Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial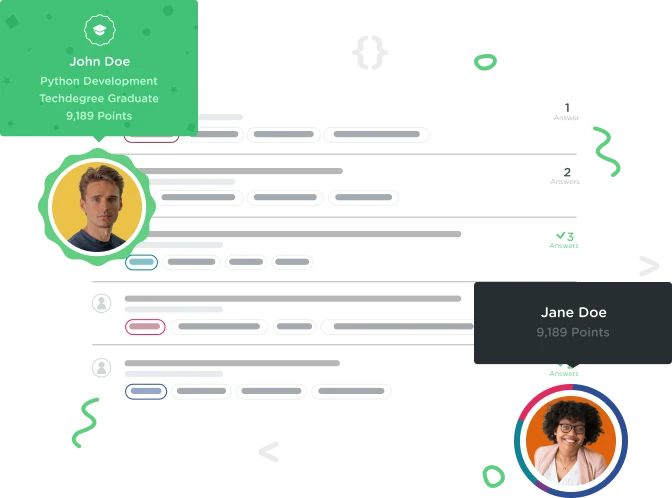

Kevin Dunn
6,623 PointsSome problems with my counter...
Hi, so I have some problems with my code. When giving an answer to questions that require the user to enter an integer, the counter works. But when the user is required to enter a string, it doesnt work. So for example the first question requires the user to enter number 10 as an answer, which in return adds 1 to the counter if correct. The second question requires the user to enter a string, but the counter doesnt add 1.
Also, any room for improvement?
Thanks.
Here below is my code.
var counter = 0;
var input1 = prompt("How much is 5 + 5?");
var answer1 = parseInt(input1);
if (answer1 === 10) {
counter = counter + 1;
}
var answer2 = prompt("Which country won the Euro 2016 Tournament?");
if (answer2.toUpperCase() === 'Portugal') {
counter = counter + 1;
}
var answer3 = prompt("What is the opposite of UP?");
if (answer3.toUpperCase() === 'Down') {
counter = counter + 1;
}
var input4 = prompt("How much is 20 * 3?");
var answer4 = parseInt(input4);
if (answer4 === 60) {
counter = counter + 1;
}
var answer5 = prompt("What is the color of the ocean?");
if (answer5.toUpperCase() === 'Blue') {
counter = counter + 1;
}
document.write('<p>' +'You got ' + counter + ' answers correct.'+'</p>')
if(counter === 5) {
document.write('<p>' +'Rank: Gold Crown!'+'</p>')
}
else if (counter >=3 && counter <=4) {
document.write('<p>' +'Rank: Silver Crown!'+'</p>')
}
else if (counter >=1 && counter <=2) {
document.write('<p>' +'Rank: Bronze Crown!'+'</p>')
}
else{
document.write('<p>' +'Sorry! You wont be recieving a crown. Try again.'+'</p>')
}
2 Answers
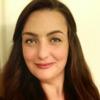
Jennifer Nordell
Treehouse TeacherHi there! Let me show you an example of the problem. Let's take a look at this line:
if (answer2.toUpperCase() === 'Portugal') {
This takes the user's answer and converts it to upper case. Let's say I'm the user and I input "porTUgal". It's going to turn my answer into "PORTUGAL". There is nothing that I can input that will make my answer explicitly equal to "Portugal" because it's turning my answer into all upper case. So all your answers need to be in upper case. In this example:
if (answer2.toUpperCase() === 'PORTUGAL') {
Hope this helps!
edited for additional note
Elvis Reinis Zeltītis is correct about your if else statements. Because this is an integer it's never going to be in between 3 and 4. It couldn't be say 3.7. You could do as he suggests and do a or statement, but I'd suggest simplifying it in this manner:
if(counter === 5) {
document.write('<p>' +'Rank: Gold Crown!'+'</p>')
}
else if (counter >=3) {
document.write('<p>' +'Rank: Silver Crown!'+'</p>')
}
else if (counter >=1 ) {
document.write('<p>' +'Rank: Bronze Crown!'+'</p>')
}
else{
document.write('<p>' +'Sorry! You wont be recieving a crown. Try again.'+'</p>')
}

Elvis Reinis Zeltītis
1,925 PointsHey!
First thing I noticed was, but its not that important, and it doesnt change the functionality of the program
counter = counter + 1;
You can replace it with
counter +=1
And theres a big issue. You dont want it to check if both are true you want to make it so it checks if atleast one of them is true. Because you wont number 3 and 4 at the same time.
else if (counter >=3 && counter <=4) {
document.write('<p>' +'Rank: Silver Crown!'+'</p>')
}
The fix for this would be:
else if (counter >=3 || counter <=4) {
document.write('<p>' +'Rank: Silver Crown!'+'</p>')
}
// from else if (counter >=3 && counter <=4) {
// to else if (counter >=3 || counter <=4) {
Can't spot anything else, so far good I think! :) Maybe others will see something my eye didn't catch! Good luck on your further coding! :)
If I said something wrong please dont judge me and correct me :)

Kevin Dunn
6,623 PointsThanks!!!
Kevin Dunn
6,623 PointsKevin Dunn
6,623 PointsThanks a lot!