Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial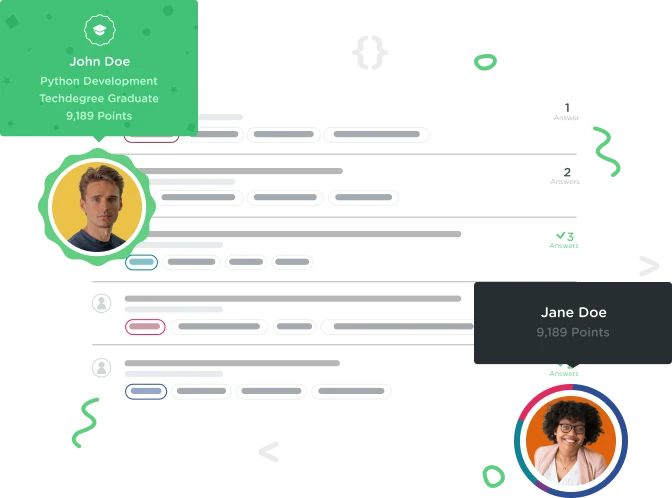

jordan baker
49 PointsSpriteKit - End game after certain amount of time
Would it be possible to end the game after a certain period of time? Say 5 minutes goes by and if the player survived they would get a "you win!" screen.
it seems like you should be able to do
self.lastUpdateTimeInterval = currentTime;
if ( self.totalGameTime > 300 ) {
[self performGameWin];
}
performGameWin would trigger
- (void) performGameWin {
[self.backgroundMusic stop];
SKTransition *reveal = [SKTransition flipHorizontalWithDuration:0.5];
GameWinScene *gameWinScene = [[GameWinScene alloc] initWithSize:self.size];
[self.view presentScene:gameWinScene transition: reveal];
}
I'm still learning and I'm ghetto rigging a lot of my code, so I'm not surprised that this isn't working. Any help would be much appreciated!
EDIT: I can't get the markdown to display the code correctly. Hopefully this makes sense. Sorry about the mess :(
2 Answers
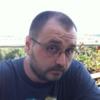
Robert Bojor
Courses Plus Student 29,439 PointsHi Jordan,
You could do something like this...
Define a lastCheckTimeInterval, lastUpdateTimeInterval and totalTimePassed:
@property (nonatomic) NSTimeInterval lastCheckTimeInterval;
@property (nonatomic) NSTimeInterval lastUpdateTimeInterval;
@property (nonatomic) NSTimeInterval totalTimePassed;
Define a method that gets called from the game loop:
- (void)updateWithTimeSinceLastUpdate:(CFTimeInterval)timeSinceLast {
self.lastCheckTimeInterval += timeSinceLast;
if (self.lastCheckTimeInterval > 1) {
self.lastCheckTimeInterval = 0;
self.totalTimePassed++;
}
if (self.totalTimePassed > 30) {
// Call method for ending the game
}
}
And the game loop will look something like this:
- (void)update:(NSTimeInterval)currentTime {
// Handle time delta - If frames drop below 60fps
CFTimeInterval timeSinceLast = currentTime - self.lastUpdateTimeInterval;
self.lastUpdateTimeInterval = currentTime;
if (timeSinceLast > 1) { // more than a second has passed since the last update
timeSinceLast = 1.0 / 60.0;
self.lastUpdateTimeInterval = currentTime;
}
[self updateWithTimeSinceLastUpdate:timeSinceLast];
// the rest of your game loop logic
}
The code in the game loop will account for time delta - dropping below 60fps will normally distort your seconds count if you do it just using currentTime. Once the timeSinceLast is calculated, the updateWithTimeSinceLastUpdate: method is called and that's where you decide when to end the game. I have hardcoded the 30 sec threshold but you can use a variable there if needed.
Hope this helps.
PS I haven't tested it but I believe you will need to initialize self.totalTimePassed to 0 first, so you don't get an error when it will try to increment it.

jordan baker
49 PointsThanks for your answer. I followed your suggestions, but two things go wrong with this solution. The first problem is that for some reason inserting the handle time delta code messes up the game loop and no enemies (spacedogs) appear.
After 10 seconds (i changed it to 10 for quicker testing purposes) the game tries to call the method for ending the game, but it just causes the game to freeze up (no errors, just freezing.)
Any ideas why? I'm not even getting an error so I can't debug it.
EDIT: I solved the freezing up problem, but the no enemies bug still exists. Trying to troubleshoot it without errors is tough. Let me know if you or anyone has any suggestions.
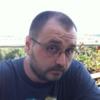
Robert Bojor
Courses Plus Student 29,439 PointsMove the call to line [self updateWithTimeSinceLastUpdate:timeSinceLast]; at the end of the update method. Like I said, I haven't had the time to test the solution - will do and come back.
Stone Preston
42,016 PointsStone Preston
42,016 Pointsformatted your code for you. this animation shows you how to format it