Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial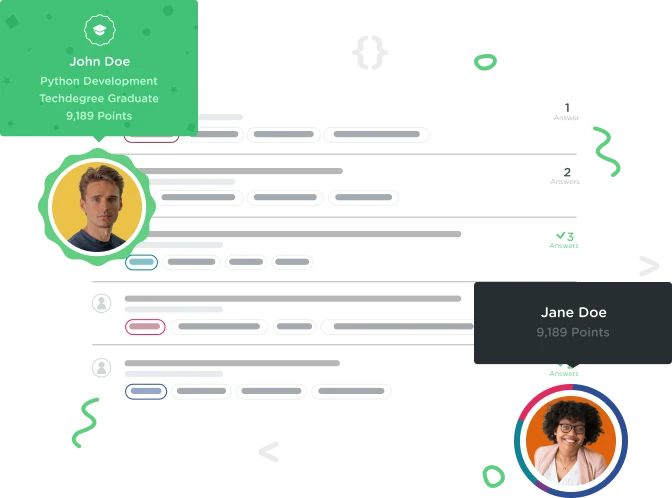

ange yannick nimba
1,689 PointsSomething's wrong with this script. The value in the variable money is 9. But if you preview this script, the browser di
const money = 9;
const today = 'Friday'
if ( money > 10 && today === 'Friday' ) {
alert("Time to go to the theater.");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner.");
} else if ( today == 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out.");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
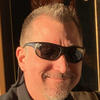
Peter Vann
36,427 PointsHi Ange!
The trick is really to think about the logic!
It looks like you're on the right track (2 out of 3).
This passes:
const money = 9;
const today = 'Friday'
if ( money > 10 && today === 'Friday' ) {
alert("Time to go to the theater.");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner.");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out.");
} else {
alert("This isn't Friday. I need to stay home.");
}
But this would be even better logic, really:
const money = 9;
const today = 'Friday'
if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner.");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time to go to the theater.");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out.");
} else {
alert("This isn't Friday. I need to stay home.");
}
Because the first way, if you had $100.00 it would suggest going to the theater, but you can actually afford dinner and a movie!?! Does that make sense?
(BTW, the second way passes also...)
I hope that helps.
Stay safe and happy coding!
Blake Larson
13,014 PointsBlake Larson
13,014 PointsThe first else if is now passing in your code because one of these conditions is passing.
money >= 50 || today === 'Friday'
You want both those to pass in order to go to movie and dinner.