Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial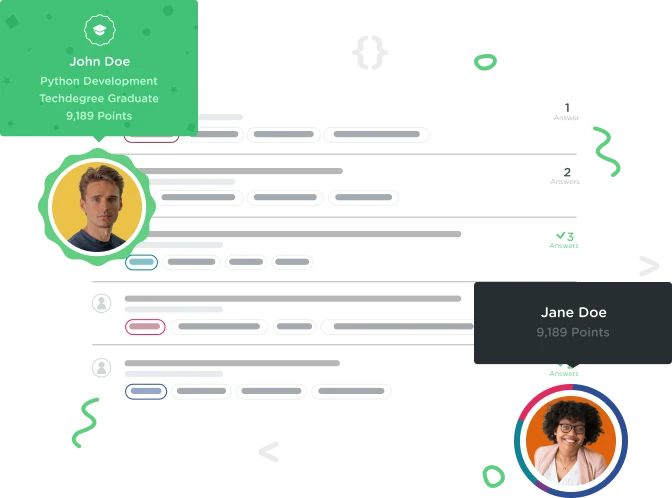
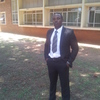
Brett Dube
2,653 PointsStage 2 Harnessing the power of objects
Hie, can somebody help me understand where i am getting it wrong
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive (int laps){
int newCharge =mBarsCount-laps;
if (newCharge<0){
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount=newCharge;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
5 Answers
William Li
Courses Plus Student 26,868 PointsI don't see anything wrong with your code. Are you getting error or sth?
OK, i see the problem now, you have duplicated drive
method.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
delete this block of code, and you're good to go.
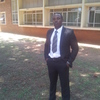
Brett Dube
2,653 Points/GoKart.java:25: error: illegal start of expression public void drive(int laps) { ^ ./GoKart.java:25: error: illegal start of expression public void drive(int laps) { ^ ./GoKart.java:25: error: ';' expected public void drive(int laps) { ^ ./GoKart.java:25: error: ';' expected public void drive(int laps) { ^ ./GoKart.java:30: error: illegal start of expression public void charge() { ^ ./GoKart.java:30: error: illegal start of expression public void charge() { ^ ./GoKart.java:30: error: ';' expected public void charge() { ^ ./GoKart.java:36: error: illegal start of expression public boolean isBatteryEmpty() { ^ ./GoKart.java:36: error: ';' expected public boolean isBatteryEmpty() { ^ ./GoKart.java:40: error: illegal start of expression public boolean isFullyCharged() { ^ ./GoKart.java:40: error: ';' expected public boolean isFullyCharged() { ^ ./GoKart.java:44: error: reached end of file while parsing } ^ JavaTester.java:54: error: cannot find symbol if (!kart.isBatteryEmpty()) { ^ symbol: method isBatteryEmpty() location: variable kart of type GoKart ./GoKart.java:25: error: variable laps is already defined in method drive(int) public void drive(int laps) { ^ ./GoKart.java:31: error: cannot find symbol while (!isFullyCharged()) { ^ symbol: method isFullyCharged() location: class GoKart ./GoKart.java:37: error: cannot return a value from method whose result type is void return mBarsCount == 0; ^ ./GoKart.java:41: error: cannot return a value from method whose result type is void return mBarsCount == MAX_BARS; ^ 17 errors
William Li
Courses Plus Student 26,868 PointsI don't know what you have done wrong then.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive (int laps){
int newCharge =mBarsCount-laps;
if (newCharge<0){
throw new IllegalArgumentException ("Not enough battery remains");
}
mBarsCount=newCharge;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
Copy this code over and replace the code template provided by the Challenge, you'll pass. This code is your original code with the duplicated method removed.
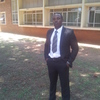
Brett Dube
2,653 Pointsthat is what the java editor is saying but at first it was only saying "variable drive is already defined" so now i think i messed up something else which i i am failing to figure out now
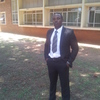
Brett Dube
2,653 Pointsokay william, let me try to start afresh, i think i have messed up something else, thank you so much. will keep you posted