Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial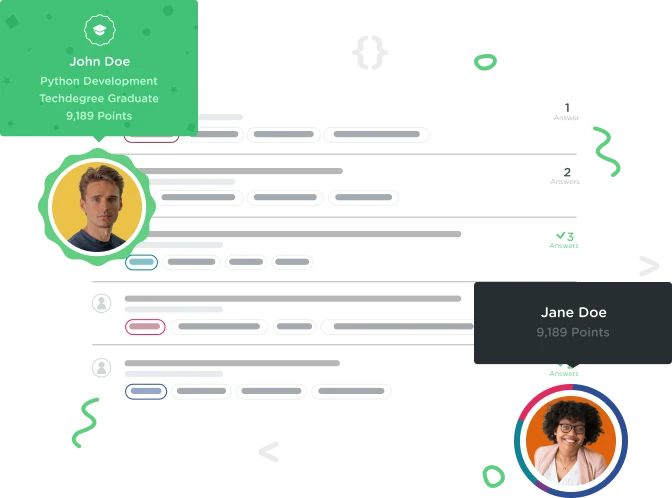

fadhil suhendi
7,680 Pointsstatic method and instance method
What is the difference between static method and instance method? and for example when I create a class, can I just call the method by static method ? instead of creating a new object.. thank you
1 Answer
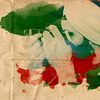
Gunjeet Hattar
14,483 PointsWhat is the difference between static method and instance method?
Instance method or simply method is a class member which needs its reference, so as to be used someplace else
Static methods are methods that do need reference to be used.
A very common example is the method main which you have been using all this while. So before anythings loads it is important that the main is called first. Since it is static and does not rely on a reference, it can do so.
and for example when I create a class, can I just call the method by static method
Whenever you need to use an instance method outside of its scope you need to refer it through the classes' instance.
class A {
public void hello() {
// some code
}
}
public class MainClass {
A a = new A(); // here a is an instance of class A
a.hello(); // to access hello method inside A we need to refer it via its reference variable
}
Now when I add static to a method what it means is that you do not need to create a reference variable to refer it in some other class.
class A {
static void hello() {
// some code
}
}
public class MainClass {
hello(); //no need to create a reference variable of the class
}
However, there are some rules when applying static to a method
- they can only call other static methods
- they cannot use this and super keywords
- and can only access static data
Hope this helps! Ask again if you require.
fadhil suhendi
7,680 Pointsfadhil suhendi
7,680 PointsHi, thanks for answering my question! I think I understand it now the different between both method. So when do we want to use static method ? is there any particular situation where static method is useful ?
Gunjeet Hattar
14,483 PointsGunjeet Hattar
14,483 PointsThere could be a number of possible situations. For instance say you want to initialise something even when no object for it is ever defined. If you think it makes sense to do that, then go ahead and make it static. There aren't any hard and fast rules as to when to make a method static.