Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial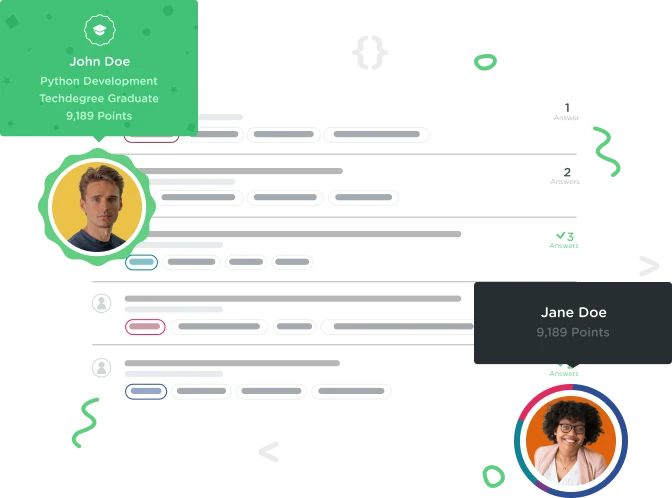

Kim Still
7,355 PointsSongs not showing up - no console errors
My songs are not showing up and I have no idea why. I've looked over my code again and again with no luck. I'm not getting a console error and I even pasted it into JSHint and got no errors. Any ideas?
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if (this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function() {
this.songs.push();
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML = "";
for(var i = 0; i < this.songs.length; i++) {
list.innerHTML += this.songs[i].toHTML();
}
};
var playlist = new Playlist();
var hereComesTheSun = new Song("Here Comes the Sun", "The Beatles", "2:54");
var walkingOnSunshine = new Song("Walking on Sunshine", "Katrina and the Waves", "3:43");
playlist.add(hereComesTheSun);
playlist.add(walkingOnSunshine);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
2 Answers

Unsubscribed User
8,841 PointsHi, The song is added to the playlist by calling the add function. But it is not referenced and used in the add function.
playlist.add(hereComesTheSun);
playlist.add(walkingOnSunshine);
can you modify the add function to add to the songs array.
Playlist.prototype.add = function() {
this.songs.push();
};
To be modified: console.log can be added to validate if the song has been added
Playlist.prototype.add = function(song) {
this.songs.push(song);
console.log(this.songs[0]);
};
Please change and let me know if it works.
Thanks, Aishu

Kim Still
7,355 PointsThat was the problem! Thanks for your help.