Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial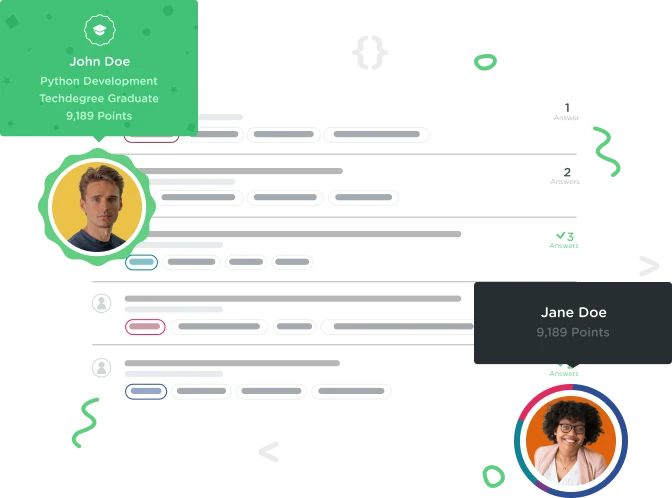
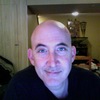
Charles Harpke
33,986 Pointsstarter is a naive datetime. Use pytz to make it a "US/Pacific" datetime instead and assign this converted datetime...
It is stating that 'local' isn't the right timezone.... Here is my code:
import datetime
import pytz
pacific = pytz.timezone('US/Pacific')
fmt = '%m-%d %H:%M %Z%z'
utc = pytz.utc
start = pacific.localize(datetime.datetime(2015, 10, 21, 4, 29))
start_pacific = start.astimezone(pacific)
starter = datetime.datetime(2015, 10, 21, 4, 29)
starter_utc = datetime.datetime(2015, 10, 21, 4, 29, tzinfo=utc)
local = starter_utc.astimezone(pacific)
import datetime
import pytz
pacific = pytz.timezone('US/Pacific')
fmt = '%m-%d %H:%M %Z%z'
utc = pytz.utc
start = pacific.localize(datetime.datetime(2015, 10, 21, 4, 29))
start_pacific = start.astimezone(pacific)
starter = datetime.datetime(2015, 10, 21, 4, 29)
starter_utc = datetime.datetime(2015, 10, 21, 4, 29, tzinfo=utc)
local = starter_utc.astimezone(pacific)
10 Answers
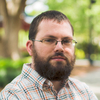
Kenneth Love
Treehouse Guest TeacherSince starter
is naive and, thus, has no timezone, you don't have to turn it into UTC first (that's for converting between timezones). You should just need to localize starter
as US/Pacific.

Andrew Winkler
37,739 PointsThis worked for me:
import datetime
import pytz
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
pacific = pytz.timezone('US/Pacific')
local = pacific.localize(starter)
pytz_string = local.strftime(fmt)

MUZ140348 Sympathy Makururu
10,209 PointsAfter a long struggle with this one: Thanks it passed.
import datetime import pytz
fmt = '%m-%d %H:%M %Z%z' starter = datetime.datetime(2015, 10, 21, 4, 29)
pacific = pytz.timezone('US/Pacific') local = pacific.localize(starter) pytz_string = local.strftime(fmt)

