Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial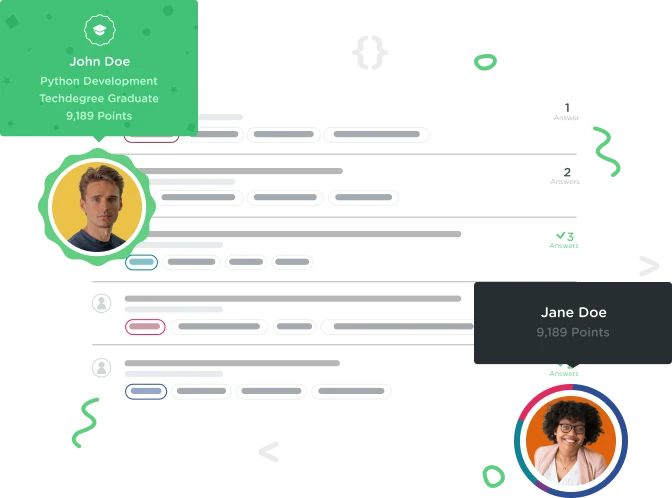
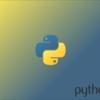
Kevin Faust
15,353 Pointssooo confused
so we can go up to parents objects fine but when we go down to child objects we have to put a parantheses with the child element class? i dont really understand the type casting concept.
and can someone the arrays part from this video?
im only familar with String [] but this one is with "Object" in front. and then why do we have (Treet) below it when we want to call "someStuff".
Object[] someStuff = {treet, "a string"};
((Treet) someStuff[0].getDescription();
and i dont get the part where we started using instanceOf.
so to sum it up i dont get what we accomplished or did in this video. i already watched it several times with 0.75 speed but still all foreign..i need like a plain english beginner explanation of this stuff
2 Answers
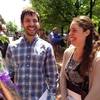
Mike D'Aguillo
5,475 PointsI haven't watched this video, but I think I understand your question and will try to answer it as best as I can.
In Java, every type of class you make is an extension of the type "Object". Think of an object as the most general/abstract form of something. An object has some super basic functions and properties, and all classes in Java implement these properties/functions in some way (the .ToString() function for example is available for all types of classes).
In the first line, you're instantiating an array of "Objects". An array is just a type of collection, and you can have an array of any type (like you said you were familiar with string arrays. That's just an array composed of only strings). All the program knows at this point, is this array is composed of the most general things you could tell it. In this example, you're actually creating the array and adding an object of type "treat", and a string literal. However, because you declared the array as an object array, the compiler only knows that it has 2 "objects" in the array. Java is a strongly typed language, and this is what they mean when they say that. You must tell Java EXACTLY what type of class a variable is before attempting to use a variable. Otherwise, the java compiler will complain. This helps you write cleaner code that won't break during run time.
In the line below, you're attempting to access the first element in the array. Now again, recall that because the array is of type Object, the program only knows that we have some type of object in the first position. It doesn't know any more details. However, WE know we put an object of type Treet, and would like to access one of the Treet specific functions (the getDescription() function). But you can't just call getDescription on the object that we currently have, because objects don't have that function. We need to tell the program that the object we have is ACTUALLY a Treet object. This is called "casting", and is what you're doing when you preface the someStuff[0] statement with the (Treet) statement. Putting the type of the object we're using in parenthesis before we retrieve the object from the array tells the program that the object that is returned from the first element is a Treet object. Now that we've cast the object, we can then call its function "getDescription". As long as the object is ACTUALLY a treet object, this statement will work. You'll get an exception if you were to declare a string or something, then cast it as a treet object and attempt to call the getDescription function.
Does this make sense? I recommend looking up the difference between strongly type languages such as java, and weakly typed languages such as Python or Javascript. It might help you understand what's going on.
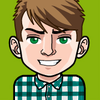
Harry James
14,780 PointsHey Kevin!
I'll try and summarise each bit you don't quite understand below in an easy-to-read manner. Go read through them and if there's anything you're still unsure on, let me know in the comments!
Type casting when downcasting
Let's go back to Craig's example for this. We have an Object and this can be anything (Remember, Everything extends Object) - now let's try and set the Treet variable:
Object obj = new Treet();
Treet another = obj();
Now, we can see how this doesn't work as we're trying to set another
to a Treet but instead, we're giving it an Object. Java has no idea what object this is so we tell it by putting the type cast in.
