Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial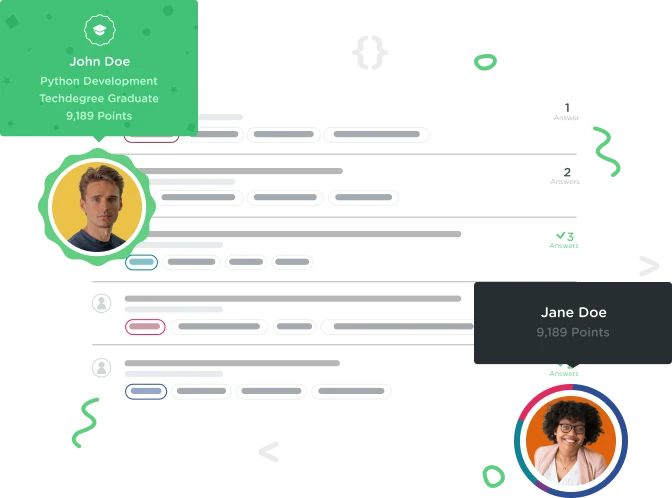
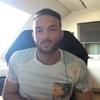
Johann Peters
40,749 PointsSomething's messed up in this challenge!?
Challenge description asks for a firstLetter function. The error message says I need to declare a firstName function! Can anybody help me out?
// Enter your code below
extension String{
func modify(operation: String -> String) -> String{
return operation(self)
}
}
func firstName(String -> String){
return String.characters.prefix(1)
}
3 Answers

pascalac
237 PointsHi Johann,
the function firstName should get a String as a parameter and return a String. You wrote a function that gets a closure as parameter and has no return type.
Something like this should be fine:
// Enter your code below
extension String{
func modify(operation: String -> String) -> String{
return operation(self)
}
}
func firstName(input: String) -> String { // No closure as parameter here, just a normal String and return String
return String(input.characters.prefix(1)) // You need to cast the result of prefix back to String
// return String(input.characters.first!) // Other possible solution
}
I hope I could help. :)

Alistair Cooper
Courses Plus Student 7,020 PointsI tried using characters.first , which didn't work. Does anyone have any ideas why? (I see in xcode that it returns an optional might that have something to do with it?)
extension String {
func modify(s: String -> String) -> String {
return s(self)
}
}
func firstLetter(s: String) -> String {
return s.characters.first
}

pascalac
237 PointsYes it's because .first returns an optional and so it doesn't match with your return type (String? != String). So you need to unwrap it first. If you're 100% sure that there'll be always a value inside you can just say "s.characters.first!". And I think you've to cast this back to String as well but I'm not sure about that. I've added the .first solution to my previous answer :)

Alistair Cooper
Courses Plus Student 7,020 PointsThanks Pascalac! That makes sense. -A