Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial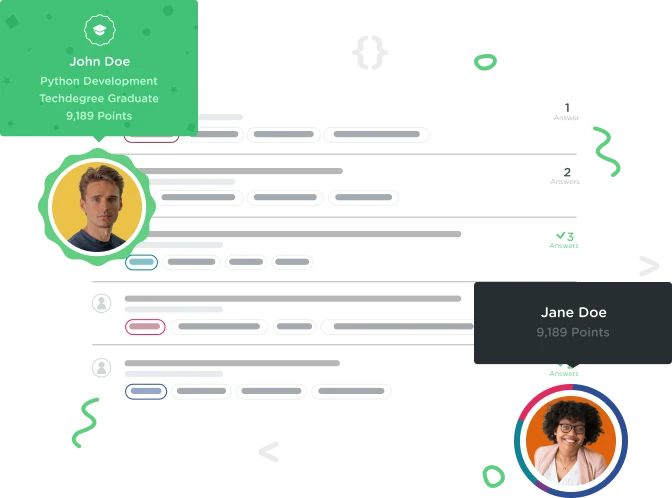

Anthony Prunsky
Front End Web Development Techdegree Student 8,725 PointsSomething is wrong! but not sure whats is ?
Well, why this not working, the site say its not correct but the function return the correct output! function reverse_evens!
lists = [1,2,3,4,5]
def first_4(lists):
return lists[:4]
def first_and_last_4(lists):
return lists[:4] + lists[-4:]
def odds(lists):
return lists[1::2]
def reverse_evens(lists):
return lists[-1::-2]
first_4(lists)
first_and_last_4(lists)
odds(lists)
reverse_evens(lists)
2 Answers
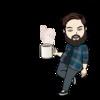
Philip Schultz
11,437 PointsHello, First, you will have to check what the length of the list is to see if it is even or odd. You can find out if the list has an even or odd number of indexes by using the modulo operator (%) and the number 2 and then testing if the value is equal to zero (or 1).
if len(list) %2 == 0:
Essentially what this is saying is - if I take the length of the list and divide it by two what will the remainder be. IF it equal to zero, then it must be an even number and anything else must be odd. Here is some more info on the modulo operator: https://stackoverflow.com/questions/4432208/how-does-work-in-python
Here is how I solved it, let me know if you have questions.
def reverse_evens(list):
if len(list) %2 == 0:
list2 = list[-2::-2]
else:
list2 = list[-1::-2]
return list2
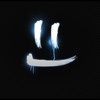
Grigorij Schleifer
10,365 PointsHi Anthony, I like Philips approach it has a nice logic behind it. I have an alternative approach and you can also both.
- If you like a shorter version just extract the even items and reverse them in the second step
# verbose version
def reverse_evens(list):
# get the evens first
# start: index 0, end: last index, step: every second index
even_items = list[::2]
# entire list get reversed using step -1
return even_items[::-1]
A more tidy way:
def reverse_evens(list):
return list[::2][::-1]
I hope that helps
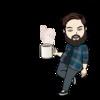
Philip Schultz
11,437 PointsThis is awesome. .. can't believe I didn't think of it this way. Much more simple.