Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial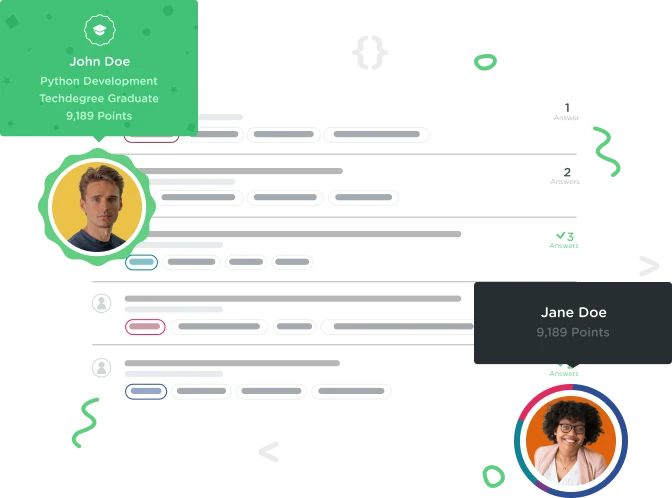

Sara Pasinato
1,023 PointsSomeone can help me ??
Now let's use your validation skills. Only letters and the $ symbols are allowed in the discount code. Check Example.java for use cases
In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
if ((! discountCode.isLetter(discountCode.length())) || ( discountCode.indexOf(0)!='$')){
throw new IllegalArgumentException("invalid discount code.");
}
return String.toUpperCase(discountCode);
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
6 Answers

Thomas Nilsen
14,957 PointsThat's because I thought only one dollar-sign was allowed.
If multiple is allowed, then this should be enough:
private String normalizeDiscountCode(String discountCode){
for(char c : discountCode.toCharArray()) {
if(!Character.isLetter(c) && c != '$') {
throw new IllegalArgumentException("invalid discount code.");
}
}
return discountCode.toUpperCase();
}

Craig Dennis
Treehouse TeacherThe indexOf
command looks for a specific character in the string and returns -1
if it is not found.
The argument and expected return value appear to be swapped here.
discountCode.indexOf(0)!='$'
That help?

Thomas Nilsen
14,957 PointsA couple of things is wrong here:
You pass length to isLetter like so:
discountCode.isLetter(discountCode.length()))
This will always return false.
Also, You can't just check if the first character is dollar-sign, as it could appear later in the string.
You need to loop through the string and make sure it is all letters or $, and that the dollar sign appear only once.
I haven't tested but this should work:
private String normalizeDiscountCode(String discountCode){
int numberOfDollarSigns = 0;
for(char c : discountCode.toCharArray()) {
if(c == '$') numberOfDollarSigns++;
if((!Character.isLetter(c) && c != '$') || numberOfDollarSigns > 1) {
throw new IllegalArgumentException("invalid discount code.");
}
}
return discountCode.toUpperCase();
}

Sara Pasinato
1,023 PointsThank you for the answer!! ? But there is this error: Bummer! Hmmm...order.applyDiscountCode("$aviNg$") threw an exception, when it shouldn't have

Cody Rikard
2,191 PointsThis worked for me after making this adjustment to the code.
private String normalizeDiscountCode(String discountCode){ int numberOfDollarSigns = 0; for(char c : discountCode.toCharArray()) { if(c == '$') numberOfDollarSigns++; if(!Character.isLetter(c) && c != '$') { throw new IllegalArgumentException("invalid discount code."); } }
return discountCode.toUpperCase();
}

Sara Pasinato
1,023 PointsNow is better!! ? but the problems is the class String end the return value . This is the errors:
/Order.java:13: error: cannot find symbol if ((! String.isLetter(discountCode.length())) || ( discountCode.indexOf(0)== -1)){ ^ symbol: method isLetter(int) location: class String ./Order.java:18: error: incompatible types: String cannot be converted to Locale return String.toUpperCase(discountCode); ^ Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. Note: Some messages have been simplified; recompile with -Xdiags:verbose to get full output 2 errors

Thomas Nilsen
14,957 PointsIt's not
String.toUpperCase(discountCode);
it is
return discountCode.toUpperCase();

Sara Pasinato
1,023 Points? But there is this error: Bummer! Hmmm...order.applyDiscountCode("$aviNg$") threw an exception, when it shouldn't have

Stephanie Youstra
18,513 PointsCraig Dennis ~ I appreciate your presence in this answer, so I'm responding here .... as I look at all the different times this question came up in the forum, I keep seeing the similar answer of creating an array and looping through it. However, since we have not yet been introduced to arrays in this Introductory Java track {that will come three video segments later}, I'm wondering if there is a particular way you were expecting us to do it. As I do each of these code challenges, I try to solve them not through external knowledge and googling, but rather through application of the previous video segments instruction {obviously, I know that previous knowledge and Google are important; I just assume that these are designed to be practice of the specific skills just taught}. However, in this case ..... am I missing something? I'm not seeing how to loop through and check for letters or $s just by applying the previous segments' lessons.

Stephanie Youstra
18,513 PointsDagnabbit .... as I just looked at my Hangman code again, I saw the toCharArray. I apologize profusely for jumping the gun in my crankiness -- I just have spent too long staring at the wrong parts of my code! :-( :-( :-(

Craig Dennis
Treehouse TeacherYou had me at dagnabbit ;) No worries at all!
Looping through the characters from getCharArray
is indeed how I intend you to solve the issue.
Sara Pasinato
1,023 PointsSara Pasinato
1,023 Pointsthanks for the answer it right !! ?