Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial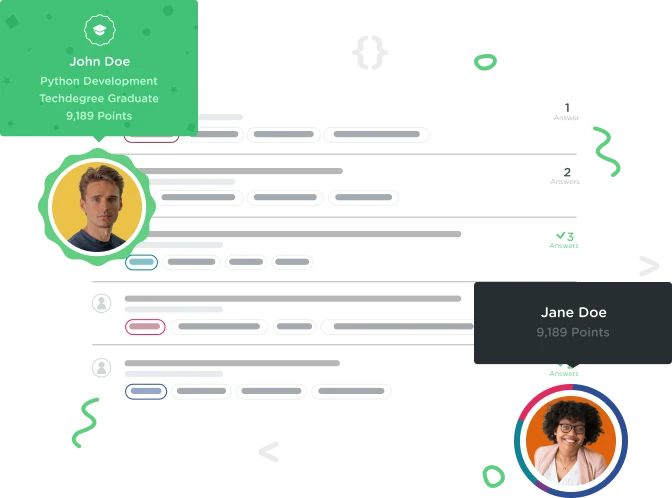
Lido Fernandez
10,556 PointsSomeone please can send/provide me a message/link with the explanation of this method?: renderInElement
I use it for the built the PlayList Challenge but I still do not get what it does.
3 Answers

LaVaughn Haynes
12,397 PointsIn the HTML you have an ordered list <ol> with an id of "playlist".
<div>
<h1>Treetunes</h1>
<ol id="playlist">
</ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
</div>
We can grab that ordered list element and store it as the variable playlistElement, and then pass it to renderInElement
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
renderInElement deletes anything in the the ordered list and then loops through all of the songs, generates the appropriate HTML for each song and puts it inside the ordered list. Think of it as updating the playlist on the screen. It deletes the old rendering of the list and redraws it.
Playlist.prototype.renderInElement = function(list) {
//delete any html inside the our ordered list by setting it to an empty string
list.innerHTML = "";
//loop through each song
for(var i = 0; i < this.songs.length; i++) {
//generate an HTML element for each song and put it inside the ordered list
//toHTML() explained below
list.innerHTML += this.songs[i].toHTML();
}
};
This is the part that generates HTML for each song. As you see it makes a list element <li> which contains information for a song
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - '
htmlString += this.artist;
htmlString += '<span class="duration">'
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
Hopefully this helps. You can also re-watch where he talks about around the 2 minute mark here https://teamtreehouse.com/library/objectoriented-javascript/constructor-functions-and-prototypes/making-the-ui-work
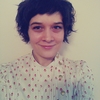
Kristopher Van Sant
Courses Plus Student 18,830 PointsHi! I found a few forum posts, from a few months ago, that talk about this method. Hope they help!
https://teamtreehouse.com/community/how-does-the-renderinelement-method-work
https://teamtreehouse.com/community/why-do-we-need-to-call-renderinelement-in-the-onclick-functions

isral duke
7,315 Pointsi had the same question as Lido. After some thought i finally realized that the array's contents (all of the elements in the array) have to be output to HTML. Sometimes it helps to think about the code for a while to make it click
in the greybox.