Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial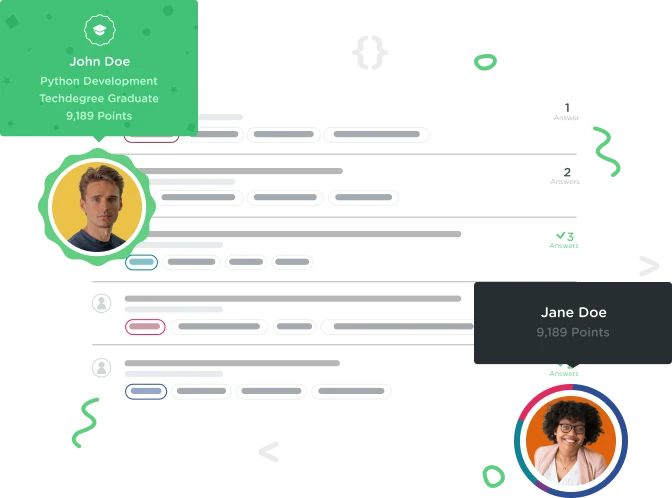

JONATHAN PACHA
12,039 PointsSort an array and then listing the items in a descending order using a for or while loop
I am trying to do just that with this array
var temperatures = [100,90,99,80,70,65,30,10];
my only guess is this
for ( var i = [0]; i <= [7]; i += [1]) { console.log(i); }
var temperatures = [100,90,99,80,70,65,30,10];
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>

Damien Watson
27,419 PointsHey Maxwell, you are spot on. Copy this into an answer so Jonathan can mark as the correct answer, I was about to press 'post answer' when I saw your comment. :)
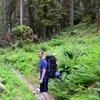
Maxwell Carlson
7,265 PointsThanks Damien, I didn't realize that there was a separate instance for entering in an answer, that should help haha.

Damien Watson
27,419 PointsNo problems. :)
2 Answers
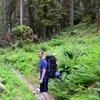
Maxwell Carlson
7,265 PointsIt will look something like this:
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 0; i < temperatures.length; i++) { console.log(temperatures[i]); }
You set i equal to zero in the loop. Tell the loop to stop when it's longer than the length of the array (here arrary is 0,1,2,3,4,5,6,7 - so it stops when it is shorter than 8). i++ just increases i by one each time through the loop.
Next you console.log() the i value of the array temperatures. So first time through the loop i = 0, it will log the 0 position of the array on the same run through the loop with console.log(temperatures[i]); remember, things in the array can be accessed by array[x]. In this case temperatures[0] for the first iteration through the loop. Hope that helps and let me know if you have any more questions!

JONATHAN PACHA
12,039 Pointsso just to get this straight i++ sorts the array to decrease the value?
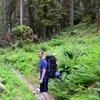
Maxwell Carlson
7,265 Pointsall i++ does is increase the i variable, in this case the counter, by one for each time the loop runs. It's the same as saying:
i + = 1
The
console.log(temperatures[i])
could be better said to sort the array from temperatures [0] - temperatures[7] (end of array). But every part of the code I linked above in my answer plays a role in it. If you don't understand what a particular piece of code does feel free to ask.

Damien Watson
27,419 PointsThere might be a misunderstanding here, none of the code on this page actually sorts the array. The array is already in highest to lowest, so all that is happening is writing out all values from the first to the last value (they just happen to be in 'sorted' order already).

JONATHAN PACHA
12,039 Pointshow can they be sorted from highest to lowest already when [90,99,80] are what are listed
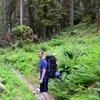
Maxwell Carlson
7,265 PointsOh, I understand what you're saying. Are you doing this for a challenge? I remember a similar challenge that asked you to do what you're asking. I don't think they expected you to sort the array, since it was already sorted. If you're trying to actually sort it then that's a totally different ball game. Sorry for the misunderstanding. In order to sort it if it was not in order, I would use .sort()
var temperatures = [100,90,99,80,70,65,30,10];
function myFunction() {
temperatures.sort(function(a, b){return a-b})
}
Some good info on the subject is located here - http://www.w3schools.com/jsref/jsref_sort.asp
That's going beyond what the challenge is asking though.

Damien Watson
27,419 PointsJonathan, you are correct, I was a little tired last night when I read the numbers. The challenge doesn't actually require you to sort, but again Maxwell is on the money. :)
Maxwell Carlson
7,265 PointsMaxwell Carlson
7,265 PointsIt will look something like this:
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 0; i < temperatures.length; i++) { console.log(temperatures[i]); } you set i equal to zero in the loop. Tell the loop to stop when it's longer than the length of the array (here arrary is 0,1,2,3,4,5,6,7 - so it stops when it is shorter than 8). i++ just increases i by one each time through the loop.
Next you console.log() the i value of the array temperatures. So first time through the loop i = 0, it will log the 0 position of the array on the same run through the loop with console.log(temperatures[i]); remember things in the array can be accessed by array[x] in this case temperatures[0] for the first iteration through the loop. Hope that helps and let me know if you have any more questions!