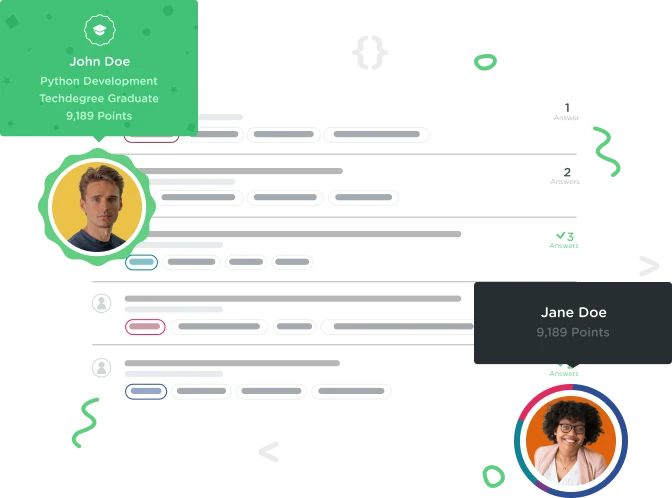
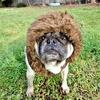
Kathryn Kassapides
9,260 PointsSolved this different from teacher
In the Branch and Loop project in Python, I solved it using while and if but the teacher used an if and else. It worked but is it in Python?
ticket_price = 10
tickets_remaining = 100
print("There are {} left to buy".format(tickets_remaining))
name = input("name: ")
ticket_number = input("{}, how amny tickets do u want? ".format(name))
ticket_number = int(ticket_number)
price = ticket_price * ticket_number
print("{}, you owe ${} for {} ticket".format(name, price, ticket_number))
continue_it = input("Do u wish 2 continue? y/n")
while continue_it == 'y':
print("SOLD!")
total_tickets = tickets_remaining - ticket_number
if continue_it == 'n':
print("{}, thanks".format(name))
3 Answers
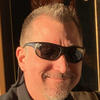
Peter Vann
36,429 PointsHi Kathryn!
That you are trying to come up with solutions that deviate from the challenges is a good sign.
It shows you are gaining confidence with the material and getting creative, which is awesome and is clearly anyone's endgame in a discipline like programming.
And while loops are a useful way to prompt for user input (and keep re-prompting until they give a valid input!?! LOL)
And yes, you are thinking like a true Pythonista! :)
This is my solution, which goes well beyond the challenge, too:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
valid = False
while valid != True:
client_name = input("What is your name? ")
if (len(client_name) == 0):
print("*** INVALID SELECTION ***")
else:
try:
val = int(client_name) ### IF THIS FAILS, client_name is a string - the desired value type ###
except ValueError: ### BACKWORDS LOGIC HERE ###
valid = True
else:
print("*** INVALID SELECTION ***")
valid = False
while valid != True:
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
try:
val = int(num_ticket)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val <= tickets_remaining:
valid = True
else:
print("*** ERROR ***: There {} only {} ticket{} remaining. Try again.".format(verb, tickets_remaining, remaining_s))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
If you notice, in one section I sort of use ValueError backwards, but it makes it work. - I try to cast clientName to an int, and when it errors, I know it's a string (and therefore valid when it errors!?!) Again, kind of backwards logic, but sometimes you have to think outside-the-box!?! LOL
I hope that helps.
Stay safe and happy coding!
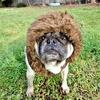
Kathryn Kassapides
9,260 PointsThank you! This helps me very much! I'll definitely be referring to this while I learn :)
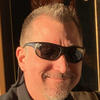
Peter Vann
36,429 PointsHi Kathryn!
Cool - I'm glad that helps.
One recommendation, when doing the challenges, if you want an easy way to test small samples of code, I go here:
https://www.katacoda.com/courses/python/playground
(And I tend to run python3 app.py, not python app.py)
Also, you will probably eventually encounter (in Object-Oriented Python) a "concentration"-like game project coding challenge.
