Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial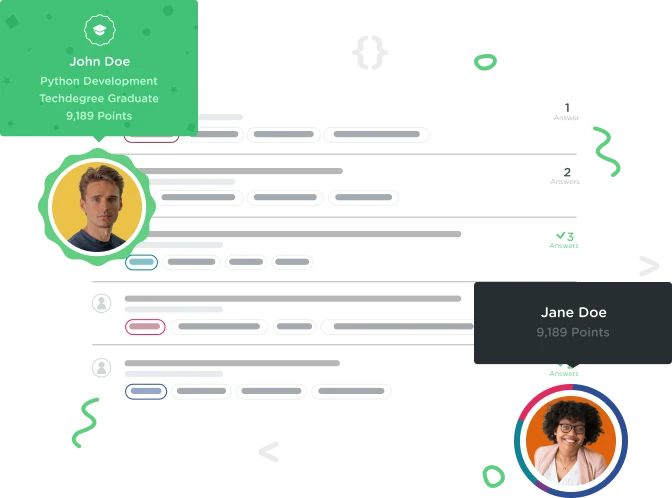

carlos .
9,703 Pointssong lists not rendering to page: here's all the formatted files.
snapshot: https://w.trhou.se/igo4fbnfcn
Error:
Uncaught ReferenceError: song is not defined
at Playlist.add (playlist.js:7)
at app.js:6
app.js
var playlist = new Playlist();
var hereComesTheSun = new Song("Here Comes The Sun", "The Beatles", "2:54");
var walkingOnSunshine = new Song("Walking on Sunshine", "Katrina and the Waves", "3:43");
playlist.add(hereComesTheSun);
playlist.add(walkingOnSunshine);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement = function(list){
list.innerHTML = "";
for(var i = 0; i < this.songs.length; i++) {
list.innerHTML += this.songs[i].toHTML();
}
};
playlist.js
function Playlist() {
this.songs= [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function() {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex ++;
if(this.nowPlayingIndex === this.songs.length){
this.nowPlayingIndex = 0;
}
// this.play();
};
Playlist.prototype.renderInElement = function() {
};
song.js
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying){
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString +- '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
index.html
<!DOCTYPE html>
<html>
<head>
<title>Treetunes</title>
<link rel="stylesheet" href="style.css"/>
</head>
<body>
<div>
<h1>Treetunes</h1>
<ol id="playlist">
</ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
</div>
<script src="playlist.js"></script>
<script src="song.js"></script>
<script src="app.js"></script>
</body>
</html>
style.css
@import url(http://fonts.googleapis.com/css?family=Varela+Round);
@import url(http://necolas.github.io/normalize.css/3.0.2/normalize.css);
body {
background: #ECEEEF;
text-align: center;
margin: 100px auto;
width: 80%;
padding: 0 40px;
font: 14px normal 'Varela Round', Helvetica, serif;
}
h1 {
font-size: 20px;
color:white;
}
div {
background: #c25975;
border-radius: 4px;
border: 1px solid #D5DDE4;
margin: -40px 0 0;
}
ol {
list-style-type: none;
padding: 0;
background:white;
margin: 0 0;
width: 100%;
display: inline-block;
}
li {
color: #2C3238;
width: 96%;
padding: 15px 2%;
margin: 0;
text-align: left;
border-top: 1px solid #D5DDE4;
}
li span {
float: right;
color: #777B7E;
}
li.current {
background: #ffd7d8;
}
button {
margin:10px;
padding:10px 35px;
border: none;
border-radius: 5px;
background: white;
}
4 Answers

Dan Oswalt
23,438 PointsJust taking a glance, your function definition doesn't take any parameters, it should read Playlist.prototype.add = function(song)
, whereas at the moment it's empty. The error is telling you that in this function scope, 'song' isn't recognized.

Dan Oswalt
23,438 Pointsplaylist.renderInElement()
doesn't get called anywhere that I can tell. If I'm reading this correctly, it looks like what you'd need to do would be to call playlist.renderInElement(playlistElement)
at the end of app.js?

carlos .
9,703 Pointshmmm. I do have that on line 10 of app.js
playlist.renderInElement(playlistElement);
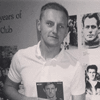
Dom Farnham
19,421 PointsTry adding song as a parameter when defining your Playlist.add method.
Playlist.prototype.add = function (song) {
this.songs.push(song);
};

Dan Oswalt
23,438 PointsOh, it's not there in the app.js snippet that you have up, it sounds like you may be running a different version of app.js locally than what is posted at this point.
carlos .
9,703 Pointscarlos .
9,703 PointsYeah I tried that before. When I pass in
song
an error is no longer generated. But still don't get rendered html. So there still must be something else missing.