Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial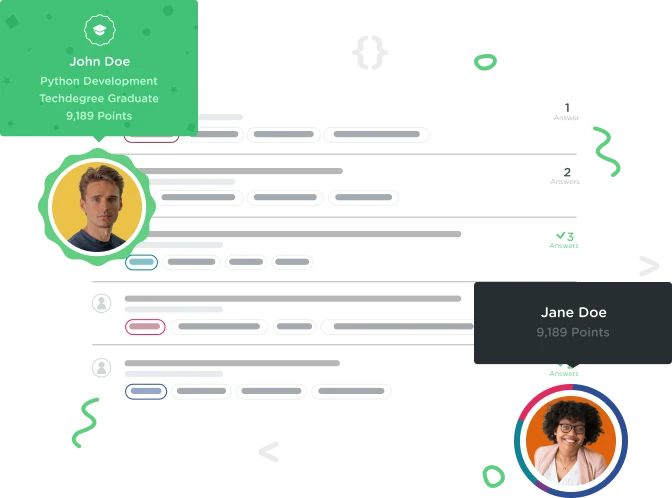

Gareth Gray
3,336 PointsSpotted something unusual...
I passed the challenge with the following code:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> categoryCount = new HashMap<String, Integer>();
for (BlogPost post : getPosts()) {
String category = post.getCategory();
Integer count = categoryCount.getOrDefault(category, 0);
categoryCount.put(category, count + 1);
}
return categoryCount;
}
Yet whenever I tried count++ instead of count + 1, it failed.
Can anyone shed light on why that would be?
Should the code above have failed?
3 Answers
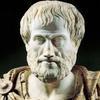
Florian Tönjes
Full Stack JavaScript Techdegree Graduate 50,856 PointsHello Gareth,
with 'count++' you are using the post increment operator. It increments count by one but count still evaluates to its original value in the expression. Use the pre increment operator '++count', which increments count and evaluates to its new value, instead.
Regards, Florian
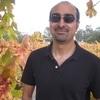
Kourosh Raeen
23,733 PointsIt has to do with the difference between count++
and ++count
. The code will pass if you use the prefix version, ++count
. This is what the documentation says:
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++; and ++result; will both end in result being incremented by one. The only difference is that the prefix version (++result) evaluates to the incremented value, whereas the postfix version (result++) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo, illustrates the prefix/postfix unary increment operator:
class PrePostDemo {
public static void main(String[] args){
int i = 3;
i++;
// prints 4
System.out.println(i);
++i;
// prints 5
System.out.println(i);
// prints 6
System.out.println(++i);
// prints 6
System.out.println(i++);
// prints 7
System.out.println(i);
}
}
I suggest using count++
only if it is statement on its own and if you need to increment or decrement an argument to a method/function or if this operation is part of a larger expression use count + 1
or count - 1
.

Simon Coates
28,694 Pointsclass Main {
public static void main(String[] args) {
int x = 0;
System.out.println(x++);
System.out.println(x);
System.out.println(++x);
System.out.println(x);
}
}
prints 0 1 2 2. When you run increment on it's own line, you don't usually get to see it's behaviour, but used in an expression, it bites you. using ++ after the value means the increment happens after use.
Gareth Gray
3,336 PointsGareth Gray
3,336 PointsThank you Florian!