Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial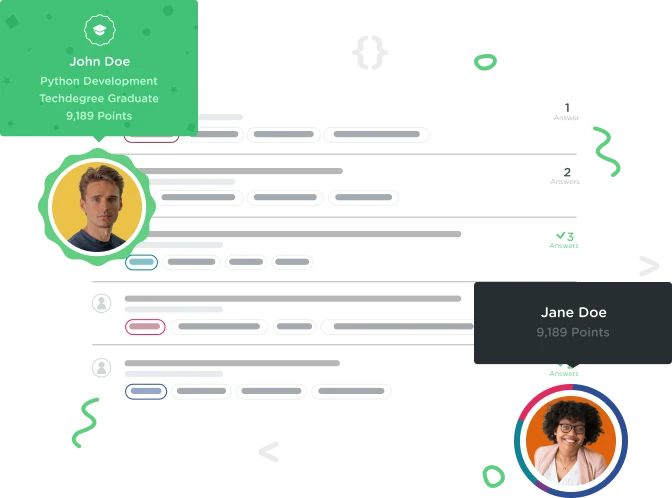
Unsubscribed User
866 PointsSort Array in Javascript
I'm trying to sort an array without using the method .sort(). But my code is not sorting the array.
function sorter(array){ var swap; var count = 0; var swapCount = 0;
do{
for (var i = 1, swapCount = 0; i < array.length; i++){
if(array[count]>array[i]){
swap = array[count];
array[count] = array[i];
array[i] = swap;
swapCount +=1;
}
count++;
}
}while(swapCount>0 );
return array;
}
sorter([4,10,2,9]);
/// shoots back [4, 10, 9, 2] - what am I doing wrong?
Unsubscribed User
866 PointsHey Jesse,
I'm trying to prep for an interview, so I'm taking on algorithm challenges...
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Jen,
I think your problem is that the count variable is never re-initialized to zero when beginning another iteration for the for loop. It just keeps going up. Seems like you would eventually be accessing the array out of bounds.
Also, you don't really need the count variable but I think it's always suppose to be 1 less than i
. Might as well use i - 1
. I think that's more readable since what you're doing is comparing one element with the one that comes before it. At least in my mind, using i
and i - 1
makes that relationship clearer.
I updated your function to this:
function sorter(array){
var swap;
var swapCount = 0;
do{
for (var i = 1, swapCount = 0; i < array.length; i++){
if(array[i - 1]>array[i]){
swap = array[i - 1];
array[i - 1] = array[i];
array[i] = swap;
swapCount +=1;
}
}
}while(swapCount>0 );
return array;
}
I'm a bit rusty with sorting algorithms. Were you trying to implement bubble sort here?

Jason Anello
Courses Plus Student 94,610 PointsIt's been brought up before but there really needs to be a notification that someone else is writing an answer.
Unsubscribed User
866 Points Hey Jason,
Thanks for your help. I can't believe it was as simple as getting rid of the count variable and simply using (i-1). I can't tell you how long I kept looking at my code thinking "what the heck is wrong with this thing?!" Anyway, while there was an answer prior to yours, I'm glad you got to answer because ur answer directly addressed the issue I was having. Thanks again !
ps. Not sure what a bubble sort is, I could have potentially been doing that...
Jen

Jason Anello
Courses Plus Student 94,610 PointsYou're welcome.
There are different types of sorting algorithms - bubble sort, selection sort, merge sort, quicksort, etc... They all have varying levels of efficiency in how quickly they sort the items. I don't know what the javascript sort function uses internally.
You may want to review these if you are prepping for an interview where sorting algorithms are relevant. They may ask for them by name.
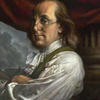
Jeff Busch
19,287 PointsHi Jen,
Took me a little while but here you go.
Jeff
function sorter(array)
{
var count = array.length - 1,
swap,
j,
i;
for (j = 0; j < count; j++)
{
for (i = 0; i < count; i++)
{
if (array[i] > array[i + 1])
{
swap = array[i + 1];
array[i + 1] = array[i];
array[i] = swap;
}
}
}
return array;
}
console.log(sorter([4, 10, 2, 9, 11, 3, 13, 5]));
Jesse Richard
1,899 PointsJesse Richard
1,899 PointsJust curious, why?