Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial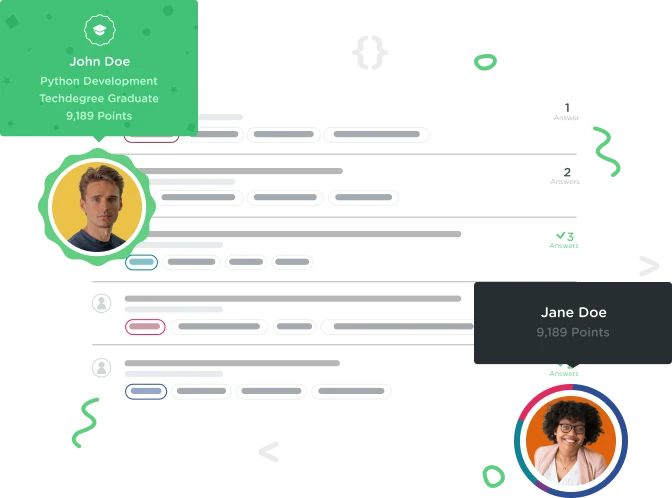
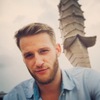
Sean Knowles
Courses Plus Student 265 PointsSpoiler Alert, Challenge 1 solved, help on the 2nd challenge?! Add commas to the input? Move to next item when , input?
Hi all,
Ok so in the last 40 seconds of the video he lays out two challenges. The first one I have completed, which was to add a comma to the end of each item of the list when it prints at the end. Which I have done by concatenating a comma to the print in the for loop at the bottom of the code. Code shown below!
===================================================
__author__ = 'seanknowles'
#Shopping list program takes user input, then outputs the list of inputs adding commas
shopping_list = list()
print("what should we pick up at the store?:")
print("Enter 'DONE' to exit and stop adding items to your list!")
while True:
new_item = input("> ")
if new_item == "DONE":
break
if new_item == "done":
break
shopping_list.append(new_item)
print("Added! List has {} items.".format(len(shopping_list)))
continue
print("Here's your new shopping list:")
for item in shopping_list:
print(item + ",")
# challenge 1 completed here with "," concatenation added to each item in for loop.
====================================================
Challenge TWO is to add commas to the user input (and, or) move to next item if comma input detected??? So thats where I am stuck at the moment any help would be greatly appreciated.
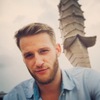
Sean Knowles
Courses Plus Student 265 PointsHey thanks Botond Fekete for your answer, it is much appreciated! Will reference this again soon once I have more knowledge. I don't understand everything you have written lol. Thanks again.
When you mentioned using .strip() method. How would you implement it. Would you use in addition to .split(). Could you give an example in ref to the code above? Thanks :)
2 Answers
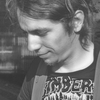
Botond Fekete
15,471 PointsNope, not addition to split, because split() is called on a string and returns an array, strip() only works on strings. So in this case, you'll have to loop through the list you get after calling split() on the input, then you can apply strip() for each item, as they are strings.
user_input_list = input('> ').split(',')
# this is similar to:
# user_input = input('> ')
# user_input_list = user_input.split(',')
# looping through the input list
for item in user_input_list:
# appending each stripped item to the shopping list
shopping_list.append(item.strip())
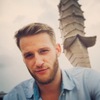
Sean Knowles
Courses Plus Student 265 PointsThanks you!! Great explanation much appreciated, it makes sense now. I went through most of the courses on code.org helped me understand some of the higher concepts. Things are falling into place on how to call functions/methods and read the documentation. Python is so well written compared to other languages. However in the end looks like will have to be learning javascript once I have got my head around this crazy dutch language :)
Botond Fekete
15,471 PointsBotond Fekete
15,471 PointsThe approach you can take in such cases is to get the user input to the variable, maybe you could name it user_input instead of new_item for the sake of consistency, because you don't know how many items will be entered. After you've checked if the input is 'done', you can use the split() method on the input string in the following way in order to get the items separated where there is a comma:
user_list = user_input.split(',')
You can have a look at the documentation of the string operations here (search for string.split): https://docs.python.org/2/library/string.html
If you want to do a perfect job, you maybe want to use the
.strip()
method to get rid of the potencial whitespaces surrounding each item, it the user hit space after each comma.You can then either append the items one by one to the shopping list using a loop, or you can use the
.extend()
method to add the items of user_list to the shopping list's end.Hope it helped :)