Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial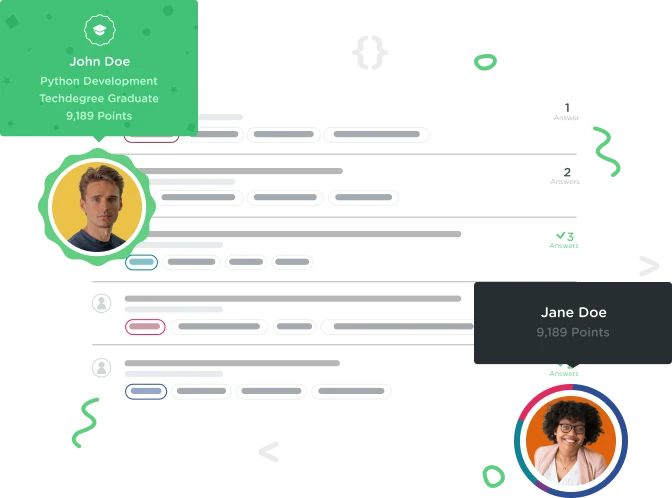

Kishore Kumar
5,451 Pointssquared.py
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
My Code:
def squared(item): try: print(int(item) ** 2) except: print(item * len(item))
squared(2) 4 squared('100') 10000 squared('Python') PythonPythonPythonPythonPythonPython
6 Answers
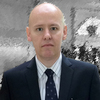
Nathan Tallack
22,159 PointsYou operations are fine, but you need to return your result not print it to the console.
Consider the following code:
def squared(item):
try:
int(item)
except:
return item * len(item)
else:
return int(item) ** 2
Does that make sense?

Sayan Mukherjee
1,033 PointsHey Nathan, but in the challenge it says "You might have to not use the else block, though." It says NOT to use the else block! So now I'm a little confused, whether to use else or not.
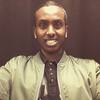
mohammed abdi abdulahi warsame
4,926 Pointsthanks for helping, but does any one know where i can get many code challenges from this topic, cuz i just googled the qustion(feeling my self cheater) and i understood it, but still i want to test my self in this topic. thanks
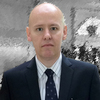
Nathan Tallack
22,159 PointsWhat you can do in this case is work with exceptions from failed typecasting.
Consider something like this. Sorry, this is a Python 3 example, so be sure to be using that.
def is_int(string):
try:
int(string)
except:
print("That does not appear to be a whole number.")
else:
print("That looks like a whole number.")
is_int("A string")
is_int("100")
So what we we are doing here is taking the string passed into this function and trying to typecast it as an int. If that throws an exception we execute the print statement in the except block. If it successfully casts as an int type we execute the print statement in the else block.
If you did not use the else block you would process that second print statement right after that except block, so something that does not type cast would end up with both print statements being executed.
So we are using the else block so that we can have an "either or" not an "either and" flow.

Chris Robinson
4,491 PointsAm I reading this wrong? I did this:
def squared(arg1):
try:
return int(arg1 * arg1)
except:
return arg1 * len(arg1)
It causes the desired results, but doesn't use "else." However I didn't use else because the wording makes it sound like I don't need to:
You might have to not use the else block, though. What is up with the "not" in that sentence?
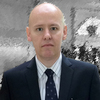
Nathan Tallack
22,159 PointsThe point of the challenge is to teach you the try except else flow control. Sure you can complete the challenge without it, but that is not the point.
The else clause is the point. It runs when there is no exception but before the finally-clause. That is its purpose. Without it the only option to run additional code before finalization would be the clumsy practice of adding the code to the try clause. That is not a good idea because it risks raising exceptions in code that was not intended to be protected by the try code block.
The need to run additional unprotected code prior to finalization doesn't arise very often, so you may not see it much. But it is important to know it is there and learn how to use it.
I think that is the point of the challenge prompting you to use the else clause. :)

Wendy Turner
718 PointsI am wondering why this works. When you say except, wouldn't you need to give it what you want it to except? For example tell it to make the exception when the agr1 isn't an integer. Does it just assume to only do it in the case of a non-integer?
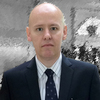
Nathan Tallack
22,159 PointsWendy, this works because it will try to return the multiplication result typecasted as an int. If the multiplication fails or the typecasting fails then it will execute the except block returning arg1 multiplied the number of times of its length.
As mentioned though this is sometimes not the best way because you may not want to risk raising an exception you did not prepare for in the try block. With the example provided by Chris you could mean to test that you can typecast but may actually fail on the multiplication of arg1 with itself. In that case you may execute the except block when you do not mean to.
That is what this lesson is trying to teach the use of the else block. So that you can learn that sometimes you want to separate the code you want to run if the try block passes from the actual try evaluation itself. As mentioned, this need does not arise often, but you need to know how to mitigate it when it does. :)

Wendy Turner
718 PointsNathan, Thanks so much for responding. I am new at this and I am having trouble understanding what you mean by "if typecasting fails." Could you break that down for me? Thanks!
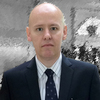
Nathan Tallack
22,159 PointsWendy, sure thing, we are here to help each other learn. :)
Consider if I passed in a number as a string to that funciton. So in my example item could be the string '1'. Before I could do multiply them together using the * operator I would need to conver it to an integer type. That is what the int(item) definition in the try code block does. It will pass because the string '1' can be "typecasted" to the type of int.
But if I passed in the 'one' as the argument, that would not typecast as an int. So the try block would fail and the except block would execute instead, returing 'oneoneone' instead.
So typecasting is when you are getting one type and "casting" it as another. str(1) would typecast as a string '1' because the 1 without being enclosed in the ' would be taken as an integer type. int('1') would typecast as an int 1 because the 1 inside the ' would be taken as a string type.
Sorry for being so verbose. Just want to be sure I can get the message across. I am a better student than teacher. ;)

Wendy Turner
718 PointsThanks so much!! That helps:-)

Lazarus Mbofana
10,941 Pointsdef square(number): return number * number result = square(6) print(result)
Kishore Kumar
5,451 PointsKishore Kumar
5,451 PointsOh, I forgot, in Code Challenge we're not supposed to use print(). Anyway Thanks again Nathan! for this help...
Cody Mills
1,567 PointsCody Mills
1,567 PointsWould you be any worse off by doing it this way?
Nathan Tallack
22,159 PointsNathan Tallack
22,159 PointsYeah, I would do it your way Cody, but the challenge asked for us to use an else clause. That's why I coded it that way.
Weird right?
Ashish Shah
1,468 PointsAshish Shah
1,468 PointsThis does not work!