Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial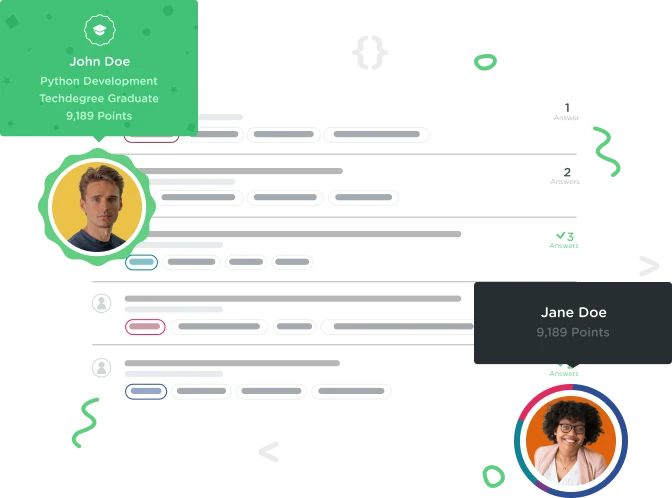

Richard Humphrey
Courses Plus Student 750 PointsStage 5 Challenge 1 How am I supposed to get numbers into a list without using input()? Just declare list_a=[1,2,3]?
I think I'm making this way harder than it should be.
3 Answers

Tatiana Jamison
6,188 PointsYep, just define the list in the code! It's not the most algorithmic, but it's a good way to do proof-of-concept by starting simple and building up.

Tatiana Jamison
6,188 PointsYou can still define the function so that it takes a variable, just skip over the part where you actually ask for user input. You can define your own list in the code so that you can see how it operates. When I'm stuck on a challenge, I like to play around in Workspaces -- you can build the program up from smaller steps, and it gives more useful feedback. Hope that helps!

Richard Humphrey
Courses Plus Student 750 PointsThanks for the reply.
After talking with a friend more knowledgeable than me, I've got the following code:
def add_list(myList):
sum = 0
for x in range(len(myList)):
sum +=myList[x]
return sum
myList = [1,2,3]
print(str(add_list(myList)))
It runs correctly and returns 6 when I run it in a Workspace, but it gets a TabError on line 3 when I try to run in the Code Challenge (I've tried moving indentations around all over.) Also, it uses techniques that haven't been introduced yet in the course... which makes me think I'm making this more complicated than it's supposed to be.
***EDIT - aaannnnnndd I tried again, and it works. :) Moving on...

Tatiana Jamison
6,188 PointsGlad you were able to get it to work! You can simplify that for loop a bit, using the same type of loop as in the shopping list project--
def add_list(my_list):
sum = 0
for item in my_list:
sum += item
return sum
Richard Humphrey
Courses Plus Student 750 PointsRichard Humphrey
Courses Plus Student 750 PointsThanks for the reply!
Here's the challenge:
"Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input()."
add_list([1, 2, 3]) should return 6
summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
Note: both functions will only take one argument each.
I'm completely lost as to how to approach this. I've reviewed the videos and can't seem to find anything that helps me here. I feel like I've missed a section or something. If I declare myList = (1,2,3), wouldn't that make it impossible to run (for example) add_list([2,4,6])?