Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial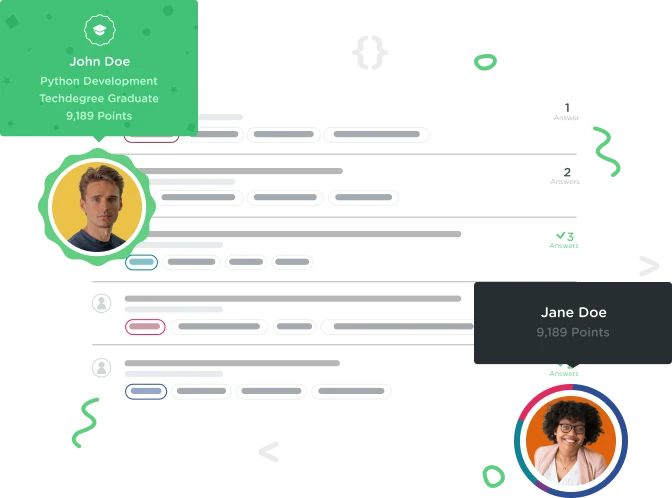

Simon Tanner
5,776 Pointssplitting an array (trying to use reactjs)
Hi, please can you help me with the code below? I am trying to split a sentence in the sentences array into separate words and then put a border around each individual word. I But I can't figure out how to make it work...
var sentences = ["The boy kicked the ball.",
"The girl hit the ball.",
"The dog chased the ball."];
var SentenceBuilder = React.createClass({
render: function() {
const style = {
border: '1px solid'
};
var singleSentence = sentences[Math.floor(Math.random()*sentences.length)];
var splitSentence = singleSentence.split(/(\s+)/);
console.log(splitSentence);
for (var i=0; i<splitSentence.length; i++) {
return (<div style={style}>
{splitSentence[i]}
</div>);
}
}
});
React.render(
<SentenceBuilder />, document.querySelector('.container')
);
Thanks for any suggestions!
4 Answers
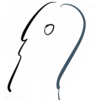
akak
29,445 PointsHi Simon,
I think this is what you're looking for.
var sentences = ["The boy kicked the ball.",
"The girl hit the ball.",
"The dog chased the ball."
];
var SentenceBuilder = React.createClass({
render: function() {
const style = {
border: '1px solid'
};
let output = sentences.map(e => e.split(' ').map(f => <span style= {style}> {f} </span>));
return (
<div>
{output}
</div>
);
}
});
React.render( < SentenceBuilder / > , document.querySelector('.container'));
In react you can't loop the return from render. But you can loop before, and feed the result to the return. Cheers!

Simon Tanner
5,776 PointsHi Jason,
Good point. It is returning just the first word of a sentence in a border. Think maybe something is not what I intend in the for loop or I am using the wrong parameter in the split method... but not too sure. I don't know much more react than you!

Jason Anello
Courses Plus Student 94,610 PointsDid you check what you're getting from your console.log statement? I suspect that your regex for splitting might have a problem.
Since you need to split around the spaces maybe you can keep it simple and just pass in a string consisting of a single space.
var splitSentence = singleSentence.split(" ");
This should give you an array with each word as an element which is what I think you're going for.
I'm not sure about the return statement because that looks like reactjs specific code but as you wrap each word in a div you may need to insert a space in between so that your words still have their original spacing.
Also, I think if each word is in a div then you're going to get one per line on the rendered page since a div is a block level element by default. Maybe this is the intended behavior?
If not you could add display: inline;
to they styling to keep the sentence inline but a better choice from a markup standpoint would be to use a span
instead. Since all you really need here is a generic inline element to be used as a hook for css styling.

Simon Tanner
5,776 PointsThanks a lot guys - yes akak, that has done the trick!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Simon,
I don't know anything about reactjs but it might be helpful to others to say what result you're getting from this.