Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial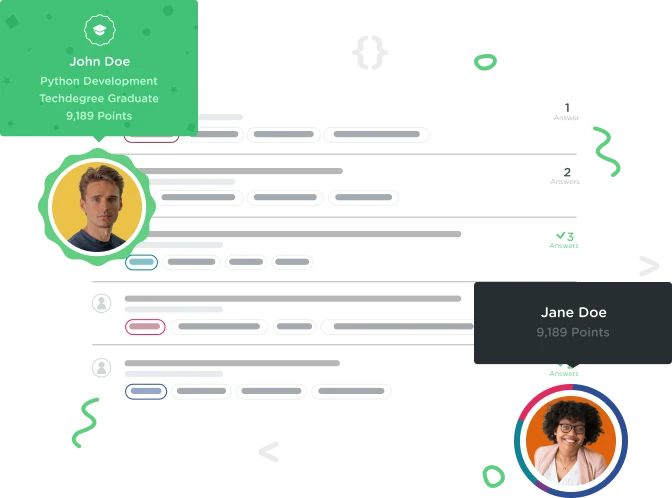

H Yang
2,066 PointsSpread operator, array.map, and initializing nested arrays
I want to create an array of arrays with initialized values, opting to use an array constructor. I'd like to get something that looks like
[[false, false], [false, false], [false, false] ]
Can someone help me understand why my first attempt
let truthTable = new Array(3);
truthTable.map(() => {
return new Array(2).fill(false);
});
doesn't construct the array I want
but using the spread operator like so gets my desired result
let truthTable = [... new Array(3)];
truthTable.map(() => {
return new Array(2).fill(false);
});
This below seems to get the result I expect.
let arr2 = new Array(3).fill(new Array(2).fill(false) )
but it's an array of arrays which all point to the same array:
let arr2 = new Array(3).fill(new Array(2).fill(false) )
arr2[0][0] = true;
// arr2 = [ [true, false], [true, false], [true, false] ]
What am I missing about the spread operator, map, constructing nested arrays?
1 Answer
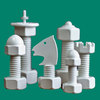
Steven Parker
230,688 PointsIn your first example, the unfilled array is empty, so the map function doesn't find anything to work with. But instead of the spread operator, you could fill the array with a temporary value as you create it (let truthTable = new Array(3).fill(0);
).
But map doesn't change the array directly, you still need to assign the result back to the original array.
Then your other issue is caused by the difference between map (which handles each element individually) and fill which will give each element a reference to the same object.
The most compact way to do what you want might be this:
let truthTable = Array(3).fill(false).map(x => Array(2).fill(x));
H Yang
2,066 PointsH Yang
2,066 PointsThank you for the explanation of how these things worked.