Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial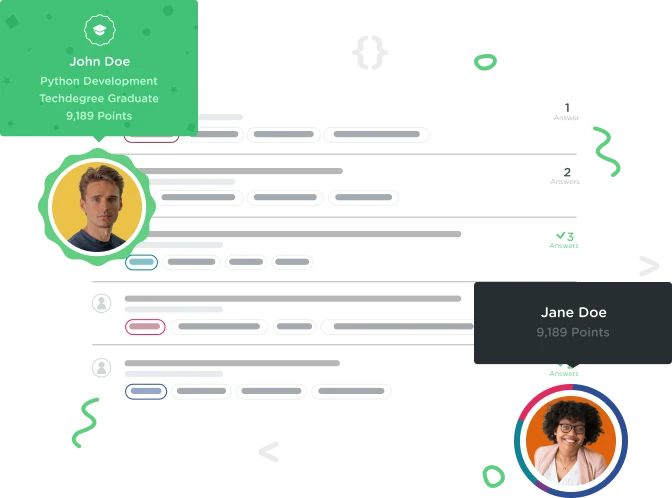
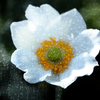
ellie adam
26,377 Pointsstage 5 creating reuseable function
function max(8, 4){ if (8 > 4){ return true ('8 is larger than 4');
}else{ return false }
}; max();
function max(8, 4){
if (8 > 4){
return true ('8 is larger than 4');
}else{
return false
}
};
max();
3 Answers
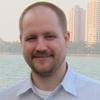
Ryan Field
Courses Plus Student 21,242 PointsYou need to replace your 8 and 4 with parameters so that the function can take ANY two numbers, not just the ones hard coded. (Additionally, you can't actually put 8 and 4 inside the parentheses because parameter and variable names are not allowed to start with numbers).
You'd have to change your code to something like this:
function max(num1, num2){
var result = '';
if (num1 > num2){
result = num1 + ' is greater than ' + num2;
}else{
result = num1 + ' is less than ' + num2;
}
return result;
};
//Here, you call your function with your two numbers.
max(100,50);
You could also change return result;
into alert(result);
to have it pop up in an alert dialog, but most of the time you'll want to return it so that it can be used in your code for other things. In the case that the input is coming from a text input or prompt dialog, you'll also need to call the parseInt()
method on num1
and num2
before comparing them.
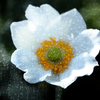
ellie adam
26,377 PointsI tried both ways but still have error.
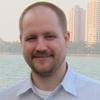
Ryan Field
Courses Plus Student 21,242 PointsI see what the issue is now. The challenge wants you to return the larger number. In that case, you want something like this:
function max(num1, num2){
if (num1 > num2){
return num1;
}else{
return num2;
}
};
For the challenges (especially with the JavaScript ones), pay close attention to what it wants the code to do. In this case, it is actually evaluating your code and will only let you pass if it returns a number.
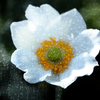
ellie adam
26,377 PointsHi there, Thanks! last code let me clear part one. In part two I am trying Alert box but not sure it goes inside the function or not.
function max(num1, num2){ if (num1 > num2){ return num1; }else{ return num2;
}
}; alert(Math.random(2,10));
Now I am stuck here