james white
78,399 PointsKenneth says:
"You should just need to localize starter as US/Pacific"
--but how? (I guess its easier said than done..)
Some tries:
Attempt #1:
import datetime
import pytz
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
pacific = pytz.timezone('US/Pacific')
fmt = '%m-%d %H:%M %Z%z'
local = starter.astimezone(pacific)
Gives this error:
Bummer! ValueError: astimezone() cannot be applied to a naive datetime
Attempt #2:
import datetime
import pytz
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
start = pacific.localize(datetime.datetime(2015, 10, 21, 4, 29))
pacific = pytz.timezone('US/Pacific')
fmt = '%m-%d %H:%M %Z%z'
local = starter.localize(pacific)
Gives this error:
Bummer! AttributeError: 'datetime.datetime' object has no attribute 'localize'
(are we having fun yet?)
Dozens of attempts later:
import datetime
import pytz
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
pacific = pytz.timezone('US/Pacific')
local = pacific.localize(datetime.datetime(2015, 10, 21, 4, 29))
Which moved (nudged) me on to part two of the challenge :
"Now create a variable named pytz_string by using strftime with the local datetime. Use the fmt string for the formatting."
What? Huh? Really?
Just for quick reference, I'll post below at the code Charles Harpke provided in the only other pytz_string related forum post (I could find):
import datetime
import pytz
starter = pytz.utc.localize(datetime.datetime(2015, 10, 21, 23, 29))
to_timezone = pytz.timezone('US/Pacific')
fmt = '%Y-%m-%d %H:%M:%S %Z%z'
utc = pytz.utc
starter.strftime(fmt)
Of course, it's not correct (kudos to Charles for the code, though..)
...but it started me down a very long and twisty path full of trial and error attempts that ended (after much time passing ---but I wanted those 317 points gawddernit!) with a single line:
pytz_string = local.strftime(fmt)
I know you're thinking:
That's all that really needs to be added (to the code I posted above that passed for part 1 of the challenge) in order to pass part 2 of the challenge? 'fraid so.. *sigh*
I think I'll go back to some Android courses for a while..

Annie Scott
27,613 PointsThank you been working on this for over 4 hours not getting any where, videos, just no help what so ever
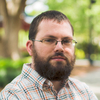
Kenneth Love
Treehouse Guest TeacherAt 2 minutes into the video, we go over using .localize()
. In fact, we even make it into a Pacific timezone datetime
using .localize()
. I'd appreciate it if you'd stop saying things aren't in the videos when they actually are.

Michael Q
4,506 Pointslord after numerous attemps, i got partit im not sure how to do the markdown, havent looked to much at it. But after every = make it be a new line
import datetime import pytz fmt = '%m-%d %H:%M %Z%z' starter = datetime.datetime(2015, 10, 21, 4, 29) pacific = pytz.timezone('US/Pacific') local = pacific.localize(starter) pytz_string = local.strftime(fmt)

Taig Mac Carthy
8,139 PointsNot all heroes wear capes

Peter Rzepka
4,118 Pointsimport datetime
import pytz
pacific = pytz.timezone('US/Pacific')
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
local = pacific.localize(starter)
#pt 2
pytz_string = local.strftime(fmt)
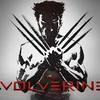
Wolverine .py
2,513 Pointslocal = pacific.localize(starter)
pytz_string = local.strftime(fmt)
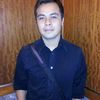
Jose Luis Lopez
19,179 Pointsimport datetime import pytz
fmt = '%m-%d %H:%M %Z%z' starter = datetime.datetime(2015, 10, 21, 4, 29) pacific = pytz.timezone('US/Pacific')
local = pacific.localize(starter) pytz_string = some code here.strftime(some other code here) this is what second question asked for not need to write anymore code

Megan L
2,905 Pointsimport datetime
# added pytz
import pytz
fmt = '%m-%d %H:%M %Z%z'
starter = datetime.datetime(2015, 10, 21, 4, 29)
# added pacific timezone
pacific = pytz.timezone('US/Pacific')
# used variable local to localize pacific time
local = pacific.localize(datetime.datetime(2015, 10, 21, 4, 29 ))
#part2
# create pytz string variable
pytz_string = local.strftime(fmt)
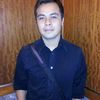
Jose Luis Lopez
19,179 Pointsimport datetime import pytz Don't forget to import pytz
fmt = '%m-%d %H:%M %Z%z' starter = datetime.datetime(2015, 10, 21, 4, 29) pacific = pytz.timezone('US/Pacific') you have to assign a timezone like this one
local = ****.localize(********) assing the local variable and convert starter to the timezone
I hope this help for all of those we were struggling with this question
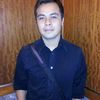
Jose Luis Lopez
19,179 PointsSorry, I forgot to mention that do not for get the indentations this code lol