Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial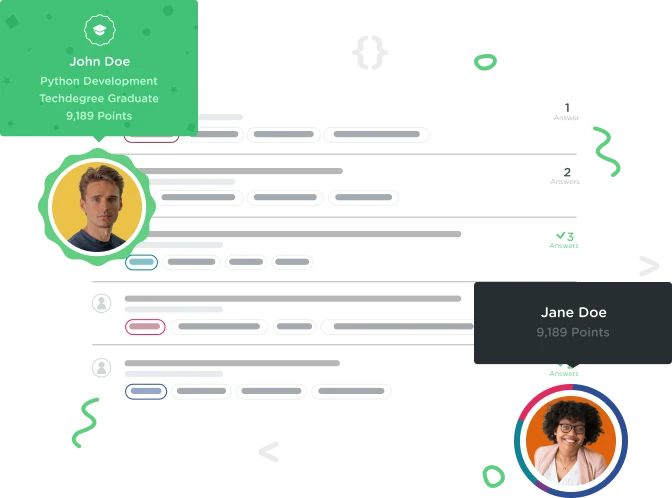

Ken Del Valle
976 PointsSomeone please help with this alternative
This is what I have
alert('Welcome to Randomize!');
var lowerNumber = parseInt(prompt("Type your lowest number."));
var upperNumber = parseInt(prompt("Type your highest number."));
if (lowerNumber < upperNumber) {
function randomNumber(lowerNumber, upperNumber) {
return Math.floor(Math.random() * (upperNumber - lowerNumber + 1)) + lowerNumber;
}
} else {
console.log('It did not work!')
}
confirm(randomNumber());
Can someone help me with this? I want to use prompts to determine the two numbers.
2 Answers
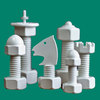
Steven Parker
231,269 PointsThe prompts appear to be working fine, but there are other aspects of this code that will cause errors (or are odd):
- if the numbers are given in the wrong order, the function isn't defined but an attempt to call it is still made
- even when the function is defined, the call doesn't provide it with any arguments (it needs 2)
- "confirm" should only be used instead of "alert" when the response will be tested

Adam Suchorzebski
7,600 PointsHi Ken,
I'm beginner when it comes to coding, but that's how I'd do it:
alert('Welcome to Randomize!');
var lowerNumber = parseInt(prompt("Type your lowest number."));
var upperNumber = parseInt(prompt("Type your highest number."));
function randomNumber(lowerNumber, upperNumber) {
return Math.floor(Math.random() * (upperNumber - lowerNumber + 1)) + lowerNumber;
}
if (lowerNumber < upperNumber) {
console.log( randomNumber(lowerNumber, upperNumber) );
}
else {
console.log('It did not work!')
}
So here we have 2 variables in the global scope, which are the outcome of user's input.
Then there's a conditional statement that checks whether the upperNumber
variable is a higher number than lowerNumber
. If it's true, then it calls the function, gets back to it, executes the code and returns with the value that is printed in the console. If the condition of the statment is not met - the upperNumber
is not higher than lowerNumber
then it returns with console.log 'It did not work!'
I'm not really sure what that confirm
at the end is for. Basically as far as I'm aware, it's something you put into conditional statement.