Your question now is probably "Well, how does upcasting work? Surely that's the same". Well it is sort of, but Java assumes that the object we are setting a variable to is a child of the object on the declaration side. Let's see this with an example:
Collection<String> collection = new TreeMap<>();
So this works because TreeMap is a child of the Collection, and that's what Java is assuming. Everything works fine here :)
Using Object[] in an array
Maybe after reading the above section, this makes sense now but let's still go over this anyway.
When we use Object for an array, we can have any object we want, Strings, integers and other classes, everything's an Object. Now in the example you provided:
Treet treet = new Treet();
Object[] someStuff = {treet, "a string"};
We have a Treet at index 0 and a String at index 1 in our someStuff
array. We can always just take a Treet out and keep it as an object:
Object ourTreetAsObject = someStuff[0];
That works - but what if we want to call something off our Treet. Right now, it's just an Object. Let's say we wanted to call our getDescription() method:
String descriptionFromTreet = ourTreetAsObject.getDescription();
// ERROR!
No that won't work, Java thinks we're calling getDescription() off of the Object - instead, we must cast it to make this work.
Using instanceof
instanceof is a great keyword and allows us to check what our Object actually is. Let's see it in action:
Object stringAsObject = "Hello world";
boolean isStringAsObjectAString = stringAsObject instanceof String;
// >> true - It is actually a String!
boolean isStringAsObjectAString = stringAsObject instanceof Integer;
// >> false - It's not an integer
As you can see, this can come in pretty handy to prevent us getting errors when attempting to downcast! So in plain English, instanceof allows us to check whether a variable is an instance of a class - handy!
Hopefully I've explained these 3 things for you in a way you understand but if you don't quite understand something here or want me to go into something in a bit more detail, drop a Comment and I'll happily help out :)
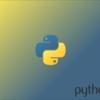
Kevin Faust
15,353 PointsHey harry, thanks. at first i still had no idea what you wrote at all. and thinking how i cant understand even a beginnerish explanation of this,i was contemplating whether or not just to quit this f-ing language. but now i finally understand what your trying to say and the concept behind these 3 points. but like at a extremely basic level.
and i swear the amount of helpful info on java for beginners out online is nothing compared to web dev. now i see why there are so many web devs as a language like java is beyond hard. just to understand the basic concept of this 5 minute video took me probably over 5 hours of searching. everything online and on youtube doesnt give enough explanation and assumes i know everthing already. even here on these treehouse vids.
what really helped me was this link: http://www.cs.utexas.edu/~cannata/cs345/Class%20Notes/14%20Java%20Upcasting%20Downcasting.htm
i tried searching for notes like that on the university of texas site but i have no idea. do you know if there are any sites like that with beginner friendly notes?
thanks for everything
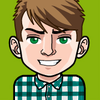
Harry James
14,780 PointsHey Kevin!
I actually learnt everything over here on Treehouse so sadly have no knowledge of any good note sites for you. I'd recommend you put up a new post on the Java section to see if anybody knows any.
Also, I agree with what you say on the documentation. Java's documentation really is not helpful for a beginner and if you're looking to understand something, chances are you'll be trying to understand a lot more than you were originally looking for!
When I put an answer on the Community section, I generally go for a moderate-knowledge answer so that I don't end up writing a huge post. That being said, there are always people who find these posts either too complicated or too basic.
I always try to explain things for anyone, no matter how much knowledge you have of the language. So, if you need something explained in a greater detail, drop a comment with what you need rephrased and I'll help you out :)
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsHey Mike,
at first i had no idea what you were saying. from start to end i was lost. but after searching for hours on youtube and online, i now understand the main concepts/ideas behind what your saying.
thanks for the help
Mike D'Aguillo
5,475 PointsMike D'Aguillo
5,475 PointsHaha I completely understand Kevin. Learning object oriented languages like Java can be massively frustrating at first, but once you start to understand the basics, it will save you from even larger headaches when programming/debugging a very complicated program in the future. My best advice is to just continue with the lessons. Some of it might not make sense right now, but I'm certain you will find yourself weeks/months down the line, coding something completely unrelated and then BAM it just clicks. It really takes hands on experience to understand some of the more dense and abstract concepts with Java.
