Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial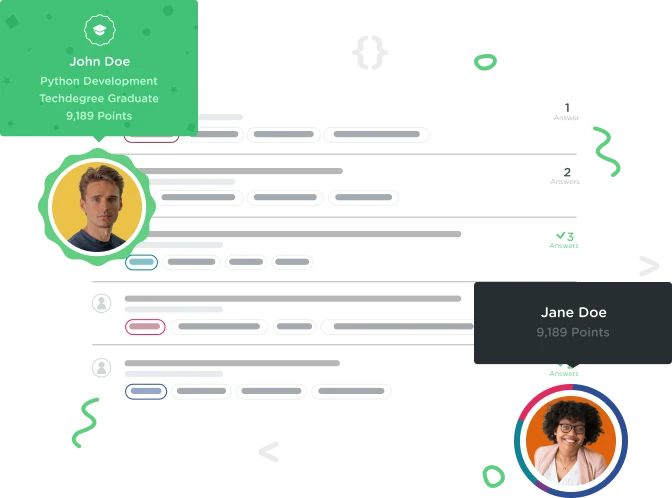
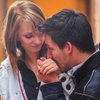
Colton Colcleasure
3,062 PointsSorting an array by length.
I've read questions about this, but they don't seem to clarify it for me. I understand that it works, but not why or how it works.
saying2.sort(function (a, b) { return a.length - b.length;});
What I see is that the function is comparing each item in the array, two at a time. (a,b) Then, it returns the length of a after subtracting the length of b and goes on to compare all other combinations of array items, two at a time. Finally, the sort method sorts our the returned values of all the a.length - b.length.
Is this correct? If so, I think I've got it. If it could be explained better, please give it a shot.
2 Answers
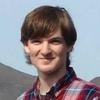
nicktroccoli
3,765 PointsYou definitely have the gist of it. Basically, when you call sort() and pass in a function, JavaScript will call the function you pass in on as many different pairs of items in your array as it needs to figure out the correct ordering. The function you pass in must satisfy the following requirements:
- It has to take 2 arguments - the two items that are being compared at that point in time
-
It has to return either a positive number, negative number, or 0:
- If you return any negative number, that tells sort() that the first argument should come before the second argument in the sorted array.
- If you return 0, that tells sort() that the first argument and the second argument are equal and their order does not matter in the sorted array.
- If you return any positive number, that tells sort() that the first argument should come after the second argument in the sorted array.
So, in the example above, your function correctly takes 2 arguments. To me, this looks like it will sort the array by ascending length. Here's why:
Let's say the sort method is comparing 2 strings: "Hello" and "Hi": a.length = 5 and b.length = 2 so your function returns 3. This indicates to sort() that "Hello" should come after "Hi" because you return a positive number.
Let's say the sort method is comparing 2 strings: "Gorilla" and "Notepad": a.length = 7 and b.length = 7 so your function returns 0. This indicates to sort() that "Gorilla" and "Notepad" are equal (and thus their order doesn't matter) because you return 0.
Lets say the sort method is comparing 2 strings: "Bear" and "Pencil": a.length = 4 and b.length = 6 so your function returns -2. This indicates to sort() that "Bear" should come before "Pencil" because you return a negative number.
It's pretty confusing, I'd agree - but the good news is that for most sorting orders that you want, there's usually a way to make a function that returns either +, 0, or - to correctly sort it. For example, to sort by descending length you could just say:
someArray.sort(function(a, b) {
return b.length - a.length;
});
If you want to sort an array of numbers in ascending order, you could just do:
someArray.sort(function(a, b) {
return a - b;
});
Hope this helps!

benevolentescalator
Courses Plus Student 9,834 PointsFor a visual, I recommend experimenting with adding console.log(a, b); to the function.
For example:
<pre>
someArray.sort(function(a, b) {
console.log(a, b);
return a - b;
});
</pre>

Matt Young
6,348 PointsGreat idea David. I padded out it a bit more to really 'see' what was going on:
var my_array = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
console.log(my_array.toString());
my_array.sort(function(a, b){
console.log(a, b);
console.log(a.length);
console.log(b.length);
console.log(a.length - b.length);
return a.length - b.length;
});
console.log(my_array.toString());
Colton Colcleasure
3,062 PointsColton Colcleasure
3,062 PointsIs it too soon to vote best answer? Haha. Thank you, I believe my head was in the right place, I just couldn't explain it that well. Furthermore; do you have a blog? You seem like you'd be a good coding blogger and someone I would definitely follow.
You are appreciated.
nicktroccoli
3,765 Pointsnicktroccoli
3,765 PointsHahaha thanks! Glad I could help. I don't have a blog, unfortunately, though that isn't a bad idea... haha I'll keep you posted if I make one.
Sam Buck
8,087 PointsSam Buck
8,087 PointsI second the blog idea! That was amazing clear answer. Thank you!
Shea Knox
12,254 PointsShea Knox
12,254 PointsThank you for the explanation. Definitely have a better understanding after reading it.