Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial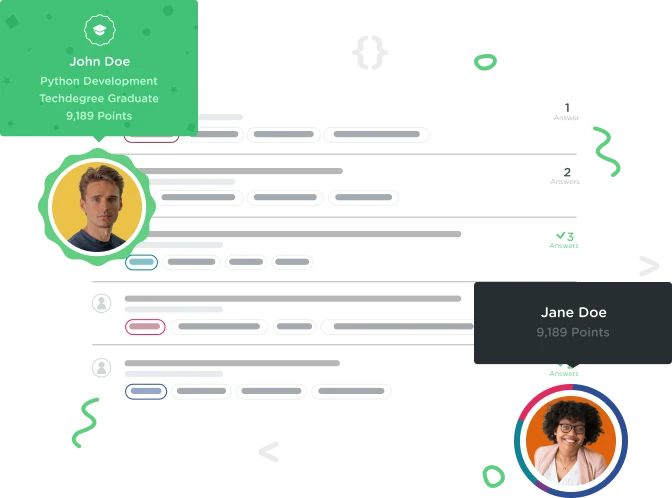

wayne ross
3,225 PointsSomething I am missing with the treehouse grading scheme on this challenge
For some reason this works for any set I pass into it using an outside test but treehouse tells me 'Bummer: Didn't get the right output from 'covers'. For outside test like workspace, online IDEs only difference that I am calling the covers function differently i.e. covers(arg1, COURSES) since I am passing in the dict.
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(arg1):
retVal=[]
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
for subject, classes in COURSES.items():
if arg1.issubset(classes):
retVal.append(subject)
return retVal
2 Answers
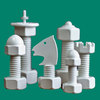
Steven Parker
231,269 PointsWhen they say "overlap", they mean any items in common. A subset would be a set that has all items in common.
So for this task, an intersection would be a better test. If the intersection of two sets is non-empty, you can say that the sets "overlap".
You also don't need to repeat the definition of "COURSES".
Keep the subset in mind for the next task.
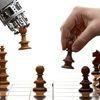
horus93
4,333 PointsSo from what I'm reading here obviously intersection would be a much cleaner way of doing it, but since this does the same thing from what I can tell (except, not exclusively for sets), is that maybe why my code here isn't passing?
def covers(items):
mylist = list()
for k, v in COURSES.items():
if all(True if item in v else False for item in items):
mylist.append(k)
print(mylist)
return mylist
covers({'Python'})
At first I thought it was failing because I wasn't able to get set input working as the functions argument, but after that was fixed it still gave me the same "wrong output from covers" message, which seemed weird because when I test it with a variety of different set value inputs from the list of subjects in the classes, they always come back with the right lists.
wayne ross
3,225 Pointswayne ross
3,225 PointsYup that did the trick
wayne ross
3,225 Pointswayne ross
3,225 PointsSteven why does using if arg1.intersection(classes) don't pass? Is it because that method does not return a Boolean or something along those lines
Steven Parker
231,269 PointsSteven Parker
231,269 PointsPerhaps you had a typo. I was able to pass using "
if arg1.intersection(classes):
"wayne ross
3,225 Pointswayne ross
3,225 PointsSteven I think I had the right concept of why that using sets.intersection wouldn't work in that syntax. The return from the method is different that the Boolean operator so the syntax would have to be different, below is how I got it to work using sets.intersection
Steven Parker
231,269 PointsSteven Parker
231,269 PointsI would think that might cause the final list to have redundant entries. I was able to pass the challenge with this:
This works because from Python's viewpoint, an empty set is "falsey" and a non-empty set is "truthy".
wayne ross
3,225 Pointswayne ross
3,225 PointsSteven for some reason I kept getting a fail with that code which brought my follow on question. I got another though with the one that I posted. I will say that that particular arg1.intersection(classes) code you used was one that was working for me in the outside IDE but it wouldnt pass the set.py test so I did some revisions and was able to pass the other way. not sure what is going on but ill play with that some more after wrapping up this track. Thanks for the great input!