Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial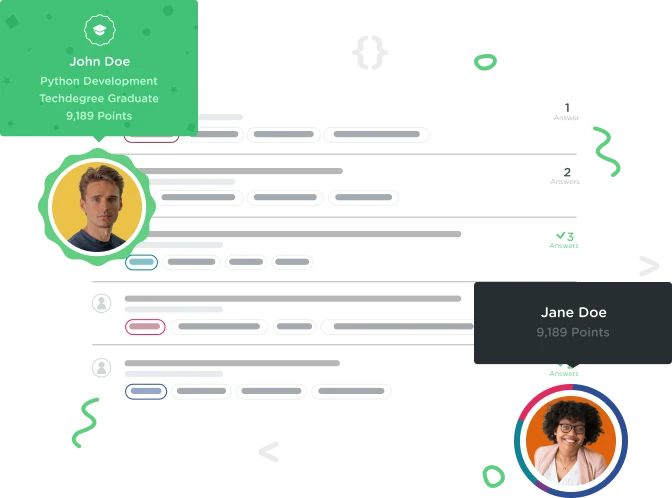

Alfred Moha
8,205 Pointssomeone to help here
def filter_col_by_string(data_sample, field, filter_condition): filtered_rows = []
col = int(data_sample[0].index(field))
filtered_rows.append(data_sample[0])
for item in data_sample[1:]:
if item[col] == filter_condition:
filtered_rows.append(item)
return filtered_rows
import csv
def open_with_csv(filename, d='\t'):
data = []
with open(filename, encoding='utf-8') as tsvin:
tie_reader = csv.reader(tsvin, delimiter=d)
for row in tie_reader:
data.append(row)
return data
def filter_col_by_string(the_data, field, filter_condition):
filtered_rows = []
#find index of field in first row
col = int(the_data[0].index(field))
filtered_rows.append(the_data[0])
for row in the_data[1:]:
if row[col] == filter_condition:
filtered_rows.append([str(x).encode('utf8') for x in row])
return filtered_rows
data_from_csv = open_with_csv('data.csv')
# YOUR CODE HERE
def filter_col_by_string(data_sample, field, filter_condition):
filtered_rows = []
col = int(data_sample[0].index(field))
filtered_rows.append(data_sample[0])
for item in data_sample[1:]:
if item[col] == filter_condition:
filtered_rows.append(item)
return filtered_rows
silk_ties = filter_col_by_string(data_from_csv, "material", "_silk")
print("Found {} silk ties".format(number_of_records(silk_ties)))
wool_ties = filter_col_by_string(data_from_csv, "material", "_wool")
print("Found {} wool ties".format(number_of_records(wool_ties)))
cotton_ties = filter_col_by_string(data_from_csv, "material", "_cotton")
print("Found {} cotton ties".format(number_of_records(cotton_ties)))
1 Answer

KRIS NIKOLAISEN
54,972 PointsTo solve this challenge you will only need to add one line of code. You will be using the given function filter_col_by_string()
and for the most part just need to figure out what to pass in as arguments
Some hints:
- assign the result of the function to
dkny_ties
- you have
the data
correct withdata_from_csv
- the field is given in the hint as "brandName"
- the filter condition is specified in the instructions as "DKNY"