Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial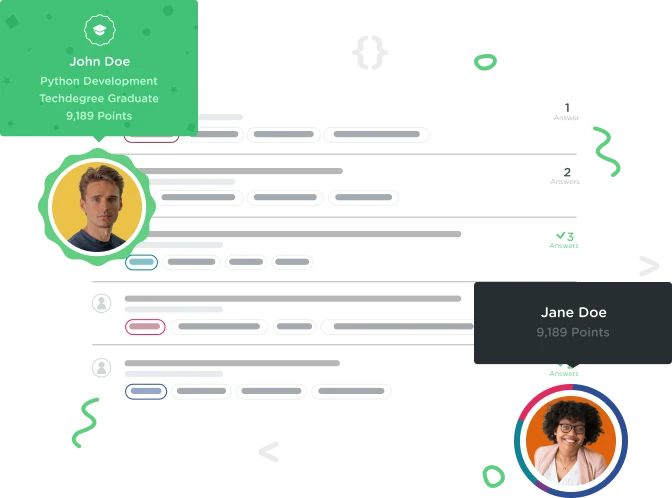

walter fernandez
4,992 Pointssorry, I posted already but I did not put the correct code. I have a problem with this challenge. can someone help me?
Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it. I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(t):
r = t.lower()
a = r.split(",")
for value in a.values():
return value
for i in value():
if value[] == value[]:
return a
for item in r.items():
print(item)
1 Answer
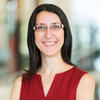
Louise St. Germain
19,424 PointsHi Walter,
There are a number of problems with this code and it will take me way too long to try to explain all of it, but I have a few general suggestions that might help you take another shot at solving this.
First, it's better to use variable names that describe what they are, so it's easier to see what you're doing. The single-letter variables you're using now, like t, a, r
are essentially meaningless (especially to others, and/or to your future self), and it makes debugging much harder than it has to be. Try replacing these variable names with something descriptive, like original_string
instead of t
, lowercase_string
instead of r
, etc.
Second, return
will bump you out of the whole function you're in, so there's no point in writing more code after it. For example,
def word_count(t):
r = t.lower()
a = r.split(",")
for value in a.values():
return value
##############################
# None of the code below this comment will ever run
# because you just used 'return' in the line above.
##############################
for i in value():
if value[] == value[]:
return a
for item in r.items():
print(item)
My last suggestion for complex coding challenges like this one would be to start with writing pseudo-code. By that I mean write out the algorithm using words first, before trying to write it in Python (or other coding language). For example, the first three lines of this program might look like:
# Set up a function called word_count that takes in a string.
# Convert the string to lower case.
# Split the string into all its individual words.
# ... etc...
Once you have all the steps written in regular words and it looks like it should work, you can then convert the steps to Python. So the same three lines above might look like:
# Set up a function called word_count that takes in a string.
def word_count(original_string):
# Convert the string to lower case.
lowercase_string = original_string.lower()
# Split the string into all its individual words.
list_of_words = lowercase_string.split()
# ... etc...
(You can take out all the comments as you go along but I have left them here so you can see how they line up.)
Use Google, help docs, Treehouse videos, etc. as necessary. They can all be very helpful! In this case I would especially suggest re-watching the Treehouse videos related to what you're having trouble with, and take notes (especially of syntax) so you can look at them later.
Good luck! You can do this! :-)