Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial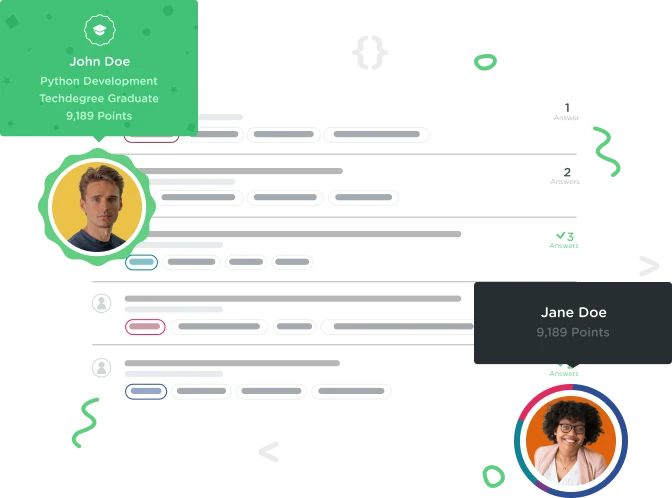

Leo Marco Corpuz
18,975 PointsSorting dates
I’m not sure how to do this part of the challenge. I got lost when watching this part of the lecture.
package com.example;
import java.util.Date;
public class BlogPost implements Comparable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public int compareTo(Object obj){
BlogPost castObj=(BlogPost)obj;
if(equals(castObj)){
return 0;
}
return 1;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
3 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsPart Three
Use compareTo() method to return either a negative or a positive int depending on whether the first object is less or greater than the second.
Hope this clarifies.
public int compareTo(Object other){
BlogPost post =(BlogPost)other;
if(equals(other)){
return 0;
}
return mCreationDate.compareTo(post.getCreationDate());
}
See you around.

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsPart 1 is the easiest part; you just use keywords implements Comparable to make class BlogPost implement Comparable Interface.
public class BlogPost implements Comparable {}
You then generate the compareTo() method with an int as the return type.
public int compareTo(Object other){ return 1; }

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsPart Two
We down cast the Object other to a BlogPost and then check if the current (this) BlogPost object is equal to the other BlogPost Object. If the BlogPost Objects are equal we return 0 - zero.
public int compareTo(Object other){
BlogPost post =(BlogPost)other;
if(equals(other)){
//you could also write the above line as --->>> if(this.equals(other)){}
return 0;
}
return 1;
}
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsAre you on part 2 or 3?