Mike D'Aguillo
5,475 PointsMike D'Aguillo
5,475 PointsAlso Kevin, I saw that you were looking for additional resources like that utexas link that explained upcasting and downcasting in simple terms. If you're looking to learn Java specifically, and you don't mind learning from a book, I highly highly recommend heads first java. You can find it on amazon here: http://www.amazon.com/Head-First-Java-2nd-Edition/dp/0596009208. The head first series is designed to teach you the basics of a language from scratch, while also teaching the concepts in a more casual manner. Reading computer science resources can be very dense and difficult to take in at first, and I found that this book made programming significantly more approachable (as is the beauty with treehouse as well).
Alternatively, if you're just looking for programming help for beginners, keep posting here, or I would also recommend reddit.com/r/learnprogramming. The community there is very helpful and happy to take beginner questions.
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsThanks Mike for that comment and for your support :) ill also definetely check that book out but dont you think its a bit outdated as the book is from 2005?
also i was re-reading your comment and the video but then this suddenly didnt make any sense to me.
Object ourTreetAsObject = someStuff[0];
wouldnt that be the same as
Object ourTreetAsObject = treet;
and it that was the case then why would be able to call the getdescription method?
String descriptionFromTreet = treet.getDescription();
maybe im missing something but thats ^^ what is being outlined in my head and i dont see what is wrong. you probably know.
Mike D'Aguillo
5,475 PointsMike D'Aguillo
5,475 PointsHey Kevin. I actually should've mentioned how old the book was, but I did have that in mind when I recommended it. The thing is, while Java has definitely changed in the past 10 years (for the better), the core of Java remains pretty much the same. And since your questions have to do with the very basics of Java (Object oriented programming, casting etc), the resources in the book are just as relevant. For what it's worth, Java was the second language I ever taught myself (after Python), and I used this book back in 2013 and still found it very relevant. I don't think 2 years will have changed anything.
Regarding your other question, you're almost there in your understanding :) Take a look at this first line:
Object ourTreetAsObject = someStuff[0];
The first word describes the type for the variable "ourTreetAsObject". In this case, we're telling the compiler it's an Object. This makes sense because we're setting the ourTreetAsObject equal to the the first element in the someStuff Object array. If the someStuff array were a String array for example, the line would look like this:
String ourTreetAsString = someStuff[0]; // someStuff is really a string array
Now lets go back to someStuff being an objectArray. What if we wanted to get that string element we placed in it at the second position in the array. We can't just do this:
String thisStringIsTheSecondElement = someStuff[1];
because the someStuff array is an array of objects. What we can do however, is downcast the second element to a string.
String thisStringIsTheSecondElement = (String) someStuff[1];
Now we've successfully retrieved the string from the object array someStuff.
So again, let's look at your code:
Conceptually it makes sense, but you need to the tell the compiler that element you're retrieving from the array is of the type "Treet". But the array is an object array, so you also have to downcast the object you retrieve in that position to a Treet object. After that, the rest of your code looks fine:
Alternatively, you could downcast in the second step, and not the first, in which case, you would be retrieving the object from the object array.
But that's not as easy to read.
In all honesty, you won't frequently be downcasting from an Object, as that is literally the most basic type in Java. I don't think I have any instances in my personal code where I'm creating an object array to store different element types. If I have to hold a collection of strings, I'll create a string array. If I have to hold a collection of Treets, I'll create a Treet array. It's overly confusing to put them both into a single object array and later downcast them to the types they actually are, but that's something that Java let's you do (and it's important to understand how).
Does that make any more sense? It's no the most intuitive at first, but becomes easier to recognize with practice.
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsHey Mike, that was a great explanation. i actually understand what you said. because we're using an object array, everything gets automatically upcasted to the top of the inheritance tree. but by doing this we lose the treets methods and properties until we downcast again. so to use the .getDesciption() method, we just gotta downcast it. everything makes sense now including Craig's example. but i agree that Craig's version was harder to read and the one you wrote was much better.
your last point about keeping different types in a object array makes alot of sense also. because we have to keep downcasting if we want to use them and that gets confusing.
this inheritance+casting stuff is pretty annoying and confusing but at least i understand whats going on now. thanks for your help and support Mike :)