Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial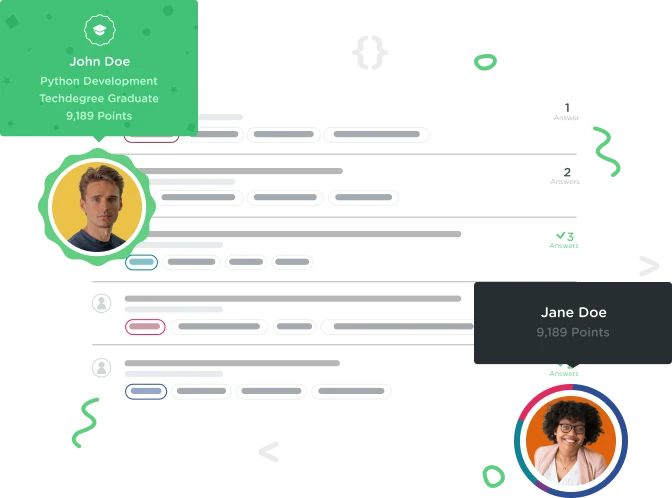

Aaron Coursolle
18,014 Points[Solved]Validation challenge?
I don't know what to put in my "if" condition to make the code work.
The comments that were included in the challenge itself are more or less the instructions for the challenge...you have to throw an IllegalArgumentException if the conditions do not pass.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' && (fieldName.charAt(1) > "A" && fieldName.charAt(1) < "Z"))
{
throw new IllegalArgumentException("The " + fieldName + " style does not match.");
}
return fieldName;
}
}
2 Answers

Philip G
14,600 PointsHi Aaron,
In your case it's best to use Character.isUpperCase()
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (!(fieldName.charAt(0) == 'm' && Character.isUpperCase(fieldName.charAt(1))))
{
throw new IllegalArgumentException("The " + fieldName + " style does not match.");
}
return fieldName;
}
}
Best Regards,
Philip

Aaron Coursolle
18,014 PointsOkay. I searched the forum, but everyone has their own way of coming up with the same answer. Here is how I got my way to work (mainly by changing quotes from double to single and changing the && to ||):
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' || (fieldName.charAt(1) < 'A' || fieldName.charAt(1) > 'Z'))
{
throw new IllegalArgumentException("The " + fieldName + " style does not match.");
}
return fieldName;
}
}
Aaron Coursolle
18,014 PointsAaron Coursolle
18,014 PointsHi Philip,
Won't the m_somethingPluggedInHere still pass the code you posted above?
Philip G
14,600 PointsPhilip G
14,600 PointsI don't think so https://ideone.com/xrKgpH
Aaron Coursolle
18,014 PointsAaron Coursolle
18,014 PointsI do like the elegance of the not operator that you used at the start of the condition statement, instead of making everything "not" one condition at a time