Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial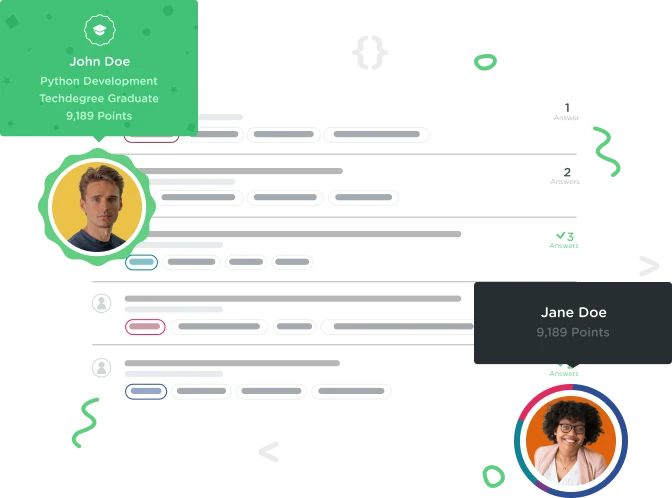

aycee morrow
1,767 PointsSomething's wrong with this script. The value in the variable money is only 9. But if you preview this script you'll see
Something's wrong with this script. The value in the variable money is only 9. But if you preview this script you'll see the "Time to go to the theater" message. Fix this script so that it correctly tests the money and today variables and prints out the proper alert message: "It's Friday, but I don't have enough money to go out"
var money = 9;
var today = 'Friday';
if (money === 9 && today === 'Friday') {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
6 Answers
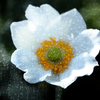
ellie adam
26,377 Pointsconst money = 9; const today = 'Friday'
if ( money > 10 && today === 'Friday' ) {
alert("Time to go to the theater.");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner.");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out.");
} else {
alert("This isn't Friday. I need to stay home.");
}
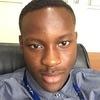
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsIn the challenge, you're asked to test the 'money' and 'today' variables which means you need to use the AND operator(&&) in all the clauses in the conditional statement. Then find a way to tweak the 'today' variable and the clause to print "It's Friday, but I don't have enough money to go out". This is one way to go about it:
var money = 9;
var today = 'Monday';
if (money >= 100 && today === 'Friday') {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money > 0 && today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Notice how I changed the value of the 'today' variable and introduced a new test condition(money > 0) in the last else-if clause just to print out "It's Friday, but I don't have enough money to go out".
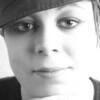
Leanne Millar
7,726 Pointsconst money = 9;
const today = 'Friday'
if ( money > 10 && today === 'Friday' ) { //Change or operator to && and
alert("Time to go to the theater.");
} else if ( money >= 50 && today === 'Friday' ) { //Change or operator to && and
alert("Time for a movie and dinner.");
} else if ( money <10 && today === 'Friday' ) { // if MONEY is less than <10 and && TODAY === is definitely equal to Friday
alert("It's Friday, but I don't have enough money to go out.");
} else {
alert("This isn't Friday. I need to stay home.");
}
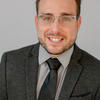
Travis Batts
4,031 PointsWell Osaro while your method does produce the correct response it does not quite make sense. I do think the challenge wants the today variable to === "Friday". Otherwise, the alert message does not make sense.. Because you're changing the variable to Monday and the alert messages say it's Friday..
So in my opinion the best way to do this would be to do else if ( money < 10 && today === "Friday") that way the alert message makes more sense.
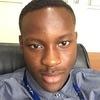
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsGood point there Travis, I noticed that the alert message didn't really tally with the changes I made to complete the challenge. Lol I guess I was too carried away with completing the challenge.
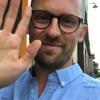
Kalle Jungstedt
4,709 PointsI´m exactly doing what you are suggesting but I´m still getting error messages on this one. Is there a bug lurking around here?
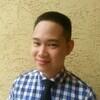
Tom Nguyen
33,500 PointsWhat I used to pass:
const money = 9;
const today = 'Friday'
if ( money > 10 && today === 'Friday' ) {
alert("Time to go to the theater.");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner.");
} else if ( today === 'Friday' && money <= 10) {
alert("It's Friday, but I don't have enough money to go out.");
} else if ( today !== 'Friday' ){
alert("This isn't Friday. I need to stay home.");
}

TechGenius App
10,060 PointsHey Kalle,
You may want to try this;
<p>
var money = 9;
var today = 'Friday';
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 10 && today === "Friday" ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
</p>
```

Mansor Almossa
Full Stack JavaScript Techdegree Student 2,525 Pointsthank you that works
ROBERT BUTLER
2,010 PointsROBERT BUTLER
2,010 PointsThis is correct.
const money = 9; const today = 'Friday'
if ( money > 10 && today === 'Friday' ) { alert("Time to go to the theater."); } else if ( money >= 50 && today === 'Friday' ) { alert("Time for a movie and dinner."); } else if ( today === 'Friday' ) { alert("It's Friday, but I don't have enough money to go out."); } else { alert("This isn't Friday. I need to stay home."); }