Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial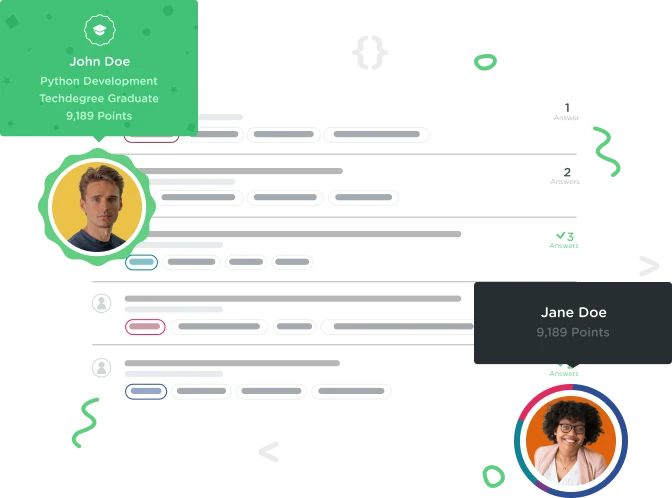
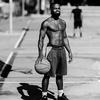
Ryan Felton
1,471 Pointssquared isn't returning the right answer
def squared(num):
try:
if num is int:
return (num*num)
except:
return num*len(num)
[MOD: added ```python formatting -cf]
4 Answers
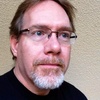
Chris Freeman
Treehouse Moderator 68,457 PointsThe statement if num is int:
will always be False
and not raise an error unless num
is undefined. The is
comparison checks to see if two objects are the same object. Since num
will likely be an int
valueand not the *class*
int`, they will not be the same object.
When using the try
statement, you typically have the expectation that some statement might raise an error that would trigger the except block.
A better solution would be to try an convert the parameter to an int
using
num = int(num)
Use this to replace the if
statement and reindent the return
statement accordingly and it should pass.
Post back (here!) if you need more help. Good luck!!

Ben Reynolds
35,170 Points- In the try block's return statement, cast num to an int. (also, if you want you can shorten it a bit and use "** 2" to square it instead of writing num twice)
- I don't know if the exercise requires it but it's usually best to put the name of the error you want to catch after the Except keyword, such as ValueError.
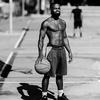
Ryan Felton
1,471 PointsWhat do you mean cast num to an int

Ben Reynolds
35,170 PointsCasting is when you convert a variable from one data type to another. The format looks like this:
datatype(thingtoconvert)
So casting num to an int would look like this:
int(num)
To square something you have to make sure it's a number first, so casting it will either work, or it will throw an exception. If it throws an exception then the except block will run instead of the try block.
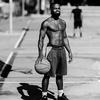
Ryan Felton
1,471 PointsYeah that's what I did and it still didn't work

Ben Reynolds
35,170 PointsYour first return statement has num in there twice, did you cast both of them? If it's still not working can you post the last version of the code you tried
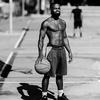
Ryan Felton
1,471 PointsI just posted the last version
Ryan Felton
1,471 PointsRyan Felton
1,471 PointsThank you