Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial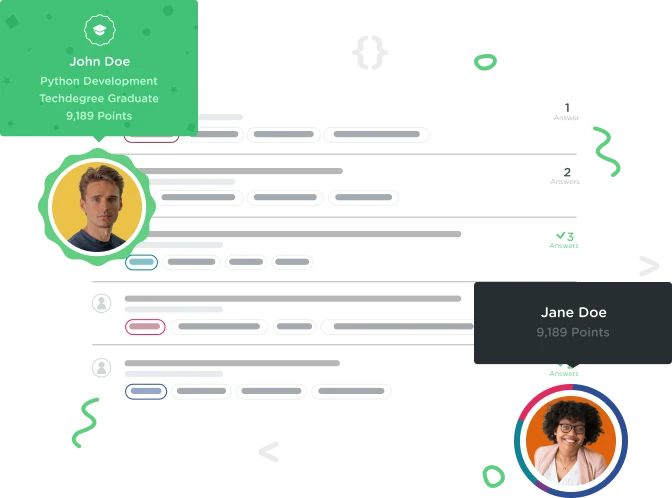

Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsSomehow my parameters are not getting read and I'm getting an array index out of bounds exception
Hello, I'm trying to pass the parameters itemName and maxNumberOfItems into my Jar class. I tried to instantiate a new Jar named jar in my main method thinking that the code from the Jar class to import the scanner and get the two variables would be called. However, I'm getting an Array Index Out of Bounds Exceptions when I call the Jar method (with parameters) in my main method, so I think that is because it is not prompting the user to enter a itemName and maxNumberOfItems like it is supposed to. Because they are not being prompted to enter anything, I believe this is why the arguements are empty??
My questions is: Why is my code for the scanner and itemName and maxNumberOfItems not working? I want the user to be prompted to enter a type of item and max number of items that can fit in a jar. Is this supposed to be in the Jar class like I have it?
MAIN CLASS:
import java.util.Scanner;
public class GuessingGame {
public static void main(String[] args) {
System.out.println("ADMINISTRATOR SETUP\n********************");
Jar jar = new Jar(args[0],args[1]);
}
}
JAR CLASS:
import java.util.Scanner;
import java.util.Random;
public class Jar {
String itemName;
public Jar(String itemName, String maxNumberOfItems) {
Scanner scanner = new Scanner(System.in);
itemName = scanner.next("What type of item?");
maxNumberOfItems = scanner.next("What is the maximum amount of " + itemName + "?");
}
}
CONSOLE OUTPUT:
ADMINISTRATOR SETUP
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 0
at GuessingGame.main(GuessingGame.java:9)
It looks like my code is running fine until it receives the empty arguments. How do I make these empty arguments filled with the results from the scanner prompts?
2 Answers

Jeff Wilton
16,646 PointsI can explain a few things and help you out.
First lets talk about how parameters are read into your program. When you use the String args array in the Main method, you are expecting the args to be passed in via the command line when you start your program like this:
java GuessingGame pencil 40
In the above example, the two arguments are pencil and 40, which would then be used in your Jar constructor, and there would be no point in prompting the user for these values since you would already have them.
However, I don't believe this was your intent. I think you intended to simply start your program, and then prompt a user to enter the itemName and maxNumberOfItems. I modified your classes a bit and actually allowed for either case, if you enter the args when starting the program, it will construct a new Jar with those inputs, print out the results and be done. If the program is started without any args, it will then prompt you to enter the itemName and maxNumberOfItems and then assign those values to the newly constructed Jar. Please take a look at the following classes and let me know if there are any questions:
GuessingGame.java
public class GuessingGame {
public static void main(String[] args) {
System.out.println("ADMINISTRATOR SETUP\n********************");
if(args.length == 2) {
Jar jar = new Jar(args[0],args[1]);
}
else {
Jar jar = new Jar();
}
}
}
Jar.java
import java.util.Scanner;
public class Jar {
private String itemName;
private String maxNumberOfItems;
public Jar(String itemName, String maxNumberOfItems) { //constructor with two args
this.itemName = itemName;
this.maxNumberOfItems = maxNumberOfItems;
System.out.println("A new Jar has been constructed with " + this.maxNumberOfItems + " " + this.itemName + " items");
}
public Jar() { //constructor with no args
System.out.println("What type of item?");
Scanner scanner = new Scanner(System.in);
this.itemName = scanner.nextLine(); //'this' isn't required but lets use it to be clear
System.out.println("What is the maximum amount of " + itemName + "?");
this.maxNumberOfItems = scanner.nextLine();
System.out.println("A new Jar has been constructed with " + this.maxNumberOfItems + " " + this.itemName + " items");
}
}

Seth Kroger
56,413 PointsAs mentioned, you're getting an out of bounds error because you're accessing args without checking its length first. I would also strongly recommend not using system I/O in a constructor. The constructor should only be for setting up the initial values of the object. If you don't know what values to use and don't have sensible defaults in mind, then you should prompt the user first before calling the constructor. There can be other means of prompting the user than System.in and out, so it's a good idea to keep that part separate from the model/logic.

Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsI appreciate your reply. I didn't realize it was necessary to check the length of the args first as mentioned by you and Jeff. I see what your saying about keeping the prompting separate like with making a new console class for example. But why is it better to separate the two if the code runs the same both ways?
Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsJennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsJeff, I really appreciate your help! ::)
I was still having trouble putting two args in the constructor, so I decided to write the constructor without it. But I wanted to also make sure I'm understanding how the code works below.
My interpretation of this ^^ code is that because public "Jar()" was created in the Jar class that it is considered a constructor. It is also considered a constructor because 'jar' begins with a capital 'J' like in the class name, and it has no return value. And because I've created a constructor, that instance of the class (found in constructor) will be called every time I create a new instance of the class.. Is that correct? What is the purpose of defining the (this.) method? Is there a System.out.println I can use now to check my newly created jar and ensure that the values that the user was prompted to add were put in there? I just want to make sure I have it right before moving forward. I couldn't get the code to compile like this :
So I wrote my if statement like this, and want to make sure it didn't mess up the functionality of my program.