Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial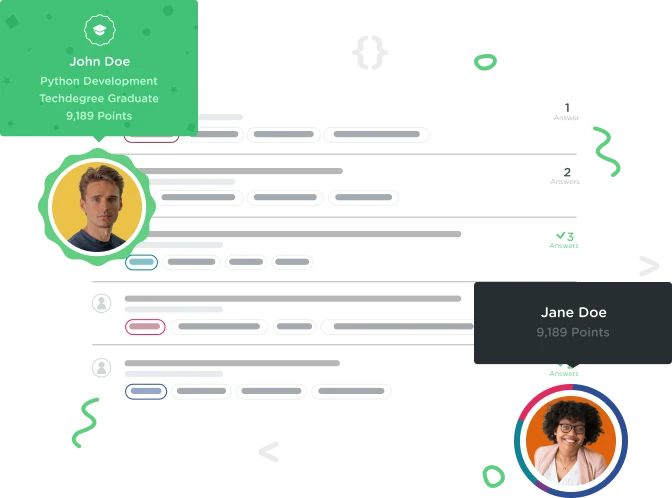

Matthew H
6,627 PointsStage 4 AJAX/API Quiz - using 'text()'
Can someone tell me where I went wrong in the last line of code? The quiz is saying that I haven't chained the .text() function to the jQuery, but I did, right?
$(document).ready(function() { var weatherAPI = 'http://api.openweathermap.org/data/2.5/weather'; var data = { q : "Portland,OR", units : "metric" }; function showWeather(weatherReport) { $('#temperature').show(); }; $('#main').text(weatherReport.main.temp); });
$(document).ready(function() {
var weatherAPI = 'http://api.openweathermap.org/data/2.5/weather';
var data = {
q : "Portland,OR",
units : "metric"
};
function showWeather(weatherReport) {
$('#temperature').show();
};
$('#main').text(weatherReport.main.temp);
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>What's the Weather Like?</title>
<script src="jquery.js"></script>
<script src="weather.js"></script>
</head>
<body>
<div id="main">
<h1>Current temperature: <span id="temperature"></span>°</h1>
</div>
</body>
</html>
5 Answers
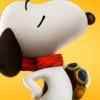
Salman Akram
Courses Plus Student 40,065 PointsHi Matthew,
Actually you don't need to put .show(), that should be replaced by .text(), you need to add JSON function something like below.
Errors here
function showWeather(weatherReport) {
$('#temperature').show();
};
$('#main').text(weatherReport.main.temp);
});
Question is Finally, to insert the temperature into the div, use jQuery's text() method. You'll need to pass the temperature into this method. The Open Weather Map API returns an object with the property main; that property holds another object with the property "temp". To access the temperature, use dot syntax, like this: weatherReport.main.temp
$(document).ready(function() {
var weatherAPI = 'http://api.openweathermap.org/data/2.5/weather';
var data = {
q : "Portland,OR",
units : "metric"
};
function showWeather(weatherReport) {
$('#temperature').text(weatherReport.main.temp);
}
$.getJSON(weatherAPI, data, showWeather);
});
Hope that helps. :)
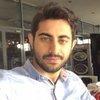
HIDAYATULLAH ARGHANDABI
21,058 PointsLet me write the full form . My friend has explained it you have make mistake in closing the brackets
$(document).ready(function() {
var weatherAPI = 'http://api.openweathermap.org/data/2.5/weather';
var data = {
q : "Portland,OR",
units : "metric"
};
function showWeather(weatherReport) {
$('#temperature').text(weatherReport.main.temp);
}
$.getJSON(weatherAPI,data,showWeather);
});

Matthew H
6,627 PointsAh! I had mixed up the $.getJSON and the txt() questions. Thank you!

Silver Kuklase
12,196 Pointsah right, I was a bit confused why do we need the .text() method there, but then realized it takes the text from the "api" not from our index.html
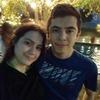
Alexis Ruiz
9,237 PointsWe were taught that the text method returned the text in the chained html element. We weren't told that we could use it to insert text into an html element.