Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial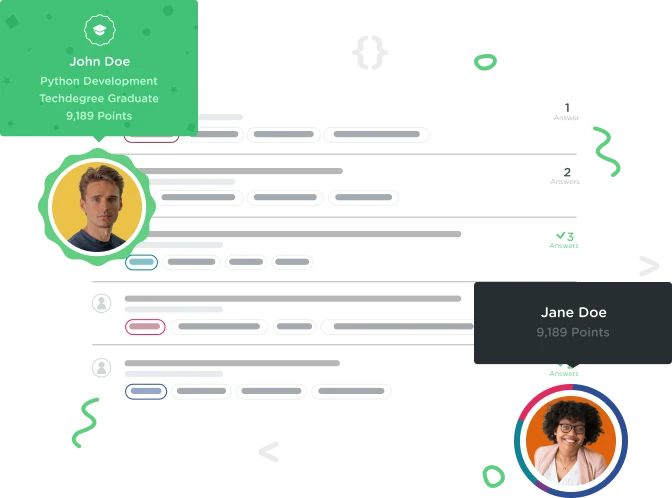

Daniel Salvatori
2,658 PointsSome assistance with this challenge
So for the student records function, I have gotten it to the point where it will print the records however I know I am doing something wrong. For one, I am using only a for loop, i'm pretty sure I should be using a while loop but whenever I try to use one it never prints anything to the page.
Also, when I search for 'Dave', the first object in the array, it prints just Dave's records, however all the other names will print their record PLUS all the other students that are in the array.
I am not sure how to proceed.
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function studentSearch (){
var search = prompt('Enter the name of the student you wish to search for.');
for (var i = 0; i < students.length; i += 1) {
student = students[i];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
if (search === student.name){
print(message);
} else if ( search === 'quit'){
break
} else {
alert('student not found')
}
}
}
studentSearch();
2 Answers

Neil McPartlin
14,662 PointsHi Daniel. I just see 2 issues.
Taking your last point first, the reason why you see additional students to the one you have matched is because at each 'for' loop, you are populating the 'message' variable with each student in the array. The solution is to simply move the 4
message +=
lines to just above theprint(message)
line, within the same code block.The
alert('student not found')
is going to pop up on every loop too, whether or not a match is found. So best to remove it from the for loop, and instead only show it after the loop condition has finished and only wheremessage == ''
meaning no match was found.
Final code is like so.
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function studentSearch (){
var search = prompt('Enter the name of the student you wish to search for.');
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name){
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
print(message);
} else if ( search === 'quit'){
break
}
} if (message == '') {
alert('student not found')
}
}
studentSearch();

Daniel Salvatori
2,658 PointsThanks Neil,
The placement of the message lines seems obvious now, don't know how I missed that.
But what about the loop: how would I get the prompt to keep coming up each time and perform a new search without having to reload the page? When I try to do a while loop I get stuck in an endless loop and workspace crashes .
Thanks in advance.

Neil McPartlin
14,662 PointsHi Daniel. You did mention the while loop, but working with your code, I was trying to get a 'one shot' search going. Since these videos were made, Dave (the teacher) has had to come back to update his teacher's notes to say that the functionality he was seeing then, will not be seen now due to updates in the way browsers handle the latest Javascript.
https://teamtreehouse.com/library/the-student-record-search-challenge-solution
That said, I have played a bit more with your code so yes, a while loop is working, but no, you won't see any search results until you type 'quit' to actually break the loop and see the result(s). I'm betting you have already been here but the new 'problem' hid your success from view.
Here's my rejig of your code.
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
var search = prompt('Enter the name of the student you wish to search for.');
if (search === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name){
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
print(message);
}
}
if (message == '') {
alert('student not found')
}
}