Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial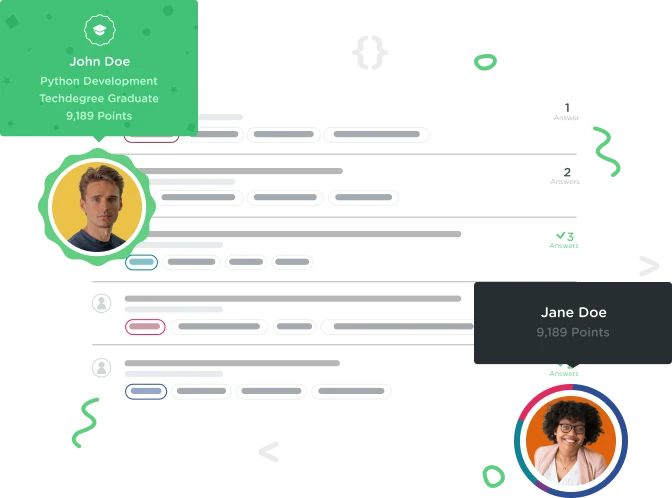

Spencer Heflin
7,389 PointsSquared Code Challenge
Hey guys not really sure where I'm going wrong here. I feel like my mistake is on trying the square the string but might be wrong. Any help would be greatly appreciated.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
int(num ** 2)
except ValueError:
return pow(num, len(num))
5 Answers
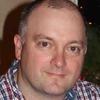
jonlunsford
15,472 PointsSpencer:
The problem is in your try::except block. If the try block succeeds, nothing is returned because your return statement is indented under your exception case. Also, you should store the result in a variable and return the result. You could do something like this.
try:
result = int(num ** 2)
except ValueError:
print("please enter a valid number.")
return result
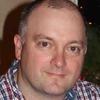
jonlunsford
15,472 PointsYes. The reason you are getting a TypeError when a string is passed is because the int() function cannot convert a string representing characters (i.e.; "tim"). To handle that you need to setup your except case to catch TypeError. So for my example provided previously, the except part would look something like.
except TypeError:
result = num * len(num)

Spencer Heflin
7,389 PointsHopefully this is my last question. I really appreciate your help. Here is what my code looks like now: def squared(num): try: result = int(num * num) except TypeError: result = num * len(num) return result
It keeps saying that squared isn't returning the right answer, but everything looks correct to me. Can you see what I'm doing wrong?

Eric Boggs
1,258 PointsI ended up assigning num to an int before trying to do math against it. Like so:
def squared(num):
try:
num = int(num)
result = num * num

Spencer Heflin
7,389 PointsThanks for your help. I'm still doing something wrong, and I can't figure it out. Any advice? My code now looks like this: def squared(num): try: num = int(num) result = num * num except TypeError: result = num * len(num) return result

Eric Boggs
1,258 PointsTL;DR - Change TypeError back to ValueError
So, I had to take the code and execute it on the command line to play with everything when I was working through the issue. If you modify your code to accept some input and pass it through to your function, you will start to see the errors.
Anyway, your code will pass if you change TypeError back to ValueError. I don't fully understand the difference between the two, but when I ran the code with exception TypeError it failed and told me I had a ValueError instead.
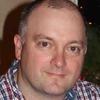
jonlunsford
15,472 PointsYou could also solve it this way:
def squared(num):
try:
result = num * num
except TypeError:
result = num * len(num)
return result
The reason you are getting a ValueError is because you are trying to convert a string to int() and it is failing. If you take away the int() part, then you need to check for TypeError in the except clause. To test this type the following into a Python interpreter:
>>> num = 'hello'
>>> num * num
results in TypeError
>>> num = int(num)
results in a ValueError.

Eric Boggs
1,258 PointsThis solution does work in the command line, but does not seem to be working in the treehouse quiz. However, the int/ValueError solution does work. If I remember correctly, we have only been introduced to ValueError in this course, so maybe they are assuming we do not know TypeError when checking for the solution??

Spencer Heflin
7,389 PointsI finally passed. Thanks a lot for your help guys.
Spencer Heflin
7,389 PointsSpencer Heflin
7,389 PointsThanks for your help. I should've mentioned this in the original question, but if the argument isn't an integer, then I'm supposed to return the argument multiplied by it's length. This is what I've got right now, but I am getting a TypeError. Can you tell what I'm doing wrong? def squared(num): try: result = int(num ** 2) except ValueError: result = pow(num, len(num)) return result