Again, I tried to code it before following the instructor's approach, so my approach differs greatly from the instructor's, and I like my version better:
This is it (if I remember correctly, it runs with Python3):
import random
import os
import sys
# functions
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def int2ascii(int):
return chr(int + 64)
def exitGame():
clear()
print('Bye!')
sys.exit()
class Cell():
def __init__(self, id, value):
self.id = id
self.value = value
self.matched = False
def __eq__(self, other):
return self.value == other.value
def __str__(self):
return self.value
class Grid():
__title = 'Concentration (Memory Game)'
__heading = ' | A | B | C | D |'
__cells = list()
__locations = list()
__values = list()
__matchCount = 0
__matchedLocations = list()
def __init__(self):
items = ['Egg',
'Hut',
'Bat',
'Cat',
'Ant',
'Dog',
'Pie',
'Eye']
list1 = items
list2 = items
random.shuffle(list1)
random.shuffle(list2)
values = list1 + list2
random.shuffle(values)
k = 0
for i in range(0, 4):
for j in range(0, 4):
id = int2ascii(j+1) + str(i+1)
#print('Row ' + str(i+1))
#print('Column ' + int2ascii(j+1))
self.__cells.append(Cell(id, values[k]))
self.__locations.append(id)
self.__values.append(values[k])
k += 1
def getCells(self):
return self.__cells
def getLocations(self):
return self.__locations
def getValues(self):
return self.__values
def setMatched(self, firstIndex, secondIndex):
self.__cells[firstIndex].matched = True
self.__matchedLocations.append(self.__cells[firstIndex].id)
self.__cells[secondIndex].matched = True
self.__matchedLocations.append(self.__cells[secondIndex].id)
self.__matchCount += 1
def getMatchCount(self):
return self.__matchCount
def getMatchedLocations(self):
return self.__matchedLocations
# def randomPrint(self):
# cells = self.__cells
# for x in range(0, 16):
# print(cells[x].id + ' ' + cells[x].value + ' ' + str(cells[x].matched))
# def drawBlankRow(self, i):
# print(str(i+1) + '| | | | |')
def draw(self, clearable):
if (clearable):
clear()
cells = self.__cells
print(self.__title)
print(self.__heading)
z = 0
for x in range(1, 17, 4):
z += 1
#new row
row = str(z) + '|'
for y in range(0, 4):
# print(str(z) + ' ' + str(y)+ ' ' + str(x+y-1))
k = (x+y-1)
if (cells[k].matched == True):
row += ' ' + cells[k].value + ' |'
else:
row += ' |'
print(row)
#grid.randomPrint()
# grid.draw()
#print(grid.getLocations())
def pick_cards(gridObj):
valid = False
possible_values = gridObj.getLocations()
alreadyMatched = gridObj.getMatchedLocations()
cells = gridObj.getCells()
# items = gridObj.getValues() # NOT NEEDED
# print(possible_values)
# print(items)
while valid != True:
firstCard = input("What's the location of your first card?: ")
firstCard = firstCard.upper()
if (firstCard == 'Q'):
exitGame()
if firstCard in possible_values:
if firstCard not in alreadyMatched:
valid = True
else:
print("*** THIS CARD (" + firstCard + ") HAS ALREADY BEEN MATCHED ***")
else:
print("*** INVALID SELECTION ***")
valid = False
while valid != True:
secondCard = input("What's the location of your second card?: ")
secondCard = secondCard.upper()
if (secondCard == 'Q'):
exitGame()
if secondCard in possible_values:
if secondCard not in alreadyMatched:
valid = True
else:
print("*** THIS CARD (" + secondCard + ") HAS ALREADY BEEN MATCHED ***")
else:
print("*** INVALID SELECTION ***")
for index, x in enumerate(cells):
if x.id == firstCard:
firstValue = x.value
firstIndex = index
break
else:
x = None
for index, y in enumerate(cells):
if y.id == secondCard:
secondValue = y.value
secondIndex = index
break
else:
y = None
print(firstCard + ': ' + firstValue)
print(secondCard + ': ' + secondValue)
if (firstValue != secondValue):
input('Those cards are not a match. Press enter/return to continue...')
else:
gridObj.setMatched(firstIndex, secondIndex)
input('Those cards match! Press enter/return to continue...')
matchCount = gridObj.getMatchCount()
# input(matchCount)
if(matchCount == 8):
clear()
print('Congrats!! You have matched them all!')
print('')
return False
else:
return True
# Play the game
grid = Grid()
tryAgain = True
grid.draw(tryAgain)
while tryAgain:
tryAgain = pick_cards(grid)
grid.draw(tryAgain)
print('')
print('*** GAME OVER ***')
print('')
print('')
(Note: you'll see some places where I comment-out certain lines of code that I would periodically uncomment for testing/debugging purposes!?!)
Also, on my own (outside of Treehouse), I coded a game that basically allows you to explore the "Monty Hall Problem":
https://www.youtube.com/watch?v=iBdjqtR2iK4
I set it up so you can play it manually, one game at a time, or you can run it "automatically" and then you can run it 100, 1000, 10,000, 100,000 times in row, to really see how it is obviously better to switch every time (odds of winning being twice as likely if you switch than if you stay.)
