Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial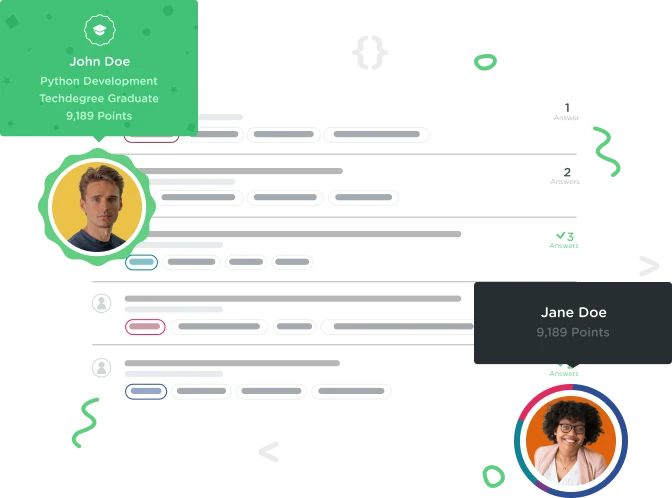
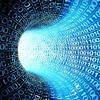
David Dong
5,593 PointsStatements Inside Functions Repeating
function alertDotRandom() {
var correctAnswer = 0;
var randomNumber = Math.floor( Math.random() * 6 ) + 1;
var numberGuess = prompt("Guess a number from 1 to 6!");
if (numberGuess == randomNumber) {
alert("Yay! You guessed the correct number!")
correctAnswer + 1;
if (correctAnswer === 4) {
document.write("Congradulations! You got all 4 questions correct, so you got a golden grown!");
} else if (correctAnswer === 3) {
document.write("Nice job! You got 3/4 questions correct, so you got a silver crown!");
} else (correctAnswer <= 2)
document.write("Keep trying! You got 2 (or less) questions correct, so you get a bronze crown!");
} else {
alert("Sorry, the correct answer was " + randomNumber);
}
}
alertDotRandom();
alertDotRandom();
alertDotRandom();
alertDotRandom();
alert("Reload this page to play again.");
I was adding on to one of the programs that the instructor had given, and I came across a weird problem. When running the above code, this happens:
1) When getting 0 guessed numbers correct, the document.write("Keep trying! You got 2 (or less) questions correct, so you get a bronze crown!");
would not run.
2) When getting 1 correct, the document.write("Keep trying! You got 2 (or less) questions correct, so you get a bronze crown!");
would run once.
3) When getting 2 correct, the document.write("Keep trying! You got 2 (or less) questions correct, so you get a bronze crown!");
would run twice.
4) When getting 3 correct, the document.write("Nice job! You got 3/4 questions correct, so you get a silver crown!");
would run three times.
5) When getting 4 correct, the document.write("Congradulations! You got all 4 questions correct, so you get a gold crown!");
would run four times.
Disclaimer: The last two may not be correct, as I have not gotten 3 or more correct yet, but out of pure logic, I think my list is correct.
Why is this?
5 Answers
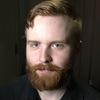
Nicholas Vogel
12,318 PointsIt looks like you've called your function 4 times at the bottom. Was that intentional? That could contribute to your issue.
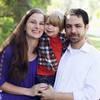
Christopher Lebbano
15,338 PointsMake sure that you are parsing your prompts at ints using parseInt();
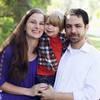
Christopher Lebbano
15,338 PointsBecause the prompt takes in a string, and a string would not be equal to a number
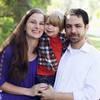
Christopher Lebbano
15,338 PointsSorry, I didn't actually reaed your code before, you can't use an if conditional statement like a for loop. Your code only interates once, so correct answer could only ever be one

Michael Lambert
6,286 PointsEach time the alertDotRandom() is called the variable correctAnswer is set back to 0 so the maximum number it ever gets to is 1. You need to move the variable declaration outside of the function so it only ever resets the correctAnswer to 0 when the page is reloaded which is what it seems was your intention.
You are also writing out the result each time a correct guess is achieved. If you correctly guessed each time then at the the end you would see 4 messages, one for each score condition. Would be better to store the message in a shared variable so that you can print out it's final content (which would only be one result) by placing the document.write just before the alert at the bottom of the script.

nico dev
20,364 PointsHey, I know it's a little late, but I wanted to add another detail that I think is also important.
When you wrote:
correctAnswer + 1; // Here you're actually just adding 1 to the value of the variable, but you're not assigning the new value to the variable. That is: correctAnswer is still the previous value.
Here:
correctAnswer += 1; // This is how you add 1 and assign the new value to the variable.