Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial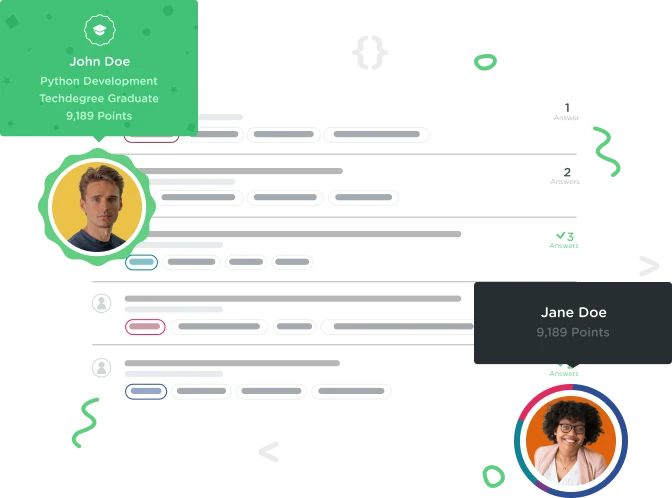

Nikolaos Christou
508 PointsStats.js is getting an error Cannot read property 'length' of undefined
Stats.js file below:
import React from 'react'
const Stats = ({players}) => {
console.log("Players: " + players);
const totalPlayers = players.length; const totalPoints = players.reduce( (total, player) => { return total + player.score; }, 0);
return ( <table className="stats"> <tbody> <tr> <td>Players:</td> <td>{ totalPlayers }</td> </tr> <tr> <td>Total Points:</td> <td>{ totalPoints }</td> </tr> </tbody> </table> ); }
export default Stats;
Player.js below:
import React, { PureComponent} from "react"; import Counter from "./Counter_"
class Player extends PureComponent { render() {
const {
name,
id,
score,
index,
removePlayer,
changeScore
} = this.props;
console.log(name + ' rendered');
return(
<div className="player">
<span className="player-name">
<button className="remove-player" onClick = { ()=> removePlayer(id)}>✖</button>
{ name }
</span>
<Counter
score={score}
index ={index}
changeScore={changeScore}
/>
</div>
);
}
}
export default Player;
Error:
Players: undefined Stats.js:8 Uncaught TypeError: Cannot read property 'length' of undefined at Stats (Stats.js:8)
1 Answer
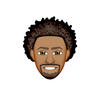
Kiel Lewis
Full Stack JavaScript Techdegree Graduate 18,732 PointsHey it seems that you are returning undefined
because you are attempting to check the length of an obj
({players}
) as opposed to an array
or string
which have that method.
So i guess the first question is, should the parameter players
be an array or an obj? My guess is an array but want to be sure.
Also this line const totalPlayers = players.length; const totalPoints = players.reduce( (total, player) => { return total + player.score; }, 0);
may fail after updating that parameter (at least for me it did). It seems you will be trying to update multiple states which may be a good use case for you to look into reacts useReducer()
hook which operates very much the same as arr.reduce()
I hope some of this can help get you back on track!