This also runs with python3:
import random
import os
import sys
# get list of words from a file
#file = open('words.txt', 'r')
#words = [x.strip() for x in file]
class door:
__hasCar = False
__isPicked = False
def set_hasCar(self, hasCar):
self.__hasCar = hasCar
def get_hasCar(self):
return self.__hasCar
def set_isPicked(self, isPicked):
self.__isPicked = isPicked
def get_isPicked(self):
return self.__isPicked
door1 = door()
door2 = door()
door3 = door()
x = 20
y = 10
scores = {
'stayed': 0,
'stayed_games_won': 0,
'switched': 0,
'switched_games_won': 0
}
#scores = [0,0,0,0]
doors = [None, door1, door2, door3]
'''
door1.set_hasCar(True)
if doors[1].get_hasCar() is None:
print("null")
else:
print(door1.get_hasCar())
'''
# functions
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def move_xy(x, y):
for i in range(y):
print("")
for i in range(x):
# sys.stdout.write(' ') Python 2
print(" ", end="") # python3
def moving_on():
nada = input("'Enter/Return' to continue...")
def is_odd(i):
if i % 2 == 0: #EVEN
return False
else:
return True
def get_auto_number():
number = 0
move_xy(30, y)
decision = input("Play manually? [Y/n]: ").lower()
if decision == 'n':
valid = False
while valid != True:
move_xy(27, 2)
number = input("How many automatic games?: ")
try:
val = int(number)
except ValueError:
print("*** INVALID SELECTION ***")
continue
valid = True
move_xy(15, 2)
print(str(val) + " of each game (stay/switch) will play automatically!")
move_xy(27, 2)
moving_on()
if val == 0:
move_xy(30, 1)
print("(" + str(val) + " automatic games = manual play!?!)")
return val # = user input
else:
return number # = 0 (default)
def mode(auto_number):
#print(auto_number == 0)
#print("Auto_Number = " + str(auto_number))
if auto_number != 0:
#print(str(auto_number) + " is NOT equal to ZERO!")
#print("Will return True")
return True
else:
#print(str(auto_number) + " is equal to ZERO!")
#print("Will return False")
return False
def put_car_behind_door():
# unpick doors/cars (for repeat play)
for i in range(1, 4):
doors[i].set_isPicked(False)
doors[i].set_hasCar(False)
car_door_number = random.randint(1, 3)
doors[car_door_number].set_hasCar(True)
return car_door_number
def pick_door():
valid = False
possible_values = [1, 2, 3]
while valid != True:
picked_door_number = input("Pick a door... (1, 2, or 3): ")
try:
val = int(picked_door_number)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val in possible_values:
valid = True
else:
print("*** INVALID SELECTION ***")
doors[val].set_isPicked(True)
return val
def auto_pick_door():
return random.randint(1, 3)
def monty_reveals_goat(doors):
goat_doors = [1, 2, 3]
for i in range(1, 4):
if doors[i].get_isPicked() == True:
print(goat_doors)
print(str(i-1))
goat_doors.pop(i-1)
goat_door_index = random.randint(0, 1)
goat_door_number = goat_doors[goat_door_index]
clear()
move_xy(x, 8)
print("Monty Hall:")
move_xy(x, 2)
print("I've opened door #" + str(goat_door_number) + " to reveal a goat.")
move_xy(x, 4)
return goat_door_number
def auto_monty(doors):
goat_doors = [1, 2, 3]
for i in range(1, 4):
if doors[i].get_isPicked() == True:
#print(goat_doors)
#print(str(i-1))
goat_doors.pop(i-1)
goat_door_index = random.randint(0, 1)
goat_door_number = goat_doors[goat_door_index]
return goat_door_number
def stay_or_switch():
decision = input("Do you want to stay (or switch)? [Y for 'Stay' / n for 'Switch']: ").lower()
if decision != 'n':
return True
else:
return False
def you_have_decided(decision):
if decision == True:
print('You have decided to stay!')
else:
print('You have decided to switch!')
def you_have_picked(picked_door_number):
print("You've picked door #" + str(picked_door_number) + "!")
def outcome(stay, picked_door_number, goat_door_number):
#print('Has car: ' + str(doors[picked_door_number].get_hasCar()))
clear()
move_xy(35, y)
if stay == True:
scores['stayed'] += 1
if doors[picked_door_number].get_hasCar() == True:
print("You won!")
scores['stayed_games_won'] += 1
else:
print("You lost!")
else: #switch
scores['switched'] += 1
if doors[picked_door_number].get_hasCar() == True:
print("You lost!")
else:
print("You won!")
