Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial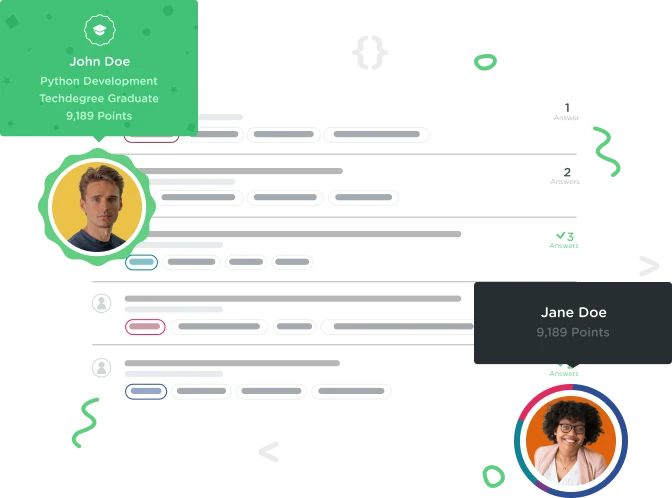
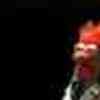
James Andrews
7,245 PointsSound not playing, I don't see what I am doing wrong.
#import "JMAViewController.h"
#import "JMACrystalBall.h"
#import <AudioToolbox/AudioToolbox.h>
@interface JMAViewController ()
@end
@implementation JMAViewController {
SystemSoundID soundEffect;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Get the path to the mp3
NSString *soundPath = [[NSBundle mainBundle] pathForResource:@"crystal_ball" ofType:@"mp3"];
NSURL *soundUrl = [NSURL fileURLWithPath:soundPath];
AudioServicesCreateSystemSoundID(CFBridgingRetain(soundUrl), &soundEffect);
// We are going to do this in main.storyboard, this is just for an example.
// UIImage *backgroundImage = [UIImage imageNamed:@"background"];
// UIImageView *imageView = [[UIImageView alloc] initWithImage:backgroundImage];
// [self.view insertSubview:imageView atIndex:0];
self.crystalBall = [[JMACrystalBall alloc] init];
self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:
[UIImage imageNamed:@"CB00001"],
[UIImage imageNamed:@"CB00003"],
[UIImage imageNamed:@"CB00004"],
[UIImage imageNamed:@"CB00005"],
[UIImage imageNamed:@"CB00006"],
[UIImage imageNamed:@"CB00007"],
[UIImage imageNamed:@"CB00008"],
[UIImage imageNamed:@"CB00009"],
[UIImage imageNamed:@"CB00010"],
[UIImage imageNamed:@"CB00011"],
[UIImage imageNamed:@"CB00012"],
[UIImage imageNamed:@"CB00013"],
[UIImage imageNamed:@"CB00014"],
[UIImage imageNamed:@"CB00015"],
[UIImage imageNamed:@"CB00016"],
[UIImage imageNamed:@"CB00017"],
[UIImage imageNamed:@"CB00018"],
[UIImage imageNamed:@"CB00019"],
[UIImage imageNamed:@"CB00020"],
[UIImage imageNamed:@"CB00021"],
[UIImage imageNamed:@"CB00022"],
[UIImage imageNamed:@"CB00023"],
[UIImage imageNamed:@"CB00024"],
[UIImage imageNamed:@"CB00025"],
[UIImage imageNamed:@"CB00026"],
[UIImage imageNamed:@"CB00027"],
[UIImage imageNamed:@"CB00028"],
[UIImage imageNamed:@"CB00029"],
[UIImage imageNamed:@"CB00030"],
[UIImage imageNamed:@"CB00031"],
[UIImage imageNamed:@"CB00032"],
[UIImage imageNamed:@"CB00033"],
[UIImage imageNamed:@"CB00034"],
[UIImage imageNamed:@"CB00035"],
[UIImage imageNamed:@"CB00036"],
[UIImage imageNamed:@"CB00037"],
[UIImage imageNamed:@"CB00038"],
[UIImage imageNamed:@"CB00039"],
[UIImage imageNamed:@"CB00040"],
[UIImage imageNamed:@"CB00041"],
[UIImage imageNamed:@"CB00042"],
[UIImage imageNamed:@"CB00043"],
[UIImage imageNamed:@"CB00044"],
[UIImage imageNamed:@"CB00045"],
[UIImage imageNamed:@"CB00046"],
[UIImage imageNamed:@"CB00047"],
[UIImage imageNamed:@"CB00048"],
[UIImage imageNamed:@"CB00049"],
[UIImage imageNamed:@"CB00050"],
[UIImage imageNamed:@"CB00051"],
[UIImage imageNamed:@"CB00052"],
[UIImage imageNamed:@"CB00053"],
[UIImage imageNamed:@"CB00054"],
[UIImage imageNamed:@"CB00055"],
[UIImage imageNamed:@"CB00056"],
[UIImage imageNamed:@"CB00057"],
[UIImage imageNamed:@"CB00058"],
[UIImage imageNamed:@"CB00059"],
[UIImage imageNamed:@"CB00060"],nil];
self.backgroundImageView.animationDuration = 1.0f;
self.backgroundImageView.animationRepeatCount = 1;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - IBActions
- (IBAction)predictButton:(id)sender {
[self makePrediction];
}
#pragma mark - motion events
- (void) motionBegan:(UIEventSubtype)motion withEvent:(UIEvent *)event{
NSLog(@"began\n");
[self hideLabelText];
self.predictionLabel.text = nil;
}
- (void) motionEnded:(UIEventSubtype)motion withEvent:(UIEvent *)event{
if(motion == UIEventSubtypeMotionShake){
NSLog(@"ended\n");
[self makePrediction];
}
}
- (void) motionCancelled:(UIEventSubtype)motion withEvent:(UIEvent *)event{
NSLog(@"cancelled\n");
}
#pragma mark - touch events
- (void) touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event {
[self hideLabelText];
NSLog(@"began\n");
}
- (void) touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event {
NSLog(@"ended\n");
[self makePrediction];
}
- (void) touchesCancelled:(NSSet *)touches withEvent:(UIEvent *)event {
NSLog(@"cancelled\n");
}
#pragma mark - Prediction
- (void) makePrediction
{
AudioServicesPlaySystemSound(soundEffect);
[self.backgroundImageView startAnimating];
self.predictionLabel.text = [self.crystalBall randomPrediction];
[UIView animateWithDuration: 7.0 animations: ^{
self.predictionLabel.alpha = 1.0f;
}];
}
- (void) hideLabelText
{
self.predictionLabel.alpha = 0.0f;
}
@end
Does anyone see why my sound isn't playing? I have tried both my code and the project files linked in the project. I get no errors, or warnings, but also no sound. Help?
1 Answer
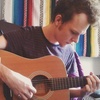
Alex Alexander
5,673 PointsHey James,
I was having the same issue. Turns out it's not a problem with the code, but with some System Preferences on your Mac.
Go into System Preferences > Sound > Sound Effects and make sure "Play user interface sound effects" is checked. If it already is, uncheck and re-check. This solved the issue for me (the box had been unchecked).
Figured this out thanks to a Stack Overflow question: link
James Andrews
7,245 PointsJames Andrews
7,245 PointsWell that blows monkey chunks. I purposefully turned that off to keep the system bell from annoying the crap out of me. Thanks for the info.