Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial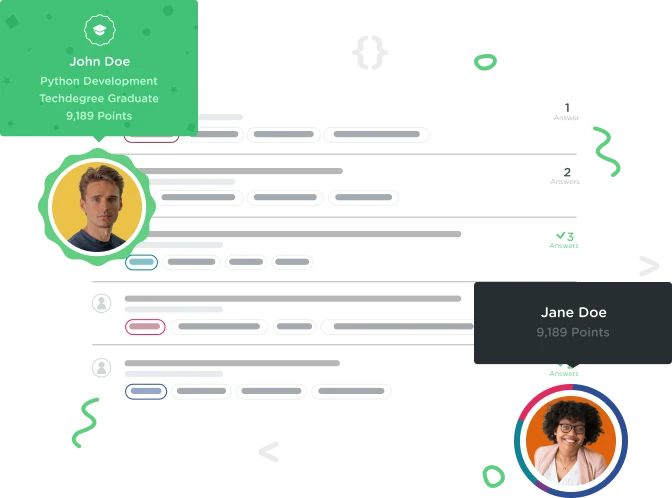
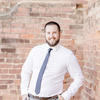
Joshua O'Brian
20,747 PointsSpring basics
Continuing our contact manager application from previous challenges, now your task is to add a Contact POJO. In Contact.java, code a POJO that includes fields for id (int), firstName, lastName, and email. Also include getters and setters for each field. Be sure to name your fields exactly as given in these instructions, and to name your getters and setters using standard Java conventions.
typed this up in IntelliJ setting the getter and setters, also manually typed it both times i get a syntax error but i see they removed the console section of the code challenge for error debugging. Not sure what I am missing here.
package com.teamtreehouse.contactmgr.model
public class Contact {
private int id;
private String firstnName;
private String lastName;
private String email;
public Contact(String email, String lastName, String firstnName, int id) {
this.email = email;
this.lastName = lastName;
this.firstnName = firstnName;
this.id = id;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstnName() {
return firstnName;
}
public void setFirstnName(String firstnName) {
this.firstnName = firstnName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
2 Answers

james white
78,399 PointsI guess the instructor made the assumption that you were going to have IntelliJ setup and generating most of this code, because otherwise this is a LOT of code to produce for a single challenge.
And this code is not all that similar to the code in the zip download for the course.
So to save myself some typing I elected to edit Joshua's code
(instead of using/editing the zip download code) to get this:
package com.teamtreehouse.contactmgr.model;
public class Contact {
private int id;
private String firstName;
private String lastName;
private String email;
public Contact(String email, String lastName, String firstName, int id) {
this.email = email;
this.lastName = lastName;
this.firstnName = firstnName;
this.id = id;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
However, that was till a lot of code to go through --to prove what?
There is so much else going on in this course.
Maybe they (whoever wrote this course) could have just expanded the number of Pojo related questions and not concentrated on requiring on so much typing just to prove you understood generating Pojo code (where most of the getter/setter code is auto-generated by IntelliJ anyway).

Seth Kroger
56,413 PointsOh, how the little things can get you. You're missing the semi-colon at the end of the package statement up top.
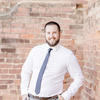
Joshua O'Brian
20,747 Pointsthose semi-colons, also just saw i had an extra n in firstName.