scores['switched_games_won'] += 1
move_xy(25, 4)
def auto_outcome(stay, picked_door_number, goat_door_number):
if stay == True:
scores['stayed'] += 1
if doors[picked_door_number].get_hasCar() == True:
scores['stayed_games_won'] += 1
else: #switch
scores['switched'] += 1
if doors[picked_door_number].get_hasCar() != True:
scores['switched_games_won'] += 1
def draw_game(picked_door_number, goat_door_number):
clear()
door_states = ['?', '?', '?']
for i in range(1, 4):
#print(door_states)
if i == picked_door_number:
door_states[i-1] = 'P'
elif i == goat_door_number:
door_states[i-1] = 'G'
print('Stayed: {}'.format(scores['stayed']))
print('Stayed games won: {}'.format(scores['stayed_games_won']))
print("================")
print('Switched: {}'.format(scores['switched']))
print('Switched games won: {}'.format(scores['switched_games_won']))
print("==================")
#clear()
move_xy(25, 3)
print("Doors:")
move_xy(30, 2)
print(" " + door_states[0] + " " + door_states[1] + " " + door_states[2])
move_xy(10, 5)
def draw_game_last(picked_door_number, goat_door_number):
clear()
door_states = ['?', '?', '?']
for i in range(1, 4):
#print(door_states)
if i == picked_door_number:
door_states[i-1] = 'P'
elif i == goat_door_number:
door_states[i-1] = 'G'
for i in range(1, 4):
#print(door_states)
if doors[picked_door_number].get_hasCar() == True:
door_states[picked_door_number-1] = 'C'
elif i == goat_door_number:
door_states[i-1] = 'G'
if not 'C' in door_states:
for i in range(1, 4):
#print(door_states)
if door_states[i-1] == '?':
door_states[i-1] = 'C'
print('Stayed: {}'.format(scores['stayed']))
print('Stayed games won: {}'.format(scores['stayed_games_won']))
print("================")
print('Switched: {}'.format(scores['switched']))
print('Switched games won: {}'.format(scores['switched_games_won']))
print("==================")
#clear()
move_xy(25, 3)
print("Doors:")
move_xy(30, 2)
print(" " + door_states[0] + " " + door_states[1] + " " + door_states[2])
move_xy(10, 5)
def play(done):
picked_door_number = '?'
goat_door_number = '?'
auto = False
car_door_number = put_car_behind_door()
clear()
#move_xy(x, y)
autonumber = get_auto_number()
auto = mode(autonumber)
autonumber = (autonumber * 2) + 1
if not auto: #MANUAL
autonumber = 2 #DEFAULT
for i in range(1, autonumber):
if not auto:
draw_game(picked_door_number, goat_door_number)
moving_on()
#clear()
if not auto:
draw_game(picked_door_number, goat_door_number)
picked_door_number = pick_door()
draw_game(picked_door_number, goat_door_number)
you_have_picked(picked_door_number)
move_xy(x, 2)
moving_on()
else:
picked_door_number = auto_pick_door()
if not auto:
goat_door_number = monty_reveals_goat(doors)
moving_on()
clear()
else:
goat_door_number = auto_monty(doors)
if not auto:
draw_game(picked_door_number, goat_door_number)
decision = stay_or_switch()
draw_game(picked_door_number, goat_door_number)
you_have_decided(decision)
move_xy(x, 2)
moving_on()
if not auto:
outcome(decision, picked_door_number, goat_door_number)
moving_on()
else:
auto_outcome(is_odd(i), picked_door_number, goat_door_number)
draw_game_last(picked_door_number, goat_door_number)
move_xy(x, 2)
moving_on()
done = True
if done:
clear()
move_xy(30, y)
play_again = input("Play again? Y/n ").lower()
if play_again != 'n':
return play(done=False)
else:
clear()
move_xy(40, 10)
print("Bye!")
print("\n\n\n")
sys.exit()
def intro():
start = input("Press enter/return to start or 'q' to quit.")
if start == 'q':
clear()
move_xy(10, 30)
print("Bye!")
move_xy(10, 0)
sys.exit()
else:
return True
def welcome():
clear()
move_xy(x, y)
print("Welcome to the 'Monty Hall Problem' Game!")
# main
welcome()
'''
mydict = {'a':1,'b':'2'}
def test(**mydict):
print('MyDic[A]: ' + str(mydict['a']))
test()
mydict = [1,2,3,4]
def test():
print('MyDic[A]: ' + str(mydict[0]))
test()
'''
done = False
while True:
# clear()
move_xy(20, 2)
intro()
play(done)
Again, I hope that helps.
Stay safe and happy coding!