Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial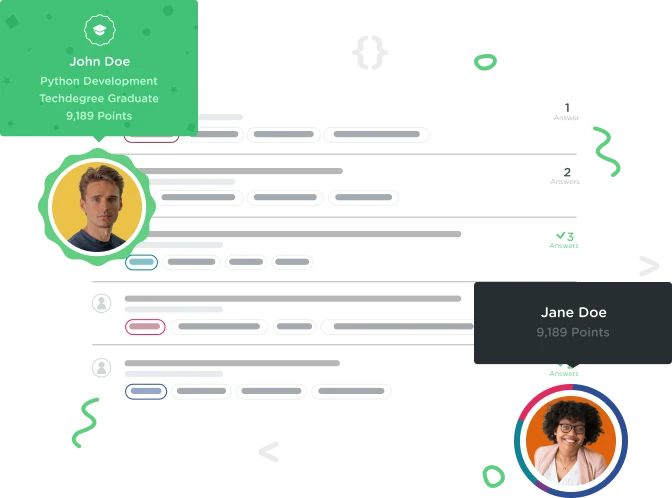
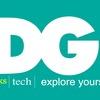
Rahul Singh
1,517 PointsSQLSTATE[23000]: Integrity constraint violation: 19 NOT NULL constraint failed: tasks.date
<?php
function addTasks($project_id,$title,$date,$time){
include 'connection.php';
$sql = "INSERT INTO tasks(project_id,title,date,time) VALUES(?,?,?,?)";
try{
$db = $db->prepare($sql);
$db->bindValue(1,$project_id,PDO::PARAM_INT);
$db->bindValue(2,$title,PDO::PARAM_STR);
$db->bindValue(1,$date,PDO::PARAM_STR);
$db->bindValue(2,$time,PDO::PARAM_INT);
$db->execute();
}catch(Exception $e){
echo $e->getMessage();
return false;
}
return true;
}
?>
Pirvan Marian
14,609 PointsPirvan Marian
14,609 PointsBasically that errors says that the date column from your tasks table can not be NULL, you have set it to be NOT NULL.By the way, you have some wrong things there.
I have no idea how your tables are related but you don't really need to add a record for the project_id column, it's added automatically if you have built your table correctly.
I see that you are including the connection.php file and I suppose that file creates a PDO object, with other words, creates a new connection with the database.If you have similar functions like the one above and you call them in the same file, you have a big problem, each time you create a new connection to database, that's not really something good, not at all.Try to pass the PDO object as a parameter for your methods, take a look at this simple example: