Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial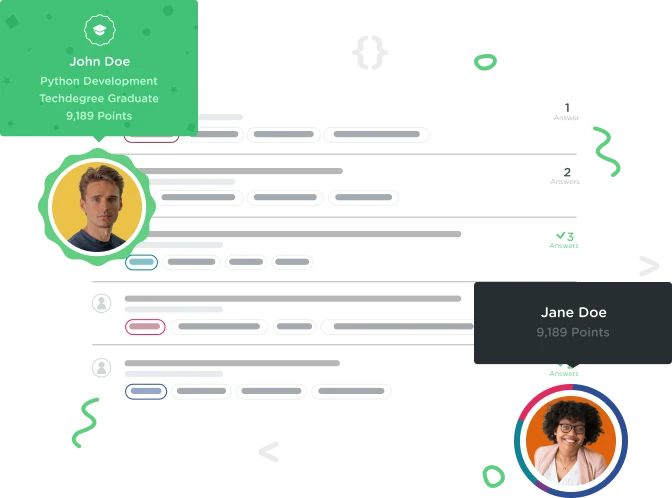
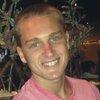
Chandler Tate
3,963 Points[SOLVED] -- Xcode returning wrong ticket prices, can't figure it out :(
The program is supposed to return the employee matinee price of $0 and print the message "Thanks for being apart of the team. Enjoy your FREE movie!" However, it keeps returning the price of the age and matinee price of $6.5 and I can't figure out why. I see the bool to true for both is employee and is matinee. If anyone can figure out what's going wrong it would be a huge help! Thanks! :)
//
// main.m
// boxOfficePlus
//
// Created by Chandler Tate on 4/1/16.
// Copyright © 2016 Chandler Tate. All rights reserved.
//
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[]) {
//AND &&
//OR ||
//NOT !
//Is the customer either under 13 years old, or 65 or over, if so, they get an "ageDiscount"
//Is the movie a matinee, as in, the first showing of the day?
//Is the customer also a theare employee?
bool ageDiscount;
bool isMatinee = TRUE;
bool isEmployee = TRUE;
//Full price tickets = $10
//For a non-employee with an age discount AND attending a matinee = $6.50
//For a non-employee with an age discount or attending a matinee = $8
//For an employee attending a non-matinee = $4.50
//For an employee attending a matinee = $0.0 (FREE)
float regularPrice = 10;
float ageOrMatineePrice = 8.5;
float ageAndMatineePrice = 6.5;
float employeeRegPrice = 4.5;
float employeeMatineePrice = 0;
int customerAge;
float customerPrice;
int youthAge = 13;
int seniorAge = 65;
NSString *empMessage;
/*
if ((customerAge < youthAge) || (customerAge >= seniorAge)) {
ageDiscount = YES;
}
*/
ageDiscount =((customerAge < youthAge) || (customerAge >= seniorAge)) ? YES:NO;
if (ageDiscount && isMatinee && isEmployee) {
customerPrice = ageAndMatineePrice;
}
else if ((ageDiscount || isMatinee) && !isEmployee){
customerPrice = ageOrMatineePrice;
}
else if (isEmployee && !isMatinee){
customerPrice = employeeRegPrice;
NSString *empMessage = @"Thanks for being apart of the team. Enjoy your movie!";
NSLog(@"%@", empMessage);
}
else if (isEmployee && isMatinee){
customerPrice = employeeMatineePrice;
empMessage = @"Thanks for being apart of team. Enjoy your FREE movie!";
NSLog(@"%@", empMessage);
}
else{
customerPrice = regularPrice;
}
return 0;
}
Chandler Tate
3,963 PointsChandler Tate
3,963 PointsHmm, I seem to have figured out what the issue was. I had to set the customer age to above 13, but less than 65. However, in the video for this lesson, the age value of the customer is never set and the program runs perfectly without any values assigned to the age. Is there something I maybe did wrong in regards to this?
Thanks again! :)