Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial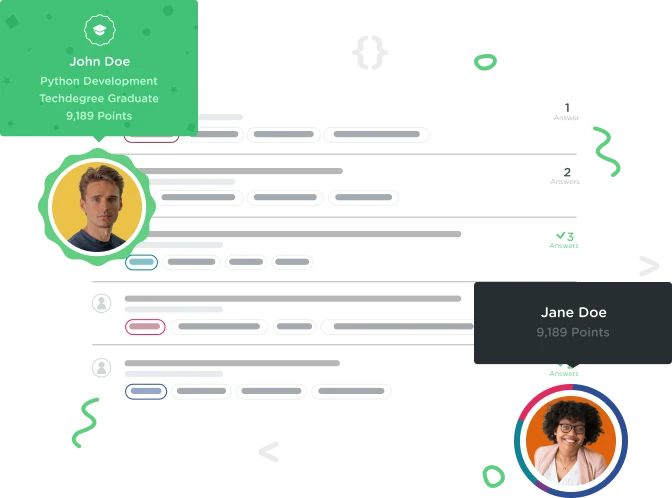

Abdulkadir Kollere
1,723 Points'squared' didn't give the right answer
Please see the attached code. It is giving me the error 'squared' didn't give the right answer. Any help will be appreciated.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
int(num) * int(num)
except ValueError:
return num * len(num)
2 Answers

andren
28,558 PointsThe problem is that in your try statement you convert and multiply the num parameter but you don't actually return it. Meaning that if num can be converted to int then your function doesn't actually return anything, which is why you get an error message about incorrect answers being returned.
Simply adding return to your try block like this:
def squared(num):
try:
return int(num) * int(num)
except ValueError:
return num * len(num)
Will allow you to pass the challenge.
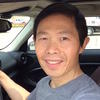
David Lin
35,864 PointsThis worked for me:
def squared(num):
try:
numInt = int(num)
return numInt * numInt
except:
return len(num) * num