Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial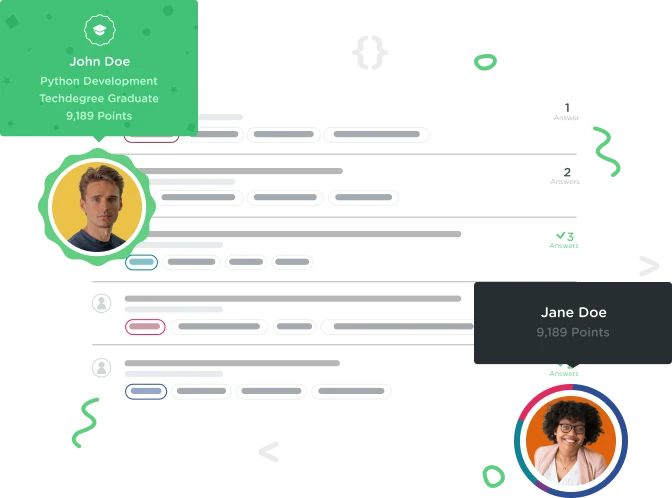

David Hernandez
Python Development Techdegree Graduate 12,181 PointsSome of this instructions are confusing.
trying to figure out how to complete this one but I'm not understanding something it's asking.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides=20)
class Hand(list):
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, number_of_dice):
hand = Hand()
for _ in number_of_dice:
hand.append(cls)
return hand
1 Answer
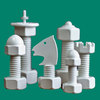
Steven Parker
241,808 PointsTry taking the instructions one step at a time, you already have several done:
- In the hands.py file, import the D20 class from dice.py
you forgot this one
- Create a classmethod named roll
you got this one
- Since it is a classmethod, it will take cls as an argument
- It will also take the number of dice as an argument
- create a new instance of the Hand class and assign it to a variable
- Append a D20 to your Hand equal to the number of dice given as an argument to the roll classmethod
- Then return the Hand of D20s
The part about "equal to the number of dice" can be done using a loop, appending as many times as the number given. Other than that, just do the include and return and you'll have it.