Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial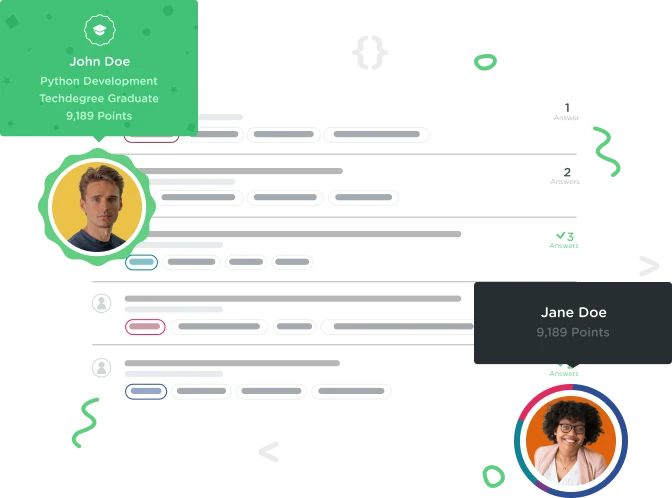
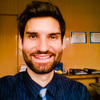
Alaric Wimer
7,940 PointsStage 4 Optional Challenge: How can I display different photos each time the user enters the same search term?
I'm wondering if someone could point me in the right direction. For example, if a user enters "cat", hits submit, and enters "cat" again, the same images appear. I'd like to get the page to display new photos each time. I think the solution lies in using $.each() method but making "i" a random number. Just not sure how to do that. Any help would be greatly appreciated.
Here's my code:
$(document).ready(function() {
$('form').submit(function (evt) {
evt.preventDefault();
var $searchField = $("#search");
// disable and change the text of submit button after clicking on it
var $submitButton = $('#submit');
$searchField.prop("disabled", true);
$submitButton.prop("disabled", true).val("searching...");
// the AJAX part
var flickerAPI = "http://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?";
var searchTerm = $('#search').val();
var flickrOptions = {
tags: searchTerm,
format: "json"
}; // end flickrOptions
function displayPhotos(data) {
// print a message if no photos are found
if (data.items.length === 0) {
alert("Sorry, no images match your search term '" + searchTerm + "'");
//reenable the search field
$searchField.prop("disabled", false);
// reenable the submit button and change it's value back
$submitButton.prop("disabled", false).val("Search");
} else { // build the html while looping through the array
var photoHTML = '<ul>';
$.each(data.items, function(i, photo) {
photoHTML += '<li class="grid-25 tablet-grid-50">';
photoHTML += '<a href="' + photo.link + '" class="image">';
photoHTML += '<img src="' + photo.media.m + '"></a></li>';
}); // end each
photoHTML += '</ul>';
// add photoHTML to the webpage
$('#photos').html(photoHTML);
//reenable the search field
$searchField.prop("disabled", false);
// reenable the submit button and change it's value back
$submitButton.prop("disabled", false).val("Search");
} // end if/else
} // end displayPhotos()
$.getJSON(flickerAPI, flickrOptions, displayPhotos);
}); // end submit
}); // end ready
1 Answer
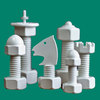
Steven Parker
231,269 PointsWhere was this "optional challenge" stated? Since the service sends back a list of specific images based on the search term, i'm not sure how you can get different ones.
You could, of course, scramble the order in which they are displayed, but $each wouldn't help with that. And that seems like a lot of trouble since they'd still be the same images.