Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial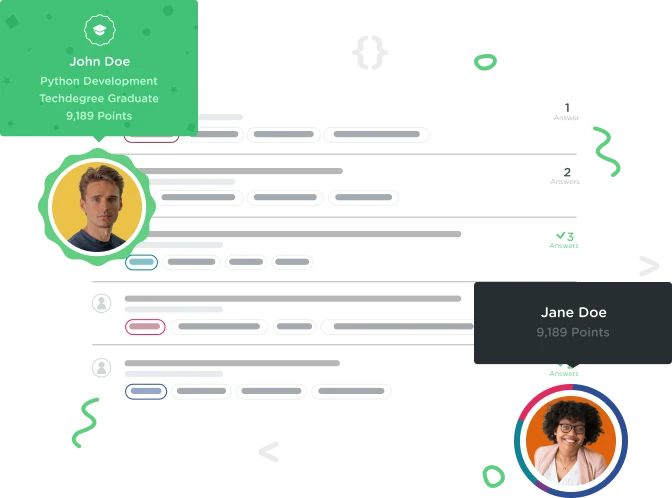
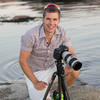
Marius Wallin
11,464 PointsSome help to understand the while in this one
vov = list("aeiouy") a_word = input("Remove vowels from my word: ") convert = list(a_word) output = []
for vow in vov: while True: try: convert.remove(vow) except: break output.append(''.join(convert).capitalize())
print(output)
The while loop inside the for loop, is it there to make sure that it will try to remove a vow until there is no vow left (it will only get an ValueError for each try) ant the try: returns false?
3 Answers
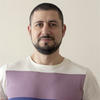
Daniel Petrov
3,495 PointsYes, they give a different outcome indeed. I tried another approach which worked without using a try/catch block and actually uses fewer steps. In Keneth's example probably the reason using it just to teach us another way to use an exception.
word_list = ['installation', 'motivation', 'initegration', 'computation']
vowels_list = ['a', 'e', 'i', 'o', 'u']
new_list = []
for word in word_list:
letter_list = list(word.lower())
for letter in letter_list:
while True:
if letter in vowels_list and letter in letter_list:
letter_list.remove(letter)
else:
break
new_list.append(''.join(letter_list).capitalize())
print(new_list)
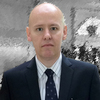
Nathan Tallack
22,160 PointsConsider the two blocks of code below, the first one being the nested for while with the break out of the while, the second one being a for loop with a continue. Both have the same functional outcome.
vov = list("aeiouy")
a_word = input("Remove vowels from my word: ")
convert = list(a_word)
output = []
for vow in vov:
while True:
try:
convert.remove(vow)
except:
break
output.append(''.join(convert).capitalize())
print(output)
vov = list("aeiouy")
a_word = input("Remove vowels from my word: ")
convert = list(a_word)
output = []
for vow in vov:
try:
convert.remove(vow)
except:
continue
output.append(''.join(convert).capitalize())
print(output)
I am not sure why they chose to use the nested loops in this video. Perhaps there is a reason to it that is not clear to us. But ultimately the lesson is about learning list mutation not control flow, so either way works for the purpose of the lesson. :)
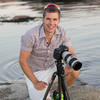
Marius Wallin
11,464 PointsThanks. That helped.
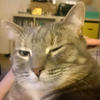
Rui W
6,269 PointsPoked at these two different approaches. I think they are eventually giving different outcomes:
The first one, or the "nested for while", is going to keep removing each of the vowels (in the order of 'aeiouy') until it fails on doing so. Meanwhile the second logic, "for loop with a continue", will only perform deletion of each of the vowels for ONE TIME.
For instance, using the word 'people', and we want to remove all vowels (2 e and 1 o) in it:
First logic
The result will be ['Pople', 'Popl', 'Ppl'] since the output variable is trying to store converted word after each vowel deletion. Here we can see the last item in the result list, or say the final version of de-vowelled word is the thing we're expecting.
What the code is doing in for loop (ignored .capitalize())
- try 'people'.remove('a'), which will return an error since there's no 'a', thus break, jump to next vowel;
- try 'people'.remove('e'), since we have "while True" there, this indicates - don't stop until "convert.remove('e')" returns an error. Thus both 'e' will be removed and now we only have 'popl' instead of 'people';
- try 'popl'.remove('i'), which will return an error since there's no 'i', thus break, jump to next vowel;
- try 'popl'.remove('o'), easy to see, 'o' will be removed and we get 'ppl'; 5...6...There 's no 'u' or 'y' in the remaining of the string.
Second logic
The result will be ['Pople', 'Pple'], we can see the last item here is still having an 'e' in it. This is because the code will continue processing to the next vowel after a successful .remove('e').
What the code is doing in for loop (ignored .capitalize())
- try 'people'.remove('a'), which will return an error since there's no 'a', thus break, jump to next vowel;
- try 'people'.remove('e'), found an 'e' in the string, removed it; then the code realize we are still in the for loop, so, goes to the next vowel!
- try 'pople'.remove('i'), which will return an error since there's no 'i', thus break, jump to next vowel;
- try 'pople'.remove('o'), easy to see, 'o' will be removed and we get 'pple'; 5...6...There 's no 'u' or 'y' in the remaining of the string.
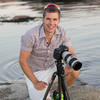
Marius Wallin
11,464 PointsRUI - thanks. Thats a very good response and made it much more clearer! ;)
Arpit Singh
942 PointsArpit Singh
942 Pointswill you please explain why this program is not removing all the variables
v = list('aeiou')
list1 =['adsgs','dgeiourhhhaaaaaa','howaejoihvnois'] e =[] for letter in list1: letter1 = list (letter) for l in letter1: while True: if l in v and l in letter1: letter1.remove(l) else: break e.append(''.join(letter1)) print(e)