Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial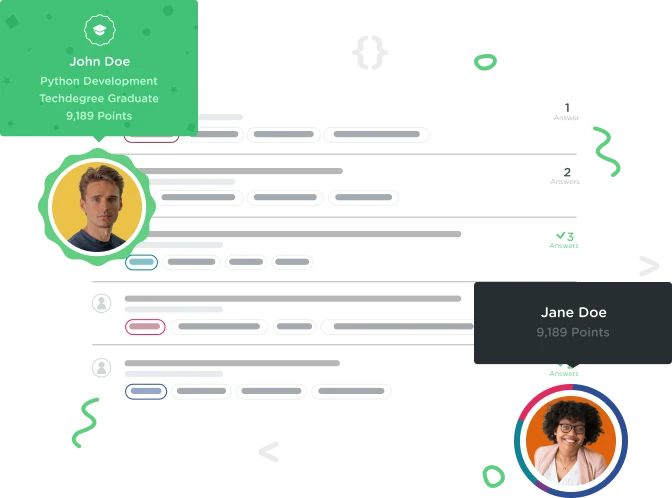

Lance McCormick
7,553 PointsStats Challenge (Step 3) - How can I improve my code?
This step really challenged me, but I powered through it with many tests in the python interpreter and my own code editor. My code passed the challenge but I feel like there might be an easier, more pythonic way of thinking about these things.
At first I ran into string conversion issues when trying to append stuff directly into my teachers list. But I eventually moved away from that and decided on using a sub-list and appending that to the main teachers list instead. This one really made me think hard about how I could create a list of lists.
How can I improve my code?
def stats(teacher_dict):
teacher_list = []
for teacher in teacher_dict:
sub_list = ['', 0]
name = teacher
num_classes = len(teacher_dict[teacher])
sub_list[0] = name
sub_list[1] = num_classes
teacher_list.append(sub_list)
return teacher_list
2 Answers
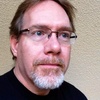
Chris Freeman
Treehouse Moderator 68,457 PointsYou have all the right elements. The only improvements would be to streamline the statements.
# The for loop block
sub_list = ['', 0]
name = teacher
num_classes = len(teacher_dict[teacher])
sub_list[0] = name
sub_list[1] = num_classes
teacher_list.append(sub_list)
# Can be replaced by
teacher_list.append([teacher, len(teacher_dict[teacher])])
Using the dict.items()
method to return both the key and the value, this can be compress further:
def stats(dct):
result = []
for teacher, course_list in dct.items():
result.append([teacher, len(course_list)])
return result
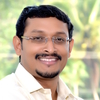
Aby Abraham
Courses Plus Student 12,531 Pointsdef stats(dict):
slist = []
for key, value in dict.items():
slist.append([key, len(value)])
return slist