Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial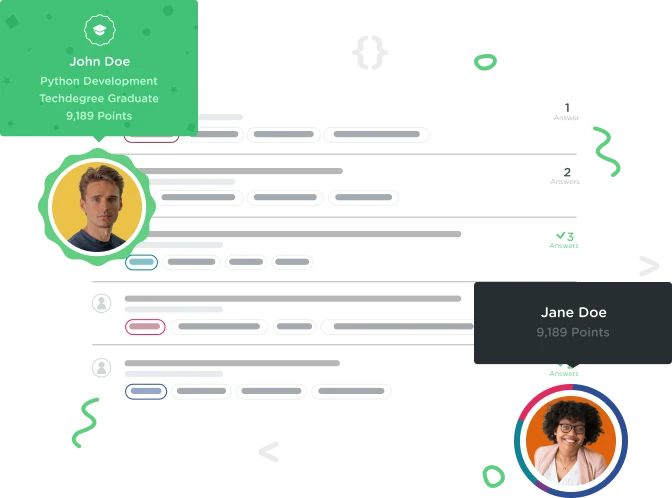
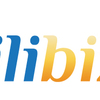
Mathieu Martinot Dubary
4,978 PointsSort the array in length order
Hello,
I'm doing a code challenge but I can't find the answer ... :( Can you help me ?
"Challenge task 2 of 2 Sort the 'saying2' array, on about line 19, so that the words are listed in length order. The shortest first. Use the 'length' property on the strings in the sort function."
My code is that
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function (a, b) {
return saying2.length
a > b;
});
</script>
I really don't understand why it's not sorted by length, can you tell me where I'm wrong ?
Thank you
5 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Mathieu,
You have to subtract the lengths of each string.
saying2.sort(function(a, b) {
return a.length - b.length;
}
The compare function needs to return a positive, negative, or zero value to let the sort method know what order a
and b
should be in.
There's more info on this mdn page: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
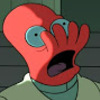
Amanda Kuek
4,109 PointsHi Mathieu,
How's this?
saying2.sort(function(a,b) {
return a.length<b.length;
});
Cheers, Amanda.
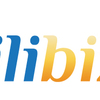
Mathieu Martinot Dubary
4,978 PointsHello Amanda,
Thank you for your answer. Unfortunatly it's not working :/ I'll keep looking to figure this out
Thank you
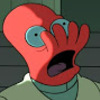
Amanda Kuek
4,109 PointsSorry, the one I said previously sorts in descending (longest first). Try reversing?
saying2.sort(function(a,b) {
return b.length<a.length;
});

Jason Anello
Courses Plus Student 94,610 PointsHi Amanda,
It's risky returning a boolean from the compare function and it's not always guaranteed to work.
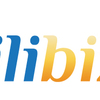
Mathieu Martinot Dubary
4,978 PointsAmanda / Jason, Thank you for your answers :)
Harry James
14,780 PointsHarry James
14,780 PointsWas looking for a site that would better explain sorting. Thanks for that!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Harry,
You're welcome. That mdn page contains a good description of the compare function and shows the longer general form of it.
This particular compare function written in the general form would look like:
This general form and the shorter expression I gave for an answer are functionally equivalent as far as the sort method is concerned. It will not be able to tell the difference between the two versions. It may be useful to you to plug in various numbers for
a
andb
into both versions to see how they're functionally equivalent as it relates to the sort method.You can always fall back on this general form if you're unable to come up with a shorter expression that does the same thing.
Harry James
14,780 PointsHarry James
14,780 PointsI understand how it works partially as in If function(a,b) is less than 0, sort a to a lower value than b, i.e. a comes first. If function(a,b) returns 0, leave a and b unchanged with respect to each other, but sorted with respect to all different elements. If function(a,b) is greater than 0, sort b to a lower index than a, i.e b comes first.
I just didn't understand why taking the two numbers away from each other decides whether a goes first or b goes first. How would a value of -1 for example, decide that a comes first. Or how does a returned value of 1 cause b to go first?
I may be going a bit off-topic here but, I'd appreciate if anybody knew the answer just for my personal sake. Haha!
Alexis Gourdol
8,467 PointsAlexis Gourdol
8,467 Pointsthe link helps a lot to understand the compareFunction concept, thanks!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHarry James
Are you asking about how the sort method uses the the return value to do the actual sorting? Or just wondering why it has to return
-1
to indicate thata
should come beforeb
?