Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial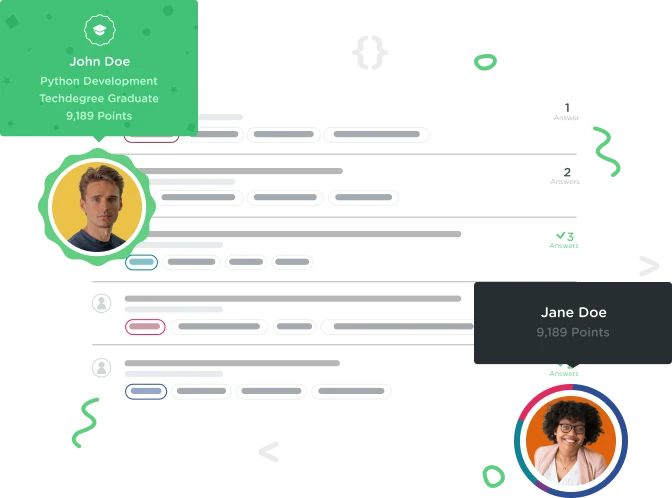

Chi Ian Wong
Courses Plus Student 35 Pointssql database problem
Hi, I am learning to build a very basic sql database app and only include the add data function. I hard coded everything because I just wanted to see if the database can be up and running. There is a error whenever I press my button that calls insert data function. Cause: invocation target exception. My code is below, the main activity and databasehandler. Thanks
Main activity: import android.content.ContentValues; import android.database.sqlite.SQLiteDatabase; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View;
public class MainActivity extends AppCompatActivity { DataBaseHandler dba;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void button(View view){
dba.insert("apple",10,4,5,6);
}
} Databasehandler:
public class DataBaseHandler extends SQLiteOpenHelper { public static final String DATABASE_NAME = "MACRO.DB";
public static final String TABLE_NAME = "MACRO.TB";
public DataBaseHandler(Context context) {
super(context, DATABASE_NAME, null, 1);
SQLiteDatabase db = getWritableDatabase();
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE MACRO_TABLE (_ID INTEGER PRIMARY KEY AUTOINCREMENT, DATE LONG, FOODNAME TEXT, CALORIES INTEGER, PROTEIN INTEGER, CARBOHYDRATE INTEGER, FAT INTEGER)");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS" + TABLE_NAME);
onCreate(db);
}
public void insert(String food, Integer cal, Integer pro, Integer carb, Integer fat){
SQLiteDatabase dba = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("FOODNAME",food);
values.put("CALORIES", cal);
values.put("DATE", System.currentTimeMillis());
values.put("PROTEIN", pro);
values.put("CARBOHYDRATE","carb");
values.put("FAT", "fat");
dba.insert("MACRO_TABLE", null, values);
Log.v("Added Food item", "Yesss!!");
dba.close();
}
}
1 Answer

Daniel Hartin
18,106 PointsHi Chi,
Any chance of posting your logCat. I can see that you aren't creating a new DatabaseHandler object you are trying to call dba.insert() on a null object you need to add dba = new DatabaseHandler(); you also never close the connection inside the Constructor. I understand when this is run the first time you need to run getReadableDatabase() to get the database to install on the device but I would make a separate method for this and definitely close the connection once the database is made.
You also add strings which are hardcoded carb and food when I think you intend to use variables now this wont cause SQLlite to crash (which is annoying at times even on an Integer field) but you should probably avoid it.
And lastly the table is the variable MACRO_TABLE not the string "MACRO_TABLE" which will throw an error and I suspect is the cause.
Hope this helps Daniel