Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial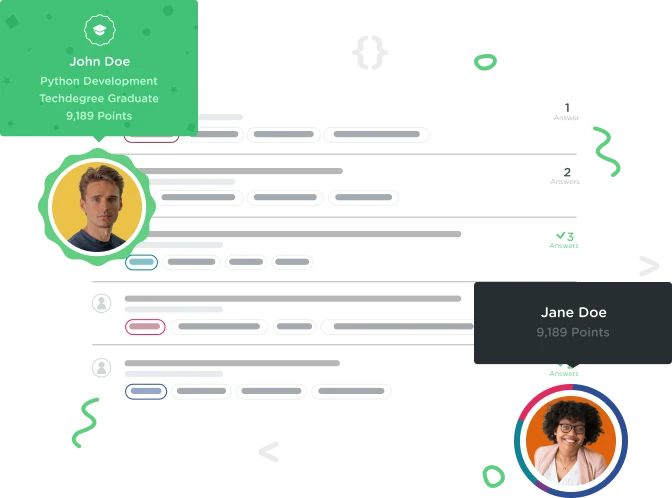
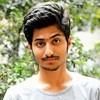
Saud Tauqeer
Courses Plus Student 8,099 Pointssome questions
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);}// INSTEAD OF THIS
//========= why cant we do this? ===================
response.on('end', () => {
try {
// Parse the data
body += data.toString();
const profile = JSON.parse(body);}//
like there might be some processing we might want to implement only when we actually get the chunk of data we get from the sv. is that all or is there some more to it?
as for my second question
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//Require https module
const https = require('https');
//Print Error Messages
function printError(error) {
console.error(error.message);
}
//Function to print message to console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
console.log(message);
}
function getProfile(username) {
try {
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
}//here ; catch (error) {
printError(error);
}// and here ;
});
});
request.on('error', printError);
} catch (error) {
printError(error);
}
}
const users = process.argv.slice(2);
users.forEach(getProfile);
if you look at this code there's no closing ' ; ' on the try and catch and how can we know what is the scope of the try and catch. also is it necessary to use try and catch both together. i mean what would happen if you only use try and not catch or if you only use catch but not try.. i have commented it out in the 2nd code. another question the 'error' in the catch's paramater is the error from the global scope right? like same error as when we say error.message. id like to improve so feel free to suggest/give your opinion. thank you.
2 Answers
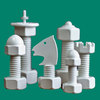
Steven Parker
231,268 PointsThe data chunks arrive with the "data" events. When you get the "end" event, it confirms that you now have all the data, but it doesn't pass any data itself. It takes both handlers to do the whole job.
The scope of the "try" is within the following block enclosed in braces. The "try" and "catch" statements always work together, neither one is useful by itself.
The "error" is like a function parameter — it is supplied by the exception. It's scope is limited to the "catch" block.
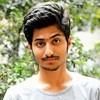
Saud Tauqeer
Courses Plus Student 8,099 Pointsi see. thanks a lot as usual. btw one more thing why we dont close try code block and catch with ; ? also if you use
try{
//code
}
//code
catch (error) {
//code
}
will this still refer to the try mentioned above? so try only prevents from spittting out a stack error?
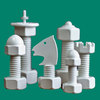
Steven Parker
231,268 PointsSemicolons are used to end statements, but they are not used after code blocks as you might see after a "try", "catch", "if" and "else".
And there should not be any code between the "try" block and the "catch" that follows it.
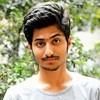
Saud Tauqeer
Courses Plus Student 8,099 PointsThanks a ton mate

Bilal Bajwa
6,212 Pointswhat is the answer to || means?
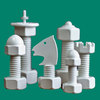
Steven Parker
231,268 PointsFor future questions, always start a fresh question of your own instead of asking a new question as an "answer".
But the "||" symbol is the logical "OR" operator, often used for testing more than one condition in a single expression. For more details, see this MDN page on Logical Operators.
Saud Tauqeer
Courses Plus Student 8,099 PointsSaud Tauqeer
Courses Plus Student 8,099 PointsSteven Parker
there are two catches used in this code. once in the if else statment and one catch